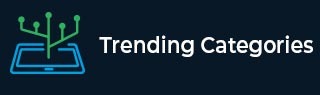
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

3K+ Views
By utilising the pwd, grp, and os modules, you can modify the owner of a file or directory. To obtain the user ID from the user name, to obtain the group ID from the group name string, and to modify the owner, one uses the uid module. Following are the methods to change the owner of a directory using Python − Using os.chown() Method To change the owner and group id of the given path to the specified numeric owner id (UID) and group id(GID), use Python's os.chown() method. Syntax os.chown(filepath, uid, gid, *, dir_fd = None, follow_symlinks = True) ... Read More

6K+ Views
On a platform with the chmod command available, you could call the chmod command like this:>>> import subprocess >>> subprocess.call(['chmod', '-R', '+w', 'my_folder'])If you want to use the os module, you'll have to recursively write it:Using os: import os def change_permissions_recursive(path, mode): for root, dirs, files in os.walk(path, topdown=False): for dir in [os.path.join(root, d) for d in dirs]: os.chmod(dir, mode) for file in [os.path.join(root, f) for f in files]: os.chmod(file, mode) change_permissions_recursive('my_folder', 0o777) This will change the permissions of my_folder, all ... Read More

18K+ Views
The user whose actions are allowed on a file are governed by its file permissions. A file's permissions for reading, writing, and executing are modified when the file's permissions are changed. This article will cover how to change a file's permission in Python. Using os.chmod() Method To modify the permissions of a file, use the os.chmod () method. Syntax Following is the syntax for os.chmod() method − os.chmod(path, mode) Where, path represents the path of the file and mode contains different values as explained below. There is no return value obtained in this method. Os.chmod() modes Following are the ... Read More

8K+ Views
When interacting and working with files and directories in Python, it often becomes necessary to check their permissions to determine and know what operations can be performed on them. Using the os module, you can conveniently check the permissions of a directory in Python. In this article, we will explore the topic of checking directory permissions and accomplish this task in a graded manner, using code examples for understanding the concepts along the way. Before we begin, you better ensure that you have a basic understanding of Python concepts and have Python installation on your system. Checking Directory ... Read More

8K+ Views
File permissions in Python empower you to determine who can perform certain operations on a file. These file attributes include read, write, and execute permissions. The os module in Python, especially the os.chmod() function in the os module, is used to set or modify permissions. The os.stat() function belonging to os module can be used to check the current permissions of a file. Managing and manipulating file permissions is important and critical for security and access control in Python programs. Checking file permissions in Python is important for ensuring the security and integrity of the data stored in files. ... Read More

22K+ Views
Python has built-in functions for creating, reading, and writing files, among other file operations. Normal text files and binary files are the two basic file types that Python can handle. We'll look at how to write content into text files in Python in this article. Steps involved writing multiple lines in a text file using Python Following are the ways to write multiple lines in text file using Python − The open() method must be used to open the file for writing, and the function must be given a file path. The following step is to write to file. ... Read More
4K+ Views
Python has built in file creation, writing, and reading capabilities. In Python, there are two sorts of files that can be handled: text files and binary files (written in binary language, 0s, and 1s). In this article, we'll look at how to write into a file. Firstly, we will have to open the file in write mode using the open() function. Then, the write() method saves a file with the provided text. The file mode and stream location determine where the provided text will be put. "a" − The text will be put at the current location in the ... Read More

4K+ Views
To create a file of a particular size, just seek to the byte number(size) you want to create the file of and write a byte there.For examplewith open('my_file', 'wb') as f: f.seek(1024 * 1024 * 1024) # One GB f.write('0')This creates a sparse file by not actually taking up all that space. To create a full file, you should write the whole file:with open('my_file', 'wb') as f: num_chars = 1024 * 1024 * 1024 f.write('0' * num_chars)

303 Views
The file truncate() method is quite effective. The first reason it is referred to as a method rather than a function is that it comprises the file name (i.e., the object of the file) and a dot operator in addition to other factors (i.e. object of file). Truncation means cutting off. In this case, we define cutting-off in terms of size. Syntax Following is the syntax used to truncate the file to a given size − file.truncate(size) Sending the parameter to this method is not essential. If the size is given as a parameter, it must be given ... Read More

5K+ Views
The read function reads the whole file at once. You can use the readlines function to read the file line by line.ExampleYou can use the following to read the file line by line:f = open('my_file.txt', 'r+') for line in f.readlines(): print line f.close()You can also use the with...open statement to open the file and read line by line. For example,with open('my_file.txt', 'r+') as f: for line in f.readlines(): print line