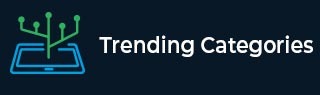
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming
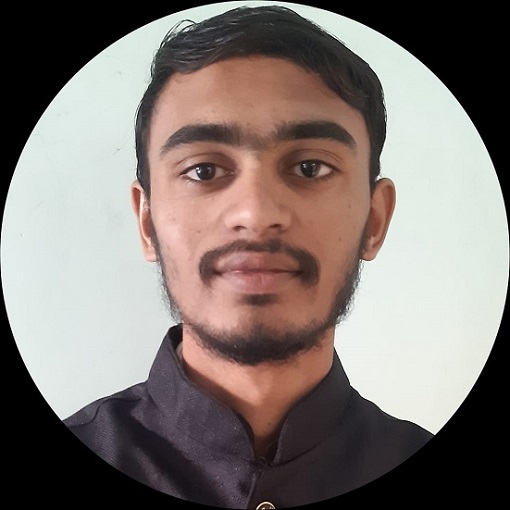
118 Views
In this problem, we need to find the maximum length of the subsequence containing the single character by increasing the multiple characters by, at most, k times. We can use the sliding window approach to solve the problem. We can find the maximum length of any window to get the result after sorting the string. Problem statement – We have given string str containing lowercase alphabetical characters. Also, we have given the positive integer k. After performing the at most k increment operations with multiple characters of the given string, we need to find the maximum length of the ... Read More
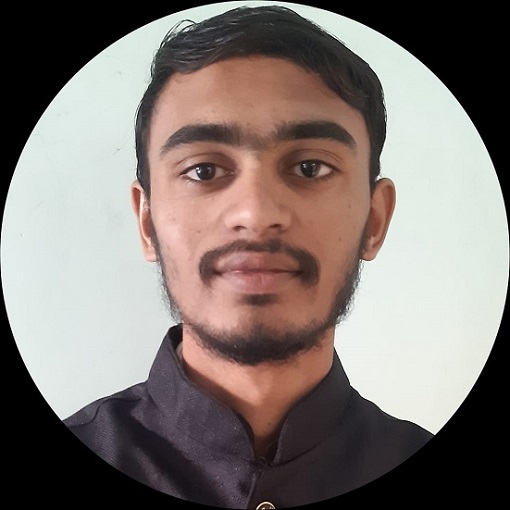
255 Views
In this problem, we need to generate a lexicographically smallest string using the first K characters of alphabets so that it doesn’t contain any repeated substring. We can generate a string so that all substrings of length 2 are unique. So, if all substrings of length 2 are unique, all substrings of length 3 or more are also unique. Problem statement – We have given a positive integer K. We need to generate a new string using the first K alphabets so that generated string can’t contain any repeated substring of length 2 or more and is lexicographically smallest. Sample ... Read More
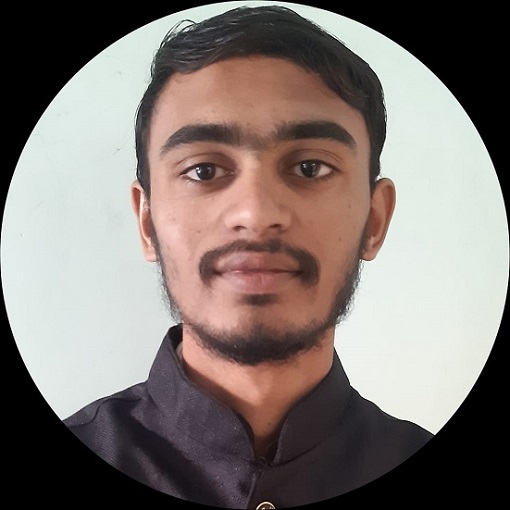
41 Views
In this problem, two players play a game by removing the string from their array, which is not already removed from the opponent's array. We need to decide the winner of the game. We can solve the problem using two different approaches. In the first approach, we can store the common strings with the set data structure. In the second approach, we can use a set to store already removed strings from both arrays. Problem statement – We have given two arrays called arr and brr. The size of the array is N and M, respectively. We need to decide ... Read More
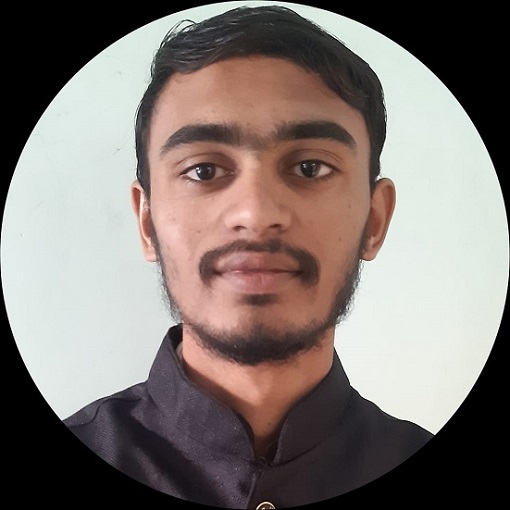
33 Views
