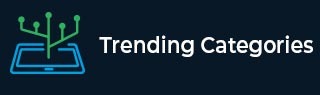
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

299 Views
In this article we will learn about different methods to find the Summation of Random Numbers using Python language. In some scenarios we need to generate some random number and find its summation so we will see basic to improved versions of functions using which we can get this task done. Let’s see some methods to find the summation of random numbers. Method 1. Using Simple Loop Example import random n = 10 rand_nums = [] for _ in range(n): rand_nums.append(random.randint(1, 100)) total = sum(rand_nums) print("Random Numbers:", rand_nums) print("Summation of random numbers:", total) Output Random Numbers: ... Read More

152 Views
In this article we will learn about Queue.LIFOQueue vs Collections.Deque in Python programming language. When we need to manage our data using the last in first out method then we can use these data structures. But to choose one of them we need to know about their functionalities and characteristics. Before that let’s get to know about Queue.LIFOQueue and Collections.Deque. LIFOQueue This class is part of the queue module. It works as stack data structure and it is considered as thread safe means we can talk between the different threads at the same time. Here are some specifications of ... Read More

206 Views
Otto Cycle An air standard cycle called the Otto Cycle is employed in spark ignition (SI) engines. It comprises of two reversible adiabatic processes and two isochoric processes (constant volume), totaling four processes. When the work interactions take place in reversible adiabatic processes, the heat addition (2-3) and rejection (4-1) occur isochorically (3-4 and 1-2). The Otto cycle's schematic is shown in Figure given below. To model the cycle in Python, the input variables considered are maximum pressure $\mathrm{(P_{max})}$, minimum pressure $\mathrm{(P_{min})}$, maximum volume $\mathrm{(V_{max})}$, compression ratio (r), and adiabatic exponent $\mathrm{(\gamma)}$. Table 2 explains the thermodynamic computations of ... Read More

128 Views
In this article we will get to know how we can replace sublist value with new list. You may have faced this problem while working with manipulation of list. Here we will see various methods using which we can replace the sublist with other list. Let’s get to know this using below example − listItem = [1, 2, 3, 4, 5, 6] new_list = [7, 8, 9] Here we have list named as listItem which contains some elements and we have another list named as new_list. So, we want to replace the sublist of listItem with new_list. Take ... Read More

77 Views
In this article we will learn how we can replace rear (word at the end of the string) word with any other given word. You may have seen this problem while doing manipulation of string. Here we will see various methods for replacing the rear word in string with given word. Let’s get to know this using below example − string_txt = "This cat is running very Fast" Here we have a string string_txt which contains sentences and we want to replace the last word which is "Fast" into "Slow" so that the resulting string will be like. ... Read More

52 Views
In this article we will learn about replacement of punctuation with letter k in the string. You may have seen this problem while performing text processing or data cleaning task in the python where you need to replace punctuations with specific characters or any symbol. Here we will see various methods for replacing the punctuations with given text "k" Let’s get to know this using below example − string = "Welcome, to, * the website ! aliens" Here we have a string which contains punctuations like [, * !] and we want to replace that with text "k". ... Read More

2K+ Views
Gauss Seidel Method is the iterative method to solve any system of linear equations. Though the method is very much similar to the Jacobi's method but the values of unknown (x) obtained in an iteration are used in the same iteration in Gauss Seidel whereas, in Jacobi's method they are used in the next iteration level. The updation of x in the same step speeds up the convergence rate. A system of liner equation can be written as − $$\mathrm{a_{1, 1}x_{1} \: + \: a_{1, 2}x_{2} \: + \: \dotso \: + \: a_{1, n}x_{n} \: = \: b_{1}}$$ $$\mathrm{a_{2, ... Read More

1K+ Views
In this article we will see method to replace negative values with zero. If we talk about data analysis then handling negative values is very crucial step to ensure meaningful calculation. So, here you will learn about various methods using which we can replace negative values with 0. Method 1. Using Traversal and List Comprehension. Example import numpy as np arr = np.array([-12, 32, -34, 42, -53, 88]) arr = [0 if x < 0 else x for x in arr] arr = np.array(arr) print("array with replaced values is: ", arr) Output array with replaced values ... Read More

271 Views
In this article we will see method to replace NaN (Not a Number) value with the average of columns. If we talk about data analysis then handling NaN value is very crucial step. So, here you will learn about various methods using which we can replace NaN (Not a Number) value with the average of columns. Method 1: Using Numpy.nanmean(). Example import numpy as np arr = np.array([[1, 2, np.nan], [4, np.nan, 6], [np.nan, 8, 9]]) col_means = np.nanmean(arr, axis=0) arr_filled = np.where(np.isnan(arr), col_means, arr) print("Column mean: ", col_means) print("Final array: ... Read More

4K+ Views
In this article we will see method to replace the multiple characters at once in any string. While working with programs where we face such situation when we will have to replace any particular character simultaneously at all of its occurences. Suppose a situation in which you have an article in which it contains one word named as “word” which is having multiple occurrences and you want to convert that “word” into “work” so you can do this manually but it is very bad practice of doing this work one by one. So, we will look at multiple methods using ... Read More