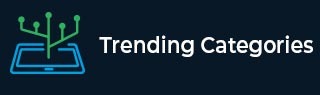
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

3K+ Views
In Python, an empty string is a string with no characters in it. It is represented by a pair of single quotes '' or a pair of double quotes "". An empty string is different from a null value, which is a special value representing the absence of any object. An empty string can be used in various ways, such as initializing a string variable or checking if a string is empty. The most elegant way to check if a string is empty in Python is to simply use the Boolean evaluation of the string. Here are some examples: Using ... Read More

6K+ Views
In this article, we are going to see how to check if multiple strings exist in another string in Python. Imagine a scenario where we must satisfy 50 requirements in order to work. To determine whether conditions are true, we typically employ conditional statements (if, if−else, elif, nested if). However, with so many conditions, the code grows long and unreadable due to the excessive use of if statements. Python's any() function is the only option for such circumstances, thus programmers must use it. The first approach is by writing a function to check for any multiple strings’ existence and then ... Read More

9K+ Views
In this article, we are going to focus on how to create a long multi−line string in Python. The first approach is by using triple quotes, we should add a triple quote then we can add separate line inside the quotes, the multi-line string is created. Using triple quotes in Python, it is possible to span strings across many lines. It can also be applied to lengthy code comments. Triple quotes can also contain special characters like TAB, verbatim, or NEWLINES. As the name would imply, the syntax consists of three single or double quotes placed consecutively. Example In the ... Read More

15K+ Views
In this article, we are going to find out how to execute a string containing Python code in Python. To execute a string containing Python code we should take the input string as multi-line input using triple quotes, then we will use the inbuilt function exec(). This will take a string as input and returns the output of the code that is present inside the string. The exec() function is used to execute Python programmes dynamically. These programmes can be either strings or object code. If it's a string, it's translated into a series of Python statements that are then ... Read More

15K+ Views
In this article, we are going to find out how to get a function name as a string in Python. The first approach is by using the function property __name__. This property will return the name of the function when the property is called. This is only present in Python 3.x version. Python does not have a main() function, so when the command to run a Python program is given to the interpreter, the level 0 indented code will be executed. Before that, though, it will define a few unique variables. One such unique variable is __name__. The interpreter sets ... Read More

548 Views
In Python, a string is a sequence of characters. It's a type of data that represents text values, such as words, sentences, or even entire documents. Strings in Python are enclosed in either single quotes ('...') or double quotes ("..."), and can include alphanumeric characters, symbols, whitespace, and more. As an immutable object, a string's ID cannot be changed in Python. As long as a new string that has the same value as the original string (valid only for small string literals), the ID of both the strings will remain same. In Python, small string literals (strings with only ... Read More

730 Views
You generate random strings with upper case letters and digits in Python. Here are three different ways to do it: Using random.choices() and string.ascii_uppercase In this method, we will use the random.choices() function to randomly choose characters from the string.ascii_uppercase and string.digits strings to generate a random string. Example We first import the random and string modules, which we will use to generate random characters and create strings, respectively. We define the length of the random string we want to generate (in this example, it's 10). We define the possible characters that can be used to create the random string, ... Read More

29K+ Views
A string is a collection of characters stored as a single value. Unlike other technologies there is no need to explicitly declare strings in Python (for that matter any variable), you just need to assign strings to a literal this makes Python strings easy to use. In Python, a string is represented by the class named String. This class provides several functions and methods using which you can perform various operations on strings. In this article, we are going to focus on how to get a variable name as a string in Python. Variables are essentially name mappings to objects, ... Read More

42K+ Views
A string is a group of characters that can be used to represent a single word or an entire phrase. Strings are simple to use in Python since they do not require explicit declaration and may be defined with or without a specifier. In Python, the class named string represents the strings. This class provides several built-in methods, using these methods we can perform various operations on strings. In this article, we are going to find out how to convert hex string into an integer in Python. Using the int() method One way to achieve this is using the inbuilt ... Read More