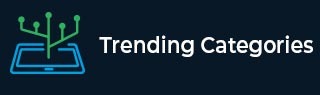
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming
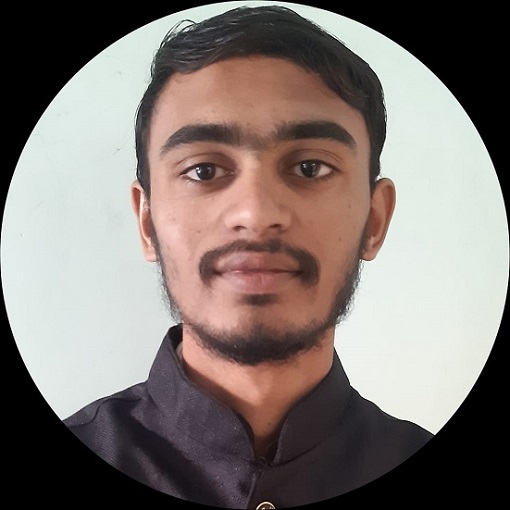
146 Views
In this problem, we need to count the total number of substrings of string str containing exactly K distinct vowels. We can solve the problem in two different ways. The first approach is to traverse all substrings and count the number of vowels in each substring. We can also use the map data structure to optimize the code of the first approach. Problem statement – We have given string str of length N. The string contains uppercase and lowercase alphabetical characters. Also, we have given integer K. We need to find the total number of substrings of str containing exactly ... Read More
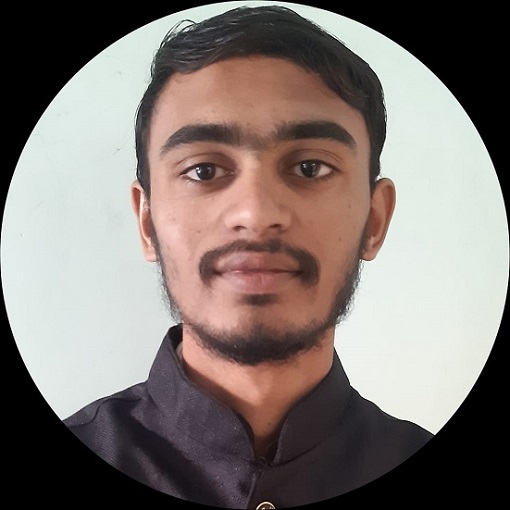
43 Views
In this problem, we need to find a total number of substrings of length K containing exactly K vowels. We will see two different approaches to solving the problem. We can use a naïve approach that checks the number of vowels in each substring of length K. Also, we can use the sliding window approach to solve the problem. Problem statement – We have given a string str of length N containing lowercase and uppercase alphabetical characters. We need to count the total number of substrings of length K which contains exactly X vowels. Sample examples Input– str = ... Read More
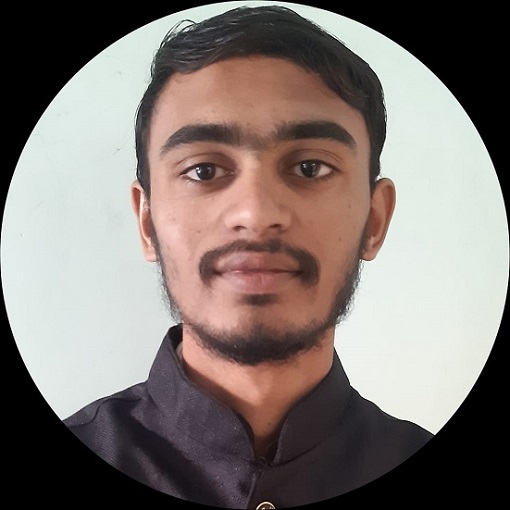
186 Views
In this problem, we need th check whether the substring S1 appears after any occurrence of the substring S2 in the given string S. We can compare the starting index of S1 and S2 in the string S to solve the problem. Problem statement – We have given three substrings named S, S1, and S2. The string S always contains S1 as a substring. We need to check whether the substring S1 appears after any occurrence of the substring S2 in the given string S. Sample examples Input – S = "abxtutorialspointwelcomepoint", S1 = "welcome", S2 = "point"; ... Read More
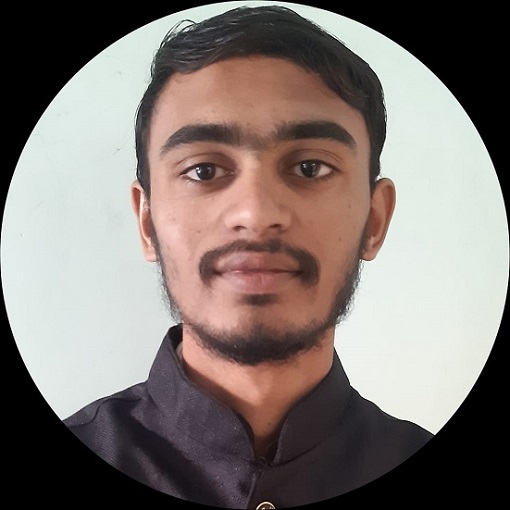
70 Views
We have given two strings and need to check whether the given string's permutations exist such that one permutation can have a larger character than another permutation at the ith index. We can solve the problem by sorting the string and comparing each character of the string one by one. Also, we can solve the problem using the frequency of characters of both strings. Problem statement – We have given a string str1 and str2 of length N. We need to check whether any permutations of both strings exist such that the permutation of one string is lexicographically larger than ... Read More
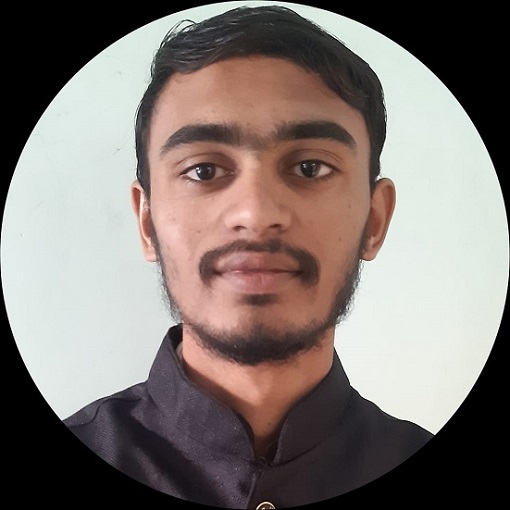
58 Views
In this problem, we need to find the maximum length of the sub sequence with the same left and right rotations. The left rotation means moving all characters of the string in the left direction and the first character at the end. The right rotation means moving all string characters in the right direction and the last character at the start. Problem statement – We have given string str containing numeric digits and need to find the sub sequence of maximum length with the same left and right rotations. Sample examples Input – str = "323232", Output – 6 Explanation– ... Read More
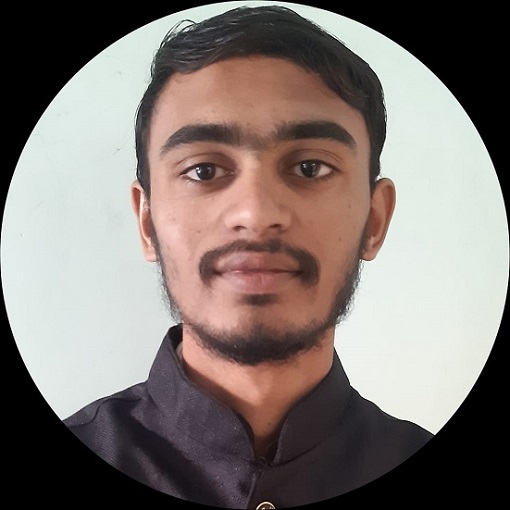
54 Views
In this problem, we need to find the average value of the count of set bits after performing K operations of all choices on the given string. There can be a brute force approach to solve the problem, but we will use the probability principles to overcome the time complexity of the brute force approach. Problem statement – We have given an integer N, array arr[] containing K positive integers, and a binary string of length N containing only set bits. We need to find the average value of the count of set bits after performing all possible choices ... Read More
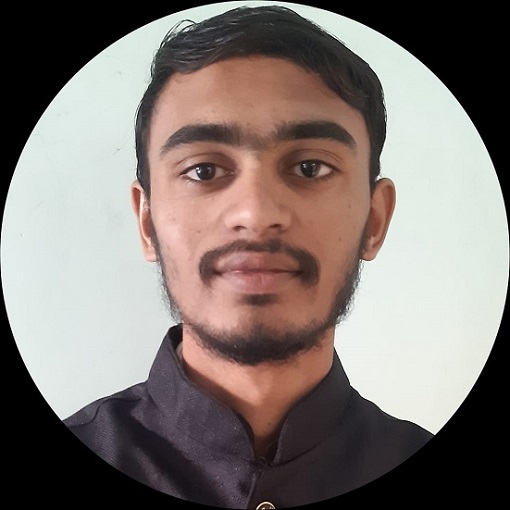
76 Views
In this problem, we need to find the total number of sub strings of length K containing at most X distinct vowels. We can solve the problem in two different ways. The first way is to get all sub strings and count distinct vowels in each sub string of length K. The second way is to use a map data structure and track the number of distinct vowels in the particular sub string. Problem statement– We have given string str of length N. The string contains only alphabetical characters. Also, we have given K and X positive integers. We need ... Read More

