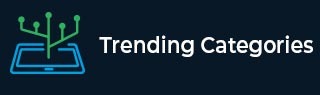
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

253 Views
First, put all dictionary objects in a list object.Initialize a dictionary object to empty directory. This is intended to contain merged directoryExampleUpdate it with each directory item from the list>>> d=[{'a':1, 'b':2, 'c':3}, {'a':1, 'd':2, 'c':'foo'}, {'e':57,'c':3}] >>> d [{'a': 1, 'b': 2, 'c': 3}, {'a': 1, 'd': 2, 'c': 'foo'}, {'e': 57, 'c': 3}] >>> merged={} >>> for x in d: merged.update(x) >>> merged {'a': 1, 'b': 2, 'c': 3, 'd': 2, 'e': 57}

9K+ Views
Given below is a nested directory objectD1={1: {2: {3: 4, 5: 6}, 3: {4: 5, 6: 7}}, 2: {3: {4: 5}, 4: {6: 7}}}ExampleFollowing recursive function is called repetitively if the value component of each item in directory is a directory itself.def iterdict(d): for k,v in d.items(): if isinstance(v, dict): iterdict(v) else: print (k,":",v) iterdict(D1)OutputWhen the initial dictionary object is passed to this function, all the key-value pairs are traversed. The output is:3 4 5 6 4 5 6 7 4 5 6 7

2K+ Views
In Python, a non-white space character is any character that is not a space, tab, or newline. These characters are important for formatting and readability in Python code. Suppose we have a string with both whitespace and non-whitespace characters: We can use the isspace() method to check if each character in the string is a whitespace character In this code, we iterate over each character in the my_string variable and use the isspace() method to determine if the character is a whitespace character or not. If the character is a whitespace character, we print "Whitespace character", and if it is ... Read More

1K+ Views
The regular expressions module in Python provides a powerful tool for pattern matching in strings in Python. Regular expressions, also known as regex, make it possible for us to search, extract, and manipulate text based on specified patterns. One routine and common task in text processing is to identify non−word characters; these include symbols, punctuation marks, and spaces. In this article, we will explore various ways in which we can use regular expressions in Python to identify and match these non−word characters. We will take up a few code examples, each followed by stepwise explanations, to guide you through the ... Read More

2K+ Views
Mastering regular expressions and their ‘re’ module opens up a world of powerful text−processing possibilities in Python. Regular expressions, often called regex, make it possible for us to identify, search for, and manipulate specific patterns within strings. One common task that we often come across in our work is matching a particular word in a text using regular expressions. In this article, we will take a deep dive into the art of using regular expressions in Python to find and match words in strings. We'll explore this domain using a few code examples, each followed by stepwise explanations, and this ... Read More

159 Views
A dictionary object is mutable. Hence one dictionary object can be used as a value component of a key. So we can create a nested dictionary object another dictionary object is defined as value associated with key.>>>> students={"student1":{"name":"Raaj", "age":23, "subjects":["Phy", "Che", "maths"],"GPA":8.5},"student2":{"name":"Kiran", "age":21, "subjects":["Phy", "Che", "bio"],"GPA":8.25}}

100 Views
You can use any Python aware editor to write Python script. Standard distribution of Python comes with IDLE module which is an integrated development and learning environment. Start IDLE and open a new file from file menu. In the editor page enter print (“Hello World!”) Save the script as hello.py and execute it from Run menu to get Hello world message on console. This is your first program. It can also be run from command prompt c:\user>python hello.py
719 Views
In python, a dictionary is an unordered collection of data which is used to store data values such as map unlike other datatypes that store only single values. Keys of a dictionary must be unique and of immutable data type such as Strings, Integers, and tuples, but the key values can be repeated and be of any type. Dictionaries are mutable, therefore keys can be added or removed even after defining a dictionary in python. They are many ways to remove a key from a dictionary, following are few ways. Using pop(key, d) The pop(key, d) method returns the value ... Read More

757 Views
Dictionary is an unordered collection of key-value pairs. Each element is not identified by positional index. Moreover, the fact that key can’t be repeated, we simply use a new key and assign a value to it so that a new pair will be added to dictionary. >>> D1 = {1: 'a', 2: 'b', 3: 'c', 'x': 1, 'y': 2, 'z': 3} >>> D1[10] = 'z' >>> D1 {1: 'a', 2: 'b', 3: 'c', 'x': 1, 'y': 2, 'z': 3, 10: 'z'}

109 Views
Built-in dictionary class has update() method which merges elements of argument dictionary object with calling dictionary object. >>> a = {1:'a', 2:'b', 3:'c'} >>> b = {'x':1,'y':2, 'z':3} >>> a.update(b) >>> a {1: 'a', 2: 'b', 3: 'c', 'x': 1, 'y': 2, 'z': 3} From Python 3.5 onwards, another syntax to merge two dictionaries is available >>> a = {1:'a', 2:'b', 3:'c'} >>> b = {'x':1,'y':2, 'z':3} >>> c = {**a, **b} >>> c {1: 'a', 2: 'b', 3: 'c', 'x': 1, 'y': 2, 'z': 3}