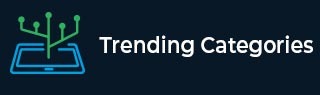
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

2K+ Views
In Python, %r and %s are used as string formatting operators to insert values into a string. The %s operator is used for representing strings, whereas %r is used to represent a string as it would appear in its raw form, including quotes and special characters. Use %s when you want to display a string in a user−friendly way, without any extra quotes or special characters. Use %r when you want to display the exact string representation of a variable, including any quotes or special characters it might contain. Use %s for most situations, as it provides a more user−friendly ... Read More

2K+ Views
You can perform some math operations on Python strings technically, but it may not work the way you expect it to. Here are a few examples: Concatenating Strings with + Example In this example, we concatenate two strings by using the + operator. This works because the + operator is overloaded in Python to work with strings as well as numbers. When we use the + operator with strings, it concatenates them together to form a single string. string1 = "Lorem " string2 = "Ipsum" result = string1 + string2 print(result) Output Lorem Ipsum Multiplying Strings with * ... Read More

4K+ Views
In this article, we are going to find out how to find the nth occurrence of a substring in a string in Python. The first approach is by using the split() method. We have to define a function with arguments as string, substring, and the integer n. By dividing at the substring with max n+1 splits, you may locate the nth occurrence of a substring in a string. If the size of the resultant list is higher than n+1, the substring appears more than n times. The length of the original string minus the length of the last split segment ... Read More

13K+ Views
In this article, we are going to find out how to convert a CSV string into an array. The first approach is by using the split() method. This method takes a parameter, and delimiters the character at which the string should be split. Since the input is a csv, which is comma-separated we will be setting the delimiter as comma and split the string into array. The split() method in Python is a string manipulation function that divides a larger string into multiple smaller strings. The strings are returned as a list by the split() method. Example In the example ... Read More

4K+ Views
In this article, we are going to find out how to remove a list of characters in string in Python. The first approach is by using the replace() method. This method takes 2 parameters, the character we would like to replace and the character with which we are replacing. This method takes a string as input and we will get the modified string as output. The replace string method creates a new string by replacing some of the characters in the given string with new ones. The initial string is unaffected and unchanged. Example In the example given below, we ... Read More

10K+ Views
In this article, we are going to find out how to get integer values from a string in Python. The first approach is by using the filter() method. We will pass the string and the method isdigit() to the filter method. Python has a built-in function called Filter(). An iterable, like a list or dictionary, can have the filter function applied to it to create a new iterator. Based on the criteria you provide, this new iterator can filter out specific elements quite well. The filter() method checks the string for digits and filters out the characters that satisfy the ... Read More

15K+ Views
In this article, we are going to find out how to extract a date from a string in Python. Regular expressions are used in the first technique. Import the re library and install it if it isn't already installed to use it. After importing the re library, we can use the regular expression "\d{4}-\d{2}-\d{2}". To extract a date from a string, you must first understand the format of the date. To extract the date, simply use a regular expression and "datetime.datetime.strptime" to parse it. For example, if you have a date in the format YYYY−MM−DD in a string, you may ... Read More

3K+ Views
In this article, we are going to find out how to scan a string for specific characters in Python. The first approach is by using the ‘in’ keyword. We can use the ‘in’ keyword for checking if a character is present in the string. If it is present in the string, True is returned otherwise, False is returned. The ‘in’ keyword is also used to iterate through a sequence in a for loop which is also used for iterating over a sequence like list, tuple and string or other iterable objects. Example 1 In the example given below, we are ... Read More

2K+ Views
In this article, we are going to find the longest common substring from more than two strings in Python. The first and only approach for finding the longest common substring is by using the Longest common substring Algorithm. We will define a function that accepts 2 strings, then we will iterate over both the strings and find for common subsequence and calculate the length. We will check entire string by iterating over and calculating the length of the sub-sequences and we will return the longest common subsequence. We will get an empty string as output if there are not any ... Read More

1K+ Views
In this article, we are going to focus on checking if a string can be converted to a float in Python. The first approach is by using the float() type cast. We will write a function using exception handling and check if the given string can be converted into string, if it can be converted then True is returned, else False is returned. A floating−point number is returned by the method float() for a given number or text. It will return 0.0 as the floating−point value if no value or a blank parameter is given. Example 1 In this example, ... Read More