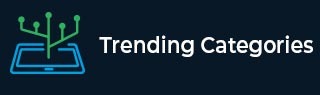
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

2K+ Views
Yes you can iteratively import python modules inside a for loop. You need to have a list of modules you want to import as strings. You can use the inbuilt importlib.import_module(module_name) to import the modules. For example,>>> import importlib >>> modnames = ["os", "sys", "math"] >>> for lib in modnames: ... globals()[lib] = importlib.import_module(lib)The globals() call returns a dict. We can set the lib key for each library as the object returned to us on import of a module.

182 Views
The most popular way to manage python packages (if you're not using your system package manager like homebrew) is to use setuptools and easy_install. It is probably already installed on your system. Use it like this:easy_install djangoeasy_install uses the Python Package Index which is an amazing resource for python developers. Have a look around to see what packages are available.A better and more reliable way to install python modules across platforms is to use pip. If you have Python 2 >=2.7.9 or Python 3 >=3.4 installed from python.org, you will already have pip and setuptools, but will need to upgrade ... Read More

405 Views
Here is a sample project that shows a very good way to structure your projects: https://github.com/kennethreitz/samplemod. The project is about creating the "sample" module. The directory structure looks as follows:README.rst LICENSE setup.py requirements.txt sample/__init__.py sample/core.py sample/helpers.py docs/conf.py docs/index.rst tests/test_basic.py tests/test_advanced.pyThe README.rst file: This file is for giving a brief description of the module, how to set it up, how to use it, etc.LICENSE: Contains license text and any copyright claims.setup.py: It is Python's answer to a multi-platform installer and make file. If you’re familiar with command line installations, then make && make install translates to python setup.py build && python ... Read More
5K+ Views
Python introduces new features with every latest version. Therefore, to check what version is currently being used we need a way to retrieve them. If a user implements features without the correct version, it can generate random issues. We can check the version using few different methods. These methods are discussed below. Using the Sys Module Using sys module, we can retrieve the current python module version. The following lines of code can be used to do so. import sys print(sys.version) Output The output obtained is as follows. 3.10.6 (main, Mar 10 2023, 10:55:28) [GCC 11.3.0] ... Read More

13K+ Views
Python has a capability that allows you to build and store classes and functions for later usage. A module is the name of the file that has these collections of methods and classes. A module may contain more modules. Let’s consider the following example to import multiple modules at once − import sys, os, math, datetime print ('The modules are imported') This imports all four of the following modules at once: datetime(to manipulate dates as date objects), math(consists of functions that can calculate different trigonometric ratios for a given angle), sys (for regular expressions), and os (for operating ... Read More

579 Views
You cannot, in general encapsulate Python modules in a single file. Because doing so would destroy the module searching method that python uses (files and directories). If you are not able to install modules on a machine(due to not having enough permissions), you could use either virtualenv or save the module files in another directory and use the following code to allow Python to search for modules in the given module:>>> import os, sys >>> file_path = 'AdditionalModules/' >>> sys.path.append(os.path.dirname(file_path)) >>> # Now python also searches AdditionalModules folder for importing modules as we have set it on the PYTHONPATH.You can ... Read More

1K+ Views
While installing cygwin, make sure you install the python/python-setuptools from the list. This will install "easy_install" package. Once you have easy_install, you can use it to install pip. Type the following command:$ easy_install-a.b pipYou must replace a.b with your python version which can be 2.7 or 3.4 or whatever else. Now you can use pip to install the module you want. For example, To install the latest version of "SomeProject":$ pip install 'SomeProject'To install a specific version:$ pip install 'SomeProject==1.4'To install greater than or equal to one version and less than another:$ pip install 'SomeProject>=1,Read More

117 Views
Here is a sample project that shows a very good way to structure your projects: https://github.com/kennethreitz/samplemod. The project is about creating the "sample" module. The directory structure looks as follows:README.rst LICENSE setup.py requirements.txt sample/__init__.py sample/core.py sample/helpers.py docs/conf.py docs/index.rst tests/test_basic.py tests/test_advanced.pyThe README.rst file: This file is for giving a brief description of the module, how to set it up, how to use it, etc.LICENSE: Contains license text and any copyright claims.setup.py: It is Python's answer to a multi-platform installer and makes file. If you’re familiar with command-line installations, then make && make install translates to python setup.py build && python setup.py ... Read More

289 Views
You need to have Python, pip, virtualenv, awswebcli and a SSH client installed to set up your Python Development Environment on AWS. You can follow instructions at http://docs.aws.amazon.com/elasticbeanstalk/latest/dg/eb-cli3-install.html to install these.Once you have all those installed, you need to set up a virtual environment so that your global packages do not get polluted. Use the following command to set up a virtual environment:$ virtualenv -p python2.7 /tmp/hello-world Running virtualenv with interpreter /usr/bin/python2.7 New python executable in /tmp/hello-world/bin/python2.7 Also creating executable in /tmp/hello-world/bin/python Installing setuptools, pip...done.Once your virtual environment is ready, start it by running the activate script located in the ... Read More

220 Views
Assuming you have set up Python 3.6, Pipenv and heroku CLI installed locally and are logged in on Heroku from the CLI using the steps mentioned here: https://devcenter.heroku.com/articles/getting-started-with-python#set-up.Your application needs to have a git repository to be deployed to heroku. You need to cd in the directory where the root of your git repo code resides. Now you need to create a heroku application using:$ heroku create Creating lit-bastion-5032 in organization heroku... done, stack is cedar-14http://lit-bastion-5032.herokuapp.com/ | https://git.heroku.com/lit-bastion-5032.gitGit remote heroku addedWhen you create an app, a git remote (called heroku) is also created and associated with your local git repository. ... Read More