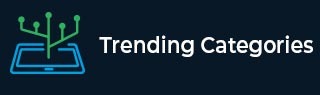
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

4K+ Views
Maximum length of a string is platform dependent and depends upon address space and/or RAM. The maxsize constant defined in sys module returns 263-1 on 64 bit system. >>> import sys >>> sys.maxsize 9223372036854775807 The largest positive integer supported by the platform's Py_ssize_t type, is the maximum size lists, strings, dicts, and many other containers can have.

2K+ Views
Standard distribution of Python contains collections module. It has definitions of high performance container data types. OrderedDict is a sub class of dictionary which remembers the order of entries added in dictionary object. When iterating over an ordered dictionary, the items are returned in the order their keys were first added. >>> from collections import OrderedDict >>> D = {5:'fff', 3:'ttt', 1:'ooo', 4:'bbb', 2:'ddd'} >>> OrderedDict(D.items()) OrderedDict([(5, 'fff'), (3, 'ttt'), (1, 'ooo'), (4, 'bbb'), (2, 'ddd')]) We also need to us sorted() function that sorts elements in an iterable in a specified order. The function takes a function ... Read More

494 Views
Standard distribution of Python contains collections module. It has definitions of high performance container data types. OrderedDict is a sub class of dictionary which remembers the order of entries added in dictionary object. When iterating over an ordered dictionary, the items are returned in the order their keys were first added. >>> from collections import OrderedDict >>> D = {5:'fff', 3:'ttt', 1:'ooo', 4:'bbb', 2:'ddd'} >>> OrderedDict(D.items()) OrderedDict([(5, 'fff'), (3, 'ttt'), (1, 'ooo'), (4, 'bbb'), (2, 'ddd')]) We also need to use sorted() function that sorts elements in an iterable in a specified order. The function takes a function ... Read More

11K+ Views
Dictionaries are a valuable and frequently used data structure in Python. This article tells us how to traverse through a dictionary while performing operations on its key-value pairs. Using dict.items() Method Python's dict.items() method allows you to loop through the dictionary. Each repetition will provide you with both the key and value of each item. Example Following is an example to iterate through a dictionary using dict.items() method − dictionary = { 'Novel': 'Pride and Prejudice', 'year': '1813', 'author': 'Jane Austen', 'character': 'Elizabeth Bennet' ... Read More

84K+ Views
Python has a built-in method called values() that returns a view object. The dictionary's values are listed in the view object. We can use the values() method in Python to retrieve all values from a dictionary. Python's built-in values() method returns a view object that represents a list of dictionaries containing every value. This article explains about the various ways to print all the values of a dictionary in Python. Using dict.values() Method Python's dict.values() method can be used to retrieve the dictionary values, which can then be printed using the print() function. Example Following is an example to print ... Read More

96K+ Views
An unordered collection of data values is what a Python dictionary is. A Python dictionary contains a key: value pair, in contrast to other data structures that only include one value per entry. This article explains about the various ways to print all the keys of a dictionary in Python. Using dict.keys() Method Python's dict.keys() method can be used to retrieve the dictionary keys, which can then be printed using the print() function. A view object that shows a list of every key in the dictionary is the result of the dict.keys() method. The dictionary's elements can be accessed using ... Read More

5K+ Views
File handling is a critical aspect of seamless data management in the realm of computer programming. There are times when choosing the buffer size is crucial when working with files, particularly when handling huge files or carrying out certain actions that need efficient memory utilization. Because of its integrated file-handling features, the strong and flexible programming language Python gives developers the freedom to choose the buffer size when opening files. This extensive essay will examine how to set the buffer size in Python when opening a file. In order to explain the ideas, we will go over them step-by-step and ... Read More

716 Views
File handling plays a pivotal role in efficiently managing data in the realm of computer programming. Python, being a versatile and powerful language, equips developers with robust mechanisms for reading and writing files seamlessly. When working with files, Python offers a range of modifiers that allow for fine-tuning the behavior of the file object. One such modifier, the 'U' modifier, holds particular significance as it influences the way Python handles line endings when reading a file in text mode. In this comprehensive exploration, we will delve into the intricacies of the 'U' modifier and its implications when opening files in ... Read More

11K+ Views
If we open a file in oython using the b modifier. The file is opened in binary mode using the 'b' modifier. Any file whose format doesn't consist of readable characters is referred to as a "binary" file. Binary files include audio files like MP3s, text formats like Word or PDF, and image files like JPEGs or GIFs. Files are automatically opened in text mode in Python. When choosing a mode, include the letter "b" for binary mode. By default, the open() function opens a file in text format. As a result, the "wb" mode opens the file in binary ... Read More