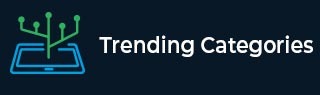
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

1K+ Views
To find the longest repetitive sequence in a string in Python, we can use the following approach: Iterate through each character of the string and compare it with the next character. If they are same, we can increase a counter variable and continue comparing with the next character. If they are not the same, we check if the counter is greater than the length of the longest repetitive sequence we have found so far. If it is, we update the longest repetitive sequence. Reset the counter to 1 and continue with the next character in the string. Here are three ... Read More

5K+ Views
In this article, we are going to find out how to remove all special characters punctuation and spaces from a string in Python. The first approach is by using isalnum() method on each character of the string by iterating it using for loop. We will check if each character is either alphabet or number using isalnum(), if they are not then they will be removed else we will go on to next character. If every character in the string is an alphanumeric character, the isalnum() method returns True (either alphabets or numbers). If not, False is returned. Example In the ... Read More

215 Views
The preferred way to concatenate a string in Python is by using the + operator or the join() method. Here's a step-by-step explanation of each method − Using the + operator To concatenate two strings using the + operator, simply put the strings next to each other with a + sign in between them. Example In this example, we concatenate the name variable with the greeting string using the + operator. The resulting string is "Hello, John!". name = "John" greeting = "Hello, " + name + "!" print(greeting) Output Hello, John! Using multiple + operators You ... Read More

4K+ Views
In this article, we are going to find out how to extract a substring from inside a string in Python. The first approach is by using Regular expressions. A string of characters that creates a search pattern is known as a regex, or regular expression. RegEx can be used to determine whether a string includes a given search pattern. We will use re.search method of Regular Expressions and we will search for the given string that is given by the regular expression and we will extract it. Example 1 In the example given below, we are taking a string as ... Read More

26K+ Views
In this article, we are going to find out how to check if the type of a variable is a string in Python. The first approach is by using the isinstance() method. This method takes 2 parameters, the first parameter being the string that we want to test and the next parameter is the keyword str. This method will return True if the given input is a string, otherwise, it returns False. It specifies that when we pass an object and class, or tuple of classes, to the isinstance() method, it will return True if the object's data type matches ... Read More

8K+ Views
In this article, we are going to find out how to remove characters except digits from a string in Python The first approach is by using the if statement in a for loop and joining them using join() method. We will iterate the string using the for loop and then we will check whether each character is a digit or not using if statement, if it is a digit then we will go to next character, otherwise we will replace that character. For iterating repeatedly through a sequence, use a for loop. This functions more like an iterator method seen ... Read More

911 Views
ASCII stands for American Standard Code for Information Interchange. ASCII is a character encoding system that assigns a unique number to each letter, digit, and symbol on a standard keyboard. In Python, each character has a numerical value based on the ASCII code, which can be checked using the ord() function. Understanding ASCII is important when working with text-based data and encoding or decoding text. To check if a string in Python is in ASCII, you can use the built-in string module in Python. Here are some ways you can check if a string is in ASCII: Using the isascii() ... Read More

684 Views
Here are some examples of how to delete a character from a string using Python. Using String Slicing One of the easiest ways to delete a character from a string in Python is to use string slicing. Here's how you can do it: Example In the first line of code, we define a string called "my_string" that contains the text "Hello World". In the second line of code, we remove the character "o" by creating a new string called "new_string". We do this by slicing the original string into two parts - the characters before the "o" and the ... Read More

668 Views
To convert a string to binary, you need to iterate over each character and convert it to binary. Then join these characters together in a single string. You can use format(ord(x), 'b') to format the character x as binary. For example:>>>st = "hello world" >>>' '.join(format(ord(x), 'b') for x in st) '11010001100101 1101100 1101100 1101111 100000 1110111 1101111 1110010 1101100 1100100' You can alsomap all characters to bin(number) using bytearray to get the array of allcharacters in binary. For example:>>>st = "hello world" >>>map(bin, bytearray(st)) ['0b1101000', '0b1100101', '0b1101100', '0b1101100', '0b1101111', '0b100000', '0b1110111', '0b1101111', '0b1110010', '0b1101100', '0b1100100']Read More

8K+ Views
To find all possible permutations of a given string, you can use the itertools module which has a useful method called permutations(iterable[, r]). This method return successive r length permutations of elements in the iterable as tuples. In order to get all the permutations as string, you'll need to iterate over the function call and join the tuples. For example: >>>from itertools import permutations >>>print [''.join(p) for p in permutations('dune')] ['dune', 'duen', 'dnue', 'dneu', 'deun', 'denu', 'udne', 'uden', 'unde', 'uned', 'uedn', 'uend', 'ndue', 'ndeu', 'nude', 'nued', 'nedu', 'neud', 'edun', 'ednu', 'eudn', 'eund', 'endu', 'enud'] If you don't want to use in built method, ... Read More