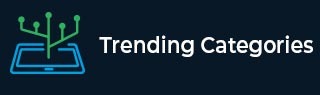
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

5K+ Views
Importing modules is an essential part of programming in Python, and it's something you'll do a lot as you develop your skills. In Python, you can import multiple modules using a few different methods. Here are some examples of how to do it: Import each module on a separate line The simplest way to import multiple modules in Python is to import each one on a separate line. For example, suppose you want to import the math, random, and time modules. You could do it like this: Example This code tells Python to import the math, random, and time modules ... Read More

7K+ Views
In Python, you can use the import statement to use functions or variables defined in another module. Here are some code examples that demonstrate how to use multiple modules with Python import statement: Suppose you have two modules module1.py and module2.py that contain some functions: Example #module1.py def say_hello(name): print("Hello, " + name + "!") #module2.py def say_goodbye(name): print("Goodbye, " + name + "!") To use these modules in another Python program, you can import them using the import statement: import module1 import module2 module1.say_hello("John") module2.say_goodbye("Jane") ... Read More

909 Views
The import statement in Python is used to bring code from external modules or libraries into your program. This is a powerful feature that allows you to reuse code and avoid duplicating code across multiple programs. Here are some examples of how to use the import statement: Import a single module Let's say you want to use the math module in your program, which provides a variety of mathematical functions. To import the math module, you simply use the import statement followed by the name of the module: Example In this example, we import the math module and use the ... Read More

2K+ Views
In Python, both modules and packages are used to organize and structure code, but they serve slightly different purposes. A Python module is a single file containing Python code that can be imported and used in other Python code. A module can define variables, functions, classes, and other Python constructs that can be used by other code. Modules are a great way to organize code and make it reusable across multiple programs. A Python package, on the other hand, is a collection of related Python modules that are organized in a directory hierarchy. A package can contain one or more ... Read More

100 Views
The zfill method is built for left padding zeros in a string. For example:>>> '25'.zfill(6) '000025'We can also use the rjust(width[, fillchar]) method in string class that right justifies the string and pads the left side with given filler char. The default filler char is space but we can provide it '0' as well. You can use it as follows:>>> '25'.rjust(6, '0') '000025'We can also use Python string formatting to achieve this result as follows:>>> print "%06d" % 25 '000025'

2K+ Views
In the English alphabet, vowels are the letters a, e, i, o, and u. Examples of words that contain vowels include "apple", "elephant", "igloo", "octopus", and "umbrella". Consonants are all the other letters in the English alphabet, which include b, c, d, f, g, h, j, k, l, m, n, p, q, r, s, t, v, w, x, y, and z. Examples of words that contain consonants include "ball", "cat", "dog", "fish", "green", and "hat". Here are some code examples with step-by-step explanations to detect vowels and consonants in Python: Using a for loop and conditional statements Example Define the ... Read More

502 Views
Here are some code examples to delete consonants from a string in Python: Using for loop and if statement One way to delete consonants from a string in Python is to loop through each character in the string and check if it is a vowel or not. Here's how you can do it: Example In the first line of code, we define a string called "my_string" that contains the text "Hello World". In the second line of code, we define a string called "vowels" that contains all the vowels (both uppercase and lowercase) in the English alphabet. In the third ... Read More

7K+ Views
Here are three code examples that demonstrate how to check if a character in a string is a letter in Python: Using the isalpha() method The isalpha() method is a built-in method in Python that returns True if all the characters in a string are alphabets (letters) and False otherwise. Example In this example, we have a string "Hello World" and we want to check if the character at index 1 is a letter. We use the isalpha() method to check if the character is a letter and print the appropriate message based on the result. string = "Hello ... Read More

1K+ Views
A Python bytestring is a sequence of bytes. In Python 3, bytestrings are represented using the "bytes" data type. Bytestrings are used to represent binary data that doesn't fit into the Unicode character set, such as images, audio, and video files. Here are some code examples that demonstrate working with Python bytestrings: Creating a bytestring To create a bytestring in Python, we can use the "b" prefix before a string literal. Example In this example, we create a bytestring with the contents "This is a bytestring." and assign it to the variable "bytestring". We then print the bytestring to ... Read More

2K+ Views
To wrap a string in a file in Python, you can follow these steps: Open a file in write mode using the open() function and assign it to a variable. Call the write() function on the file variable and pass the string you want to wrap as an argument. This will write the string to the file. Close the file using the close() function to ensure that the file is properly saved. Here is an example code snippet that demonstrates how to wrap a string in a file: Opening file in write mode Example In the following code, we are ... Read More