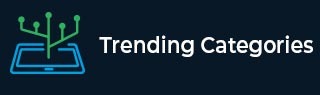
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

301 Views
Maps are reference data types in Golang, which means that assigning one map variable to another creates a reference to the same underlying data structure. To create an independent copy of a map, we need to use different methods. In this article, we will explore two methods that include the manual method and the copy() function method to create a copy of map in golanguage. Syntax newMap := make(map[keyType]valueType) In this syntax, we first declare a new map named newMap using the make() function, specifying the appropriate key and value types. originalMap := map[string]int The syntax originalMap ... Read More

2K+ Views
Maps in Go provide a convenient way to store and access data in form of key−value pairs. Deleting items from a map is a common operation when working with dynamic data. In this article, we will delete items of map in golanguage using three methods: the delete() function and iterating over the map, to remove items from a map, and creating a new map based on different conditions. Explanation Maps are versatile structures for holding key−value pairs, and managing these pairs is crucial when dealing with dynamic data. We'll use three approaches to removing items from a map, enhancing ... Read More

107 Views
Maps in Go are powerful data structures that allow us to store and retrieve key−value pairs. They are useful for various applications, such as storing and manipulating data, implementing dictionaries, and performing efficient lookups. In this article, we will write a program to create a simple map in golanguage using two methods that include the literal method and the make() function method. Algorithm Declare a map variable using the desired key and value types. Initialise the map using the literal notation by enclosing key−value pairs in curly braces {}. Assign values to the respective keys using the colon ':' ... Read More

188 Views
A slice is a portion of data extracted from an array, list or a data structure. Permutations refer to the rearrangement of elements in a specific order.Here slice permutation means to generate all the possible permutations of the number entered by user. In this article, we will explore how to perform slices permutations of numbers entered by user in Golang, using two methods the recursive approach and the iterative approach, to generate all possible permutations of the given slice. Explanation Recursive: Our first trick is the generatePermutationsRecursive() function. It starts simple, handling small batches of numbers like a warm−up. ... Read More

552 Views
A permutation is an arrangement of the characters of a string in a specific order. In some cases we need to print all the permutations of a string to create anagram games or puzzle games where users need to find out the letters hidden in the string. In this article we are going to print all permutations of a string in golanguage using two different approaches that include the recursive and the iterative approach. These methods allow us to generate all possible permutations of a given string, enabling various applications such as generating anagrams, solving permutation−based problems, and more. Explanation ... Read More

181 Views
To Print a matrix in spiral format we need to traverse the matrix in a spiral pattern, starting from the outermost layer and gradually moving inward. This approach provides a visually appealing way to display matrix elements. In this article we will use two methods to print a matrix in spiral format, the first method is by using the iterative approach and the other one is using the recursive approach. The examples below will help you understand these methods. Explanation Let us assume we have a 3 x 3 matrix, to print a matrix in spiral format we need to ... Read More

75 Views
Comb sort algorithm is a simple and efficient comparison−based sorting algorithm, Comb Sort improves upon Bubble Sort by eliminating small values towards the end of the array, resulting in faster sorting times. We can use two methods to implement Comb Sort algorithm in Gzolang, the first method is using a naive approach and the other one is using an optimized approach in which we enhance the efficiency of the algorithm by introducing a flag variable to track swaps. In this article, we will discuss the principle of comb sort algorithm and provide the syntax for the program. Explanation The Comb ... Read More

198 Views
The median is the element in the middle of a dataset when the dataset is sorted. The Median of Medians algorithm is a powerful technique that is used to find out the median element in an unsorted array. In this article, we will implement median of medians algorithm in golanguage, using two methods like the recursive method and the iterative method. Explanation The Median of Medians algorithm, an efficient technique for determining the median value in an unsorted array. It introduces two distinct methods: the recursive approach and the iterative approach. Recursive Method: The findMedianRecursive function is introduced to ... Read More

97 Views
The Rabin−Karp algorithm in Golang is a powerful string searching algorithm used to efficiently locate a pattern within a larger text. In this article, we need to implement Rabin Karp algorithm in golanguage that will enable efficient pattern matching and showcasing the flexibility of this algorithm in Golang. We can use methods like the single function approach as well as using the modular approach. Pattern Matching Let us assume we have the text: “ABCABCDABCABC” and the pattern “ABC”, so by implement Rabin Karp algorithm in golanguage we can find out how many times and where this pattern repeat itself in ... Read More

651 Views
Pthreads is an execution model that helps use multiple processors to work at the same time for solving a problem. It is independent of the programming language. Problem Statement Given an array of integers. Find the sum of all the elements of the array using pthreads. Need for Multithreading for Calculating sum The problem is to add the elements in an array. Although it is a simple problem where a linear traversal of the array can do the work very easily with a time complexity of O(n) where n is the number of elements in the array. But if we ... Read More