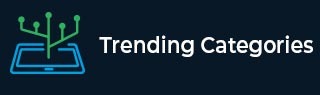
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

611 Views
One efficient way to repeat a string to a certain length in Python is to use the string multiplication operator (*) and string concatenation. Here are some code examples with step−by−step explanations: Using string multiplication operator Example Define the original string and the desired length to repeat it to. Calculate the number of times the string needs to be repeated by dividing the desired length by the length of the string using integer division (//). Multiply the string by the calculated number of repetitions using the string multiplication operator (*). Add any remaining characters to the end of the repeated ... Read More

549 Views
You can use dictionaries to interpolate strings. They have a syntax in which you need to provide the key in the parentheses between the % and the conversion character. For example, if you have a float stored in a key 'cost' and want to format it as '$xxxx.xx', then you'll place '$%(cost).2f' at the place you want to display it.Here is an example of using string formatting in dictionaries to interpolate a string and format a number:>>>print('%(language)s has %(number)03d quote types.' % {'language': "Python", "number": 2}) Python has 002 quote types.You can read up more about string formatting and their ... Read More

5K+ Views
In this article, we are going to find out how to sort the letters in a string alphabetically in Python. The first approach is by using sorted() and join() methods. We send a string as input and we get alphabetically sorted string as output. We need to send the string as parameter to the sorted() method and after that we can join them using the join() method. The sorted() method returns a list after ordering the items of an iterable in either ascending or descending order. Example 1 In the example given below, we are taking a string as input ... Read More

1K+ Views
In Python, a string is a sequence of Unicode characters, while a byte string is a sequence of raw bytes. Here are three examples that demonstrate the difference between a string and a byte string: Creating a String Example In this example, we define a string "Lorem Ipsum" using double quotes. This string is made up of Unicode characters that can be encoded in different ways. # Define a string my_string = "Lorem Ipsum" # Print the string print(my_string) Output Lorem Ipsum Creating a Byte String Example In this example, we define a byte string "Lorem Ipsum" using ... Read More

9K+ Views
In this article, we are going to find out how to convert a string to a list of words in Python. The first approach is to use the built−in method split (). This function separates a text at the delimiter we specify. The delimiter parameter is sent to the split() function, and it indicates where a text should be divided. As a result, we must pass white space to the split() function as a delimiter. This function returns a white-space-separated modified list. Example 1 In the example given below, we are taking a string as input and we are converting ... Read More

6K+ Views
A string is a collection of characters that may be used to represent a single word or an entire phrase. Strings are useful in Python since they don't need to be declared explicitly and may be defined with or without a specifier. For dealing with and accessing strings, Python includes a variety of built-in methods and functions. A string is an object of the String class, which contains multiple methods because everything in Python is an object. In this article, we are going to find out how to split a multi-line string into multiple lines in Python. The first technique ... Read More

1K+ Views
In this article, we are going to find out how to remove a substring from the end of a string in Python. The first approach is by using slicing approach. In this method, we will check if the string is ending with the given substring or not, if it is ending with the given substring then we will slice the string, removing the substring. The ability to access portions of sequences like strings, tuples, and lists in Python is known as slicing. Additionally, you can use them to add, remove, or edit the elements of mutable sequences like lists. Slices ... Read More

2K+ Views
JSON (JavaScript Object Notation) is a lightweight data interchange format used to transmit data between web applications and servers. In Python, JSON data is represented as key-value pairs. The json module provides several methods for working with JSON data in Python, including dumps(), loads(), and dump(). These methods allow you to encode Python objects as JSON strings, decode JSON strings into Python objects, and write JSON data to a file, respectively. JSON data is easy for humans to read and write, and easy for machines to parse and generate. Overall, JSON is an important tool for web developers working with ... Read More

686 Views
In this article, we are going to find out how can we tell if a string repeats itself in Python. The first approach is by using slicing and find(). We want to see if the string we have is made up entirely of repeats of a substring of the string. We can confirm this by looking for a rotation of the string in a pair of strings. After adding a string and checking the root string in this string, except for the last and first character, we may search for the root string. This approach doesn’t work for strings with ... Read More

1K+ Views
In this article, we are going to find out how to do string concatenation without plus operator in Python. The first technique is utilizing the string inbuilt library's join() method. To mix strings with a separator, use this approach. This method produces a string sequence as output. Python's join() method offers the ability to combine iterable components that have been separated by a string operator. To return a string of iterators with the sequence's items connected by a string separator, use the built-in Python join function. Example 1 In the example given below, we are taking 2 strings as input ... Read More