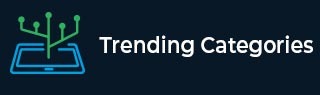
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

356 Views
Quantile and decile ranks are commonly used statistical measures to determine the position of an observation in a dataset relative to the rest of the dataset. In this technical blog, we will explore how to find the quantile and decile ranks of a Pandas DataFrame column in Python. Installation and Syntax pip install pandas The syntax for finding the quantile and decile ranks of a Pandas DataFrame column is as follows − # For finding quantile rank df['column_name'].rank(pct=True) # For finding decile rank df['column_name'].rank(pct=True, method='nearest', bins=10) Algorithm Load the data into a Pandas DataFrame. Select the ... Read More

615 Views
Finding the largest file may be helpful in a number of circumstances, including locating the biggest files on a hard drive to make room for smaller ones or examining the size distribution of files in a directory. The biggest file in a directory may be found using a Python script, which will be covered in this article. Algorithm Import the os module. Define a function called find_largest_file that takes a directory as input. Initialize a variable called largest_file to None and a variable called largest_size to 0. Use os.walk to traverse the directory tree recursively, starting from the root ... Read More

291 Views
Finding the k smallest values of a NumPy arra Installation and Syntax Pip, Python's package installer, is often used to install NumPy. pip install numpy The following function may be used to identify the k NumPy array elements with the smallest values − np.sort(array)[:k] This returns the first k items of the sorted array after sorting it in ascending order. The array may be be sorted using the alternative syntax shown below, which will retrieve the last k entries and sort the array in descending order − np.sort(array)[::-1][:k] Algorithm The algorithm for finding the k smallest ... Read More

1K+ Views
Copy documents, which consume additional room on the hard drive as we assemble information on our PC, are a commonplace event and therefore, finding and removing duplicate files manually can be time-consuming and tiresome; Thankfully, we are able to automate this procedure with Python so in this lesson we will take a look at exactly how to do that with a short script. Setup For this tutorial, we'll use the built-in os and hashlib modules in Python, so no additional packages need to be installed. import os import hashlib Algorithm Compose a function called hashfile() that utilizes the ... Read More

2K+ Views
In image processing, finding the difference between two images is a crucial step in various applications. It is essential to understand the difference between the two images, which can help us in detecting changes, identifying objects, and other related applications. In this blog, we will explore how to find the difference between two images using the Python Imaging Library (PIL). Installation and Syntax To use PIL, we need to install it using the pip package manager. We can install PIL by running the following command in the terminal − pip install pillow To find the difference between two images ... Read More

618 Views
Duplicate rows in a dataset must frequently be found and removed in data science and machine learning and to solve this issue, a well-liked Python toolkit for numerical computation called NumPy offers a number of methods for manipulating arrays. In this tutorial, we'll go through how to use Python to locate unique rows in a NumPy array. Installation and Setup NumPy must first be installed using pip before it can be used in Python. pip install numpy Once installed, we can import the NumPy library in Python using the following statement − import numpy as np Syntax The ... Read More

229 Views
NumPy is a popular Python library that provides support for numerical computations. It is widely used for array and matrix operations in scientific computing, data analysis, and machine learning. One of the most common tasks in NumPy is finding the union of two arrays. A new array that contains all the distinct elements from both arrays is created when two arrays are joined. In this article, we will explore different ways to find the union of two NumPy arrays. Installation and Syntax NumPy is usually installed with Anaconda or Miniconda distribution. If you don't have it installed, you can install ... Read More

756 Views
A common method for extracting data from web pages is known as web scraping, and the potent Python package BeautifulSoup makes it simple to do so. In this post, we'll concentrate on utilizing Python's BeautifulSoup to extract title tags from a given HTML text. Installation and Syntax Make sure BeautifulSoup is set up on your machine before you start writing any code, this can be done via the Python package installer, pip by typing out the command in your terminal. pip install beautifulsoup4 We must first build a BeautifulSoup object by supplying the HTML content or file to ... Read More

410 Views
In python, a matrix is a particular type of two-dimensional array in which all of the data elements must have exactly the same size. Hence, every matrix is also a two-dimensional array, but not the other way around.For many mathematical and scientific tasks, matrices are essential data structures. In this article, we will learn a python program to reverse every Kth row in a Matrix. Methods Used The following are the various methods to accomplish this task − Using for loop and reversed() function Using list comprehension and slicing Using index() and reverse() functions Using range() function ... Read More

286 Views
In Python, dictionary is a implementation of a data structure known more commonly as an associative array. A dictionary is made up of a group of key-value pairs. Each key-value combination corresponds to a key and its corresponding value. In this article, we will learn a python program to print the sum of all key-value pairs in a dictionary. Methods Used The following are the various methods to accomplish this task: Using For Loop and append() function(dictionary traversal method) Using dict.keys() Function Using For Loop, keys() and values() Functions Example Assume we have taken an input dictionary. ... Read More