In this problem, we have given a string suggesting the moving direction and starting coordinates. We need to find the revisited positions. We can use the set or map data structure to store the previously visited coordinates. We can say that position is revisited if we find any pair in the set or map. Problem statement – We have given a string str of length N containing the ‘L’, ‘R’, ‘U’, and ‘D’ characters. Also, we have given X and Y integers representing the starting position. We need to find the total number of coordinates revisited while following the path ... Read More
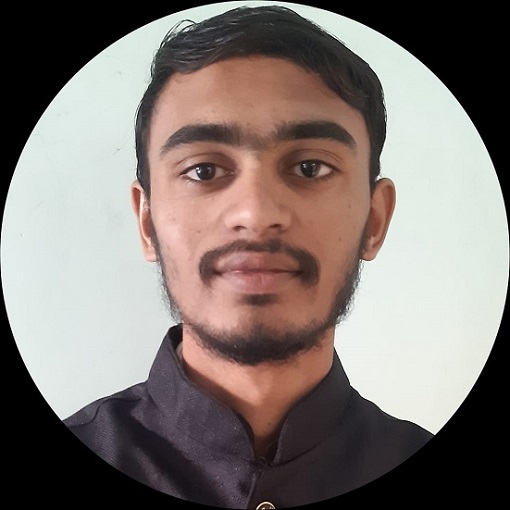
106 Views
In this problem, we need to count the total occurrences of the substring X in the str when we find the substring Y in the given string. We can keep counting the occurrences of the substring X, and when we get the substring Y, we can print the count value. Problem statement – We have given a string str, X, and Y. The length of the strings is N, A, and B, respectively. We need to total the total number of substring X in the given string str before every occurrence of the substring Y. Sample examples Inputstr = "stuxystuxy"; ... Read More
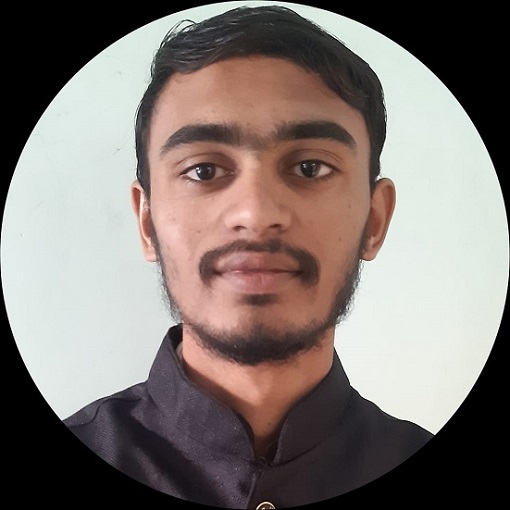
106 Views
C++ contains many precious features to enhance the performance of the code, and string_view class is one of them. It is introduced to create a lightweight and non-owning reference to a string. In this tutorial, we will discuss the string_view class and explore a few examples using the string_view class over the string class in C++. What is string_view? The string_view is a class in C++ that is used to create a read-only sequence of the string. It is a non-owning string type, meaning it does not manage the memory associated with the string and its reference. It acts as ... Read More
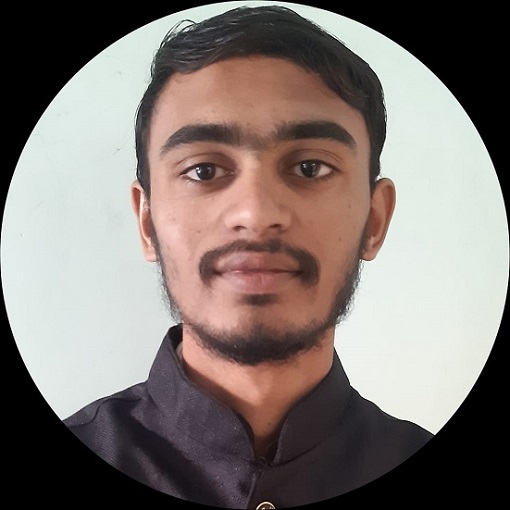
50 Views
In this problem, we need to check whether we can get the valid balanced subsequence of the parenthesis by moving at most K characters of the string at the end. To solve the problem, we can use the stack data structure. The logic to solve the problem is that if we find more than K ‘)’ (closing parenthesis) before the ‘(‘ (opening parenthesis), we can’t make a string into a valid subsequence. Problem statement – We have given string str containing the ‘(‘ and ‘)’ sequence of parenthesis. The length of the string is N. Also, We have given a ... Read More
