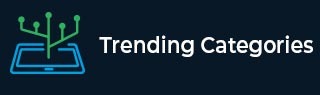
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

2K+ Views
To represent a unicode string as a string of bytes is known as encoding. To convert a string of bytes to a unicode string is known as decoding. You typically encode a unicode string whenever you need to use it for IO, for instance transfer it over the network, or save it to a disk file. You typically decode a string of bytes whenever you receive string data from the network or from a disk file. To encode a string using a given encoding you can do the following: >>>u'æøå'.encode('utf8') '\xc3\x83\xc2\xa6\xc3\x83\xc2\xb8\xc3\x83\xc2\xa5'To decode astring(using the same encoding used to encode it), you need ... Read More

15K+ Views
In this article, we are going to find out how to split a string on whitespace in Python. The first approach is by using the inbuilt method split(). This method splits the given string at our desired delimiter. The split() method accepts a parameter named as a delimiter, it specifies at what character should a string be split. So, we have to send white space as a delimiter to the split() method. This method returns the modified list that is split in white space. Example In the example given below, we are taking a string as input and we are ... Read More

7K+ Views
You can use regexes to remove the ANSI escape sequences from a string in Python. Simply substitute the escape sequences with an empty string using re.sub(). The regex you can use for removing ANSI escape sequences is: '(\x9B|\x1B\[)[0-?]*[ -\/]*[@-~]'.For example, import re def escape_ansi(line): ansi_escape =re.compile(r'(\x9B|\x1B\[)[0-?]*[ -\/]*[@-~]') return ansi_escape.sub('', line) print escape_ansi(line = '\t\u001b[0;35mSomeText\u001b[0m\u001b[0;36m172.18.0.2\u001b[0m') This will give the output: '\tSomeText 172.18.0.2'Read More

295 Views
Concatenating a string and an integer in Python is a common task in programming. Here are three different ways to do it: Using the str() function to convert the integer to a string Example In this method, we define a string called my_string and an integer called my_number. We use the str() function to convert my_number to a string, and then we concatenate the two strings using the + operator. Finally, we print out the resulting string. my_string = "The answer is: " my_number = 42 result = my_string + str(my_number) print(result) Output The answer is: 42 ... Read More

5K+ Views
In Python, you can un-escape a backslash-escaped string using the decode() method or by using the ast module. Here are few different ways to do it: Using decode() method Example In this method, we define a string called escaped_string that contains a backslash-escaped newline character . We convert this string to bytes using the bytes() function and specify the utf-8 encoding. Then, we use the decode() method with the unicode_escape codec to un-escape the backslash-escaped characters in the string. Finally, we print out the resulting un-escaped string. escaped_string = 'This is a backslash-escaped string: foo ' unescaped_string = ... Read More

6K+ Views
In this article, we are going to find out how to concatenate a string with numbers in Python. The first approach is by typecasting the number into a string using typecasting. After typecasting the number, we can concatenate using the ‘+’ operator. Type casting or type conversion in Python are terms used to describe the transformation of one data type into another. For type casting in Python, a large number of functions and methods are supported, including: int(), float(), str(), ord(), hex(), oct(), tuple(), set(), list(), dict(), etc. Example 1 In the example given below, we are taking a string ... Read More

5K+ Views
Floating numbers are the numbers which include a decimal point and in other words are also called as real numbers. By default, the number of decimal points in floating number is 6 but we can modify that using the methods given below. In this article, we are going to find out how to format a floating number to given width in Python. The first approach is by using the format method. We will include the format in a print statement and we will reference using the curly braces and we should mention the number of decimal points inside the curly ... Read More

2K+ Views
Slicing is a technique in Python used to extract a portion of a sequence, such as a string, list, or tuple. Slicing involves specifying the start and end indices of the sequence, and the resulting slice will contain all elements from the start index up to (but not including) the end index. Slicing in Python is denoted by using square brackets [] and providing the start and end indices separated by a colon ‘:’. If the start index is omitted, it is assumed to be 0, and if the end index is omitted, it is assumed to be the length ... Read More

2K+ Views
In Python, escape sequences are special characters that are used to represent certain characters that cannot be easily typed or printed. They are typically represented with a backslash (\) followed by a character that represents the special sequence. For example, the newline character () is used to represent a line break in a string. Here are some common escape sequences used in Python: : newline character \t: tab character ': single quote character ": double quote character In Python, we can process escape sequences in a string using backslashes (\) followed by a character or a combination of characters. Here ... Read More

6K+ Views
In this article, we are going to find out how to remove empty strings from a list of strings in Python.. The first approach is by using the inbuilt method filter(). This method takes input from a list of strings and removes empty strings and returns the updated list. It takes None as the first parameter because we are trying to remove empty spaces and the next parameter is the list of strings. The built-in Python function filter() enables you to process an iterable and extract those elements that meet a specified condition. This action is frequently referred to as ... Read More