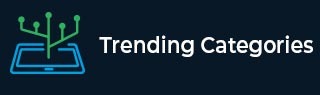
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

76 Views
In Python, strings are a fundamental data type used to represent sequences of characters. They are enclosed in single quotes ('') or double quotes ("") and offer a wide range of operations and methods for manipulation Example Assume we have taken an input string. We will now find the maximum scoring word from an input string using the above methods. Input inputString = "hello tutorialspoint python users and learners" Output Resultant maximum scoring word from the input string: tutorialspoint In the above input string, the sum of characters' positional values of the word tutorialspoint is having the ... Read More

225 Views
In Python, a dictionary is a built-in data structure that stores data in key-value pairs. It allows efficient retrieval and modification of values based on their corresponding keys. Each key in a dictionary must be unique, and it is associated with a specific value. A dictionary of lists is a dictionary where the values associated with each key are lists. This means that each key maps to multiple values, and those values are stored as elements within a list. On the other hand, a list of dictionaries is a list that contains multiple dictionaries as its elements. Each dictionary within ... Read More

71 Views
The meaning of value key assignment in Python is the process of associating a value with a specific key within a dictionary, allowing efficient retrieval and manipulation of data based on key-value pairs. It enables the creation and organization of structured data structures for various purposes Python's implementation of a data structure known more commonly as an associative array is a dictionary. A dictionary is made up of a group of key-value pairs. Each key-value combination corresponds to a key and its corresponding value. Example Assume we have taken two input dictionaries with the same keys. We will now compare ... Read More

1K+ Views
A byte string is similar to a string (Unicode), except instead of characters, it contains a series of bytes. Applications that handle pure ASCII text rather than Unicode text can use byte strings. Converting a byte string to a list in Python provides compatibility, modifiability, and easier access to individual bytes. It allows seamless integration with other data structures, facilitates modification of elements, and enables efficient analysis and manipulation of specific parts of the byte string. Demostration Assume we have taken an input byte string. We will now convert the given byte string to the list of ASCII values ... Read More

68 Views
An array(list) of comma-separated values (items) enclosed in square brackets is one of the most flexible data types available in Python. The fact that a list's elements do not have to be of the same type is important. In this article, we will learn a python program to check if any key has all the given list elements. Example Assume we have taken an input dictionary and a list. We will now check whether the values of any key of the input dictionary contain given list elements using the above methods. Input inputDict = {'hello': [4, 2, 8, 1], 'tutorialspoint': ... Read More

111 Views
Strings in Python are sequences of characters used to represent textual data, enclosed in quotes. Checking for almost similar strings involves comparing and measuring their similarity or dissimilarity, enabling tasks like spell checking and approximate string matching using techniques such as Levenshtein distance or fuzzy matching algorithms. In this article, we will learn a Python Program to check for almost similar Strings. Demonstration Assume we have taken an input string Input Input string 1: aazmdaa Input string 2: aqqaccd k: 2 Output Checking whether both strings are similar: True In this example, ‘a’ occurs ... Read More

61 Views
In Python, an element index refers to the position of an element within a sequence, such as a list or string. It represents the location of an element, starting from 0 for the first element and incrementing by 1 for each subsequent element. Assigning keys with the maximum element index means associating unique identifiers or labels with the highest possible index value in a sequence. This approach allows for easy access and retrieval of elements based on their positions using the assigned keys. Example Assume we have taken an input dictionary. We will now find the maximum element in the ... Read More

381 Views
In Python, a set is an unordered collection of unique elements, represented by {}. It allows efficient membership testing and eliminates duplicate values, making it useful for tasks such as removing duplicates or checking for common elements between sets. Appending multiple elements to a set in Python is a common operation that involves adding multiple unique elements to an existing set. In this article, we will learn how to append multiple elements in a set in python. Example Assume we have taken an input set and input list. We will now append the input list elements to the input set ... Read More

41 Views
In Python, a set is an unordered collection of unique elements, represented by {}. It allows efficient membership testing and eliminates duplicate values, making it useful for tasks such as removing duplicates or checking for common elements between sets. In this article, we will learn how to access the K element in a set without deletion in python. Example Assume we have taken an input set and K element. We will now find the index of that K element in an input set using the above methods. Input inputSet = {3, 9, 5, 1, 2, 8} k=5 Output The ... Read More

154 Views
String consonants refer to the non-vowel sounds produced when pronouncing a string of characters or letters. In this article, we are going to study how to reverse only the consonants in a string without affecting the position of other elements using the following 2 methods in Python: Using append() and join() functions Using pop() function and string concatenation Example Before Reversing : tutorialspoint After Reversing: tunopiaslroitt Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task –. The function checkConsonant(char) is defined to check if a character is a consonant ... Read More