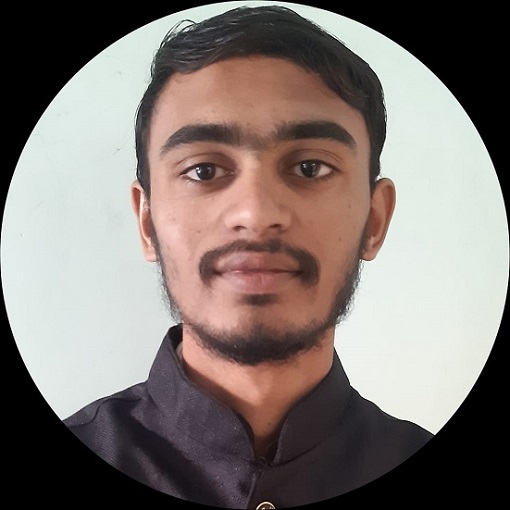
54 Views
In this problem, we have given a string, and we need to divide the string into k + 1 substrings such that the concatenation of k + 1 substrings with their reverse can give us the original string. Observation can solve the problem. If the string's first and last k characters are the same, we can say it is possible to create a k + 1 string according to the given condition. Problem statement – We have given a string of length N containing the lowercase alphabetical characters and positive integer K. We need to find whether we can ... Read More
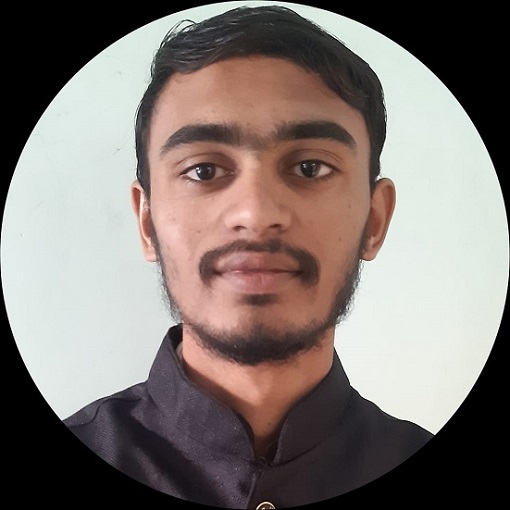
83 Views
In this problem, we need to find the last palindrome string in the array. If any string is the same in reading, either we start to read from the start or end, we can say the string is a palindrome. We can compare the starting and ending characters to check whether the particular string is palindromic. Another way to find the palindromic string is to reverse the string and compare it with the original string. Problem statement – We have given an array of length N containing the different strings. We need to find the last palindrome string in the ... Read More
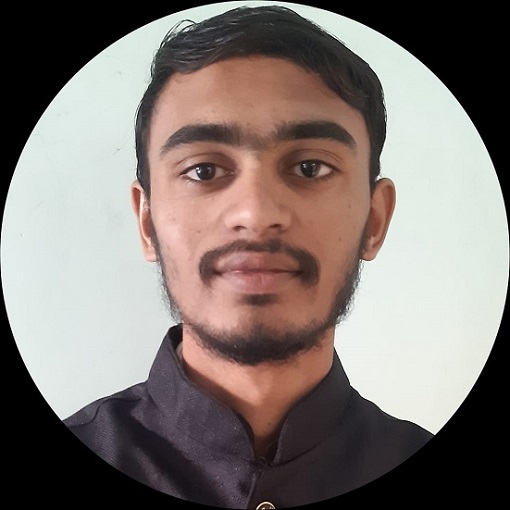
99 Views
In this problem, we need to find all missing binary strings of length N from the array. We can solve the problem by finding all permutations of a binary string of length N and checking which permutations are absent in the array. Here, we will see iterative and recursive approaches to solve the problem. Problem statement – We have given an array arr[] of different lengths containing the binary strings of length N. We need to find all the missing binary strings of length N from the array. Sample examples Input – arr = {"111", "001", "100", "110"}, N = ... Read More