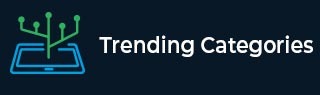
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

6K+ Views
"Binary" files are any files where the format isn't made up of readable characters. Binary files can range from image files like JPEGs or GIFs, audio files like MP3s or binary document formats like Word or PDF. In Python, files are opened in text mode by default. To open files in binary mode, when specifying a mode, add 'b' to it.For examplef = open('my_file.mp3', 'rb') file_content = f.read() f.close() Above code opens my_file.mp3 in binary read mode and stores the file content in file_content variable.
10K+ Views
Python has built in file creation, writing, and reading capabilities. In Python, there are two sorts of files that can be handled: text files and binary files (written in binary language, 0s, and 1s). There are 6 modes of accessing files. To read a text file we use read only ('r') to open a text file for reading. The handle is at the beginning of the document. There are several ways to read a text file into a list or array using python Using open() method The open() function creates a file object from an open file. The filename ... Read More
979 Views
For creating, reading, updating, and removing files, Python includes a number of functions. When reading or writing to a file, the access mode determines the kind of operations that can be performed on the file. Creating a file using Python To create new files or open existing files, we can use the open() function. This function accepts two parameters: a string value representing the name of the file you need to create and a character representing the access mode of the file that is to be created. Following is the syntax of this function. File = open("txt_file_name" ,"access_mode") ... Read More

249 Views
The function raw_input() presents a prompt to the user (the optional arg of raw_input([arg])), gets input from the user and returns the data input by the user in a string. For example, name = raw_input("What isyour name? ") print "Hello, %s." %nameThis differs from input() in that the latter tries to interpret the input given by the user; it is usually best to avoid input() and to stick with raw_input() and custom parsing/conversion code. In Python 3, raw_input() was renamed to input() and can be directly used. For example, name = input("What is your name? ") print("Hello, %s." %name)Read More

239 Views
The function close() closes an open file. For example: f = open('my_file', 'r+') my_file_data = f.read() f.close() The above code opens 'my_file'in read mode then stores the data it reads from my_file in my_file_data and closes the file. When you open a file, the operating system gives a file handle to read/write the file. You need to close it once you are done using the file. If your program encounters an error and doesn't call f.close(), you didn't release the file. To make sure it doesn't happen, you can use with open(...) as syntax as it automatically closes files regardless of whether ... Read More

149 Views
The function open() opens a file. You can use it like: f = open('my_file', 'r+') my_file_data = f.read() f.close() The above code opens 'my_file'in read mode then stores the data it reads from my_file in my_file_data and closes the file. The first argument of open is the name of the file and second one is the open mode. It determines how the file gets opened, for example, – If you want to read the file, pass in r– If you want to read and writethe file, pass in r+– If you want to overwrite thefile, pass in w– If you want to ... Read More

323 Views
In Python 2, there was an alternative syntax for using the print statement for printing that involved using the >> operator, also known as the right shift operator. However, this syntax has been deprecated and removed in Python 3. Therefore, if you see code that uses print >> syntax, it is likely written in Python 2 and will not work in Python 3. The correct syntax in Python 2 for redirecting the output of the print statement to a file-like object is using the print statement followed by the >> operator and the file object. Here are ... Read More

530 Views
In Python, among several functions and other tools that are used to achieve certain functionalities, the print() function happens to be a basic tool for displaying output to the console or terminal. This function allows programmers to achieve several tasks such as, among others, presenting information, messages, variables, and other data to users or for debugging purposes. In this article, we will investigate in detail the functionality and usage of the print() function in Python, through several code examples followed by comprehensive explanations. This will help you to add one more Python skill to your repertoire of code expertise ... Read More

683 Views
The basic way to do output to screen is to use the print statement.>>> print 'Hello, world' Hello, worldTo print multiple things on the same line separated by spaces, use commas between them. For example:>>> print 'Hello, ', 'World' Hello, WorldWhile neither string contained a space, a space was added by the print statement because of the comma between the two objects. Arbitrary data types can also be printed using the same print statement, For example:>>> import os >>> print 1, 0xff, 0777, (1+5j), -0.999, map, sys 1 255 511 (1+5j) -0.999 Objects can be printed on the same line ... Read More

2K+ Views
Currently you cannot add namespaces to XML documents directly as it is not yet supported in the in built Python xml package. So you will need to add namespace as a normal attribute to the tag. For example,import xml.dom.minidom doc = xml.dom.minidom.Document() element = doc.createElementNS('http://hello.world/ns', 'ex:el') element.setAttribute("xmlns:ex", "http://hello.world/ns") doc.appendChild(element) print(doc.toprettyxml())This will give you the document,