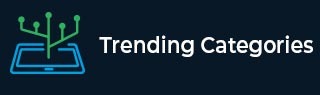
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

602 Views
There are two ways to go about replacing \ with \ or unescaping backslash escaped strings in Python. First is using literal_eval to evaluate the string. Note that in this method you need to surround the string in another layer of quotes. For example:>>> import ast >>> a = '"Hello,world"' >>> print ast.literal_eval(a) Hello, worldAnother way is to use the decode('string_escape') method from the string class. For example,>>> print "Hello,world".decode('string_escape') Hello, world

3K+ Views
This type of sort in which you want to sort on the basis of numbers within string is called natural sort or human sort. For example, if you have the text:['Hello1', 'Hello12', 'Hello29', 'Hello2', 'Hello17', 'Hello25'] Then you want the sorted list to be:['Hello1', 'Hello2', 'Hello12', 'Hello17', 'Hello25', 'Hello29'] and not:['Hello1', 'Hello12', 'Hello17', 'Hello2', 'Hello25', 'Hello29'] To do this we can use the extra parameter that sort() uses. This is a function that is called to calculate the key from the entry in the list. We use regex to extract the number from the string and sort on both text and number. import re ... Read More

4K+ Views
This problem can be solved by reversing the string, reversing the string to be replaced, replacing the string with reverse of string to be replaced with and finally reversing the string to get the result. You can reverse strings by simple slicing notation - [::-1]. To replace the string you can use str.replace(old, new, count). For example, def rreplace(s, old, new): return (s[::-1].replace(old[::-1], new[::-1], 1))[::-1] rreplace('Helloworld, hello world, hello world', 'hello', 'hi') This will give the output:'Hello world, hello world, hi world'Another method by which you can do this is to reverse split the string once on the old string ... Read More

12K+ Views
A string is a collection of characters that can represent a single word or a whole sentence. Unlike Java there is no need to declare Python strings explicitly we can directly assign string value to a literal. A string in Python is represented by a string class which provides several functions and methods using which you can perform various operations on strings. In this article, we are going to find out how to test if a string starts with a capital letter using Python. Using isupper() method One way to achieve this is using the inbuilt string method isupper(). We ... Read More

330 Views
A string is a collection of characters stored as a single value. Un like other technologies there is no need to explicitly declare strings in python (for that matter any variable), you just need to assign strings to a literal this makes Python strings easy to use. In Python, a string is represented by the class named String. This class provides several functions and methods using which you can perform various operations on strings. In this article, we are going to find out how to check whether a string starts with XYZ in Python. Using startswith() method One way to ... Read More

19K+ Views
A string is a collection of characters that can represent a single word or a whole sentence. Unlike Java there is no need to declare Python strings explicitly we can directly assign string value to a literal. A string in Python is represented by a string class which provides several functions and methods using which you can perform various operations on strings. In this article, we are going to find out how to wrap long lines in Python. Using parenthesized line breaks One way to achieve this is by using parenthesized line breaks. Using Python's inferred line continuation within parentheses, ... Read More

3K+ Views
In Python 3, the input() function always returns a string, even if the user enters a number. To check if the user input is an integer, you can use a try-except block and attempt to convert the input string to an integer using the int() function. Here are some code examples that demonstrate different ways to check if raw input is an integer in Python 3: Using a try-except block Example In this example, we use a try-except block to attempt to convert the user's input to an integer using the int() function. If the user enters an integer, the ... Read More

186 Views
% is a string formatting operator or interpolation operator. Given format % values (where format is a string), % conversion specifications in format are replaced with zero or more elements of values. The effect is similar to using the sprintf() in the C language. For example, >>> lang = "Python" >>> print "%s is awesome!" % lang Python is awesomeYou can also format numbers with this notation. For example, >>> cost = 128.527 >>> print "The book costs $%.2f at the bookstore" % cost The book costs $128.53 at the bookstoreYou can also use dictionaries to interpolate strings. They have ... Read More

3K+ Views
With a 64-bit Python installation, and 64 GB of memory, a Python 2 string of around 63 GB should be quite feasible. If you can upgrade your memory much beyond that, your maximum feasible strings should get proportionally longer. But this comes with a hit to the runtimes.With a typical 32-bit Python installation, of course, the total memory you can use in your application is limited to something like 2 or 3 GB (depending on OS and configuration), so the longest strings you can use will be much smaller than in 64-bit installations with very high amounts of RAM.

3K+ Views
In Python, the most efficient way to concatenate strings is to use the join() method. This method takes a list of strings and joins them together into a single string. Example: How to use the join() method In this example, we have a list of strings called words. We want to join these strings together into a sentence, so we use the join() method. We pass the list of strings to the method, and we also pass a separator string, which in this case is a space. The join() method then combines all of the strings in the list with ... Read More