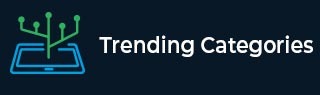
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

184 Views
In python we can use not operator or subtraction operation as well as counter function to the number that are in range but not in set. A Python set is a grouping of unsorted elements. The set's elements must all be distinct, and unchangeable, and the sets must eliminate any duplicates. Sets can be changed after they are created because they are mutable. Example Assume we have taken an input set and range Input inputSet = {3, 10, 2, 11, 15, 4, 1} lowIndex, highIndex = 0, 8 Output [0, 5, 6, 7] Here 0, ... Read More

86 Views
Python's implementation of a data structure known more commonly as an associative array is a dictionary. A dictionary is made up of a group of key-value pairs. Each key-value combination corresponds to a key and its corresponding value. In this article, we will learn how to extract keys with specific value type in python. Methods Used The following are the various methods to accomplish this task − Using for loop and isinstance() method Using list comprehension and isinstance() method Using keys() & type() methods Example Assume we have taken an input dictionary. We will now Input ... Read More

194 Views
In Python, strings are among the most often used types. These are easily made by simply surrounding letters in quotations. Python treats single quotes and double quotes the same way. Assigning a value to a variable and creating a string is really straightforward. In this article, we will learn how to expand a character frequency string in python. Methods Used The following are the various methods to accomplish this task: Using zip() and join() functions Using re(regex) module and join() function Without using any built-in functions Example Assume we have taken an input string containing characters followed ... Read More

44 Views
Python's implementation of a data structure known more commonly as an associative array is a dictionary. A dictionary is made up of a group of key-value pairs. Each key-value combination corresponds to a key and its corresponding value. In this article, we will learn how to concatenate all keys which have similar values in python. Methods Used The following are the various methods to accomplish this task: Using defaultdict() and join() functions Using dictionary comprehension, groupby() and join() functions Example Assume we have taken an input dictionary. We will now concatenate all the keys ... Read More

30 Views
In python we can use various internal functions like isupper(), islower() and and join() that can help us adding k symbol between case shifts. The following example will help you understand what is case shift and how we can add k symbol between them. Example Assume we have taken an input string and K. We will now add the input k symbol between Case shifts using the above methods. Case shift simply means the words shifting between uppercase and lowercase. Input inputString = tUtoRialsPoInt k = ‘# Output Resultant String after adding # between caseshifts − t#U#to#R#ials#P#o#I#nt ... Read More

514 Views
Python has internal functions lie count, counter and operator functions that can be used for printing all the repeated digits present in a number that too in sorted order. The following example will help you understand the concept more clearly. Example Assume we have taken an input string. We will now print all repeating/duplicate digits present in a given input number in sorted order using the above methods. Input inputNum = 5322789124199 Output 1 2 9 In the above input number, 2, 1, and 9 are repeated digits. So these digits are sorted in ascending ... Read More
119 Views
A dictionary in Python is a built-in data structure that allows you to store and retrieve values using unique keys. It is an unordered collection of key-value pairs enclosed in curly braces {}. Dictionaries provide a convenient way to organize and manipulate data, making it easy to access values based on their corresponding keys. In this article, we are going to see how to convert Dictionary to Concatenated String using Python which means that all the keys and values of the dictionary will be combined in the form of a string as follows. Input = {'one': 1, 'two': 2, ... Read More
173 Views
Data handling or organizing or structuring in Python has a lot of common tasks and 1 such task is the conversion of list to list of dictionaries. It is a simple process that allows us to associate specific keys with their corresponding values; creating a collection of dictionaries that can be simply accessed and/or manipulated. Before going forward with the techniques let us 1st see an example input and output. Consider the following: Input test_list = ['John', 25, 'Smith', 30, 'Alice', 35] key_list = ['name', 'age'] Output [{'name': 'John', 'age': 25}, {'name': 'Smith', 'age': 30}, {'name': 'Alice', 'age': ... Read More
2K+ Views
When we speak of "converting an image into string format and back again in Python", this refers to converting image files to something that can be stored and managed using strings of characters, before eventually returning it back into its original image format. Converting Image to String − Converting images to strings involves transcribing their binary data into text format for transmittal or storage over network protocols that require text-based images. This conversion method can help us transmit or store an image more efficiently when working with APIs, databases or sending images over network protocols requiring text data transmission or ... Read More
1K+ Views
Python’s Turtle library is used for generating 2D graphics and animations. It has a very simple interface with help of which we can create shapes and patterns on the screen. It comes with some built-in shapes like squares, circles, triangles etc. However, we can even create our own shapes with the help of Turtle. In this article, we are going to see how to create custom turtle shapes in python. Before going forward with the creating custom shapes we need to install Turtle on the system and this can be done by simply using the following command − pip install ... Read More