256 Views
A numeric string in Python is a string that represents a numerical value using digits, signs, and decimal points, allowing for mathematical operations and data processing. In this article, we will learn a python program to increment numeric strings by K. Example Assume we have taken an input list of strings. We will now increment the numeric strings in an input list by k using the above methods. Input inputList = ["10", "hello", "8", "tutorialspoint", "12", "20"] k = 5 Output ['15', 'hello', '13', 'tutorialspoint', '17', '25'] In the above list, 10, 8, 12 20 are the numeric ... Read More

116 Views
Finding the key of the maximum value tuples in a python dictionary means identifying the key associated with the tuple that has the highest value among all the tuples in the dictionary, which can be useful for retrieving the most significant or relevant information from the data. Example Assume we have taken an input dictionary having values as tuples. We will now find the key of maximum value tuples in an input dictionary using the above methods. Input inputDict = {'hello': ("p", 2), 'tutorialspoint': ("c", 15), 'python': ("k", 8)} Output The key of the maximum value in a ... Read More

321 Views
In Python, a set is an unordered collection of unique elements, represented by {}. It allows efficient membership testing and eliminates duplicate values, making it useful for tasks such as removing duplicates or checking for common elements between sets. In this article, we will learn a python program to find the most common elements set. Methods Used The following are the various methods to accomplish this task: Using for loop and set.intersection() function Using list comprehension, max() and intersection() functions Demonstration Assume we have taken an input list containing sets. We will now get the most ... Read More