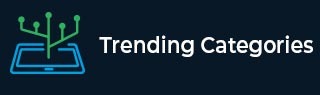
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

10K+ Views
A regular expression is a group of characters that allows you to use a search pattern to find a string or a set of strings. RegEx is another name for regular expressions. The re module in Python is used to work with regular expressions. To match the pattern over multiple lines in python by using regular expression, we use .* regular expression. Here, , indicates paragraph tag in HTML5. Implies zero or more occurrence of characters. . is a special character that matches all characters, including newline characters with the help of re.DOTALL flag. Generally, in regular ... Read More

11K+ Views
A regular expression in Python is a set of characters that allows you to use a search pattern to find a string or a group of strings. RegEx is another term for regular expressions. Regular expressions are worked within Python using the re package. To match with the end of the string in python by using a regular expression, we use ^/w+$ regular expression. Here, $ implies the start with. /w returns a match where the string contains any word characters (a z, A Z, 0 9, and underscore character). + indicates one or more occurrences of a character. ... Read More

9K+ Views
A regular expression in Python is a group of characters that allows you to use a search pattern to find a string or set of strings. RegEx is a term for regular expressions. To work with regular expressions in Python, utilise the re package. To match with the beginning of the string in python by using regular expression, we use ^/w+ regular expression. Here, ^ implies the start with. /w returns a match where the string contains any word characters (a z, A Z, 0 9, and underscore character). + indicates one or more occurrence of a character. ... Read More

383 Views
A tuple is a collection of python objects that are separated by commas which are ordered and immutable. Tuples are sequences, just like lists. The differences between tuples and lists are, that tuples cannot be changed unlike lists and tuples use parentheses, whereas lists use square brackets. Let us see about the creation of tuples in a detailed way. Empty Tuple Empty tuple means a tuple with no elements. Example Following is a way, to can create an empty tuple. temp=() print(temp) Output The following output is obtained on executing the above program. () Non Empty Tuple It ... Read More

2K+ Views
List is one of the frequently used data structures in python. A list is a data structure in python that is mutable and has ordered sequence of elements. Following is a list of integer values Example lis= [5, 10, 15, 20, 25] print(lis) Output If you execute the above snippet, it produces the following output. [5, 10, 15, 20, 25] Let us discuss various ways to split a list into evenly sized chunks in Python. Using slice operator You can print the list of elements with equally sized chunks, and they can be solved simply by using the ... Read More

4K+ Views
In this article, we will show you how to get the first 100 characters of a string in python. The following are the 4 different methods to accomplish this task − Using While Loop Using For Loop Using Slicing Using slice() Function Assume we have taken an input string. We will return the first 100 characters of the input string using the above-specified methods. Method 1: Using While Loop Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Create a variable to store the input string. Create another variable to store ... Read More
220 Views
A list is a data structure in Python that is a mutable, or changeable, ordered sequence of elements. A list's items are any elements or values that are contained within it. Lists are defined by having values inside square brackets [], just as strings are defined by characters inside quotations. They are used to store multiple items in a single variable. In Python, strings are among the most widely used types. We can create them simply by enclosing characters in quotes. Python treats single quotes the same as double quotes. Creating strings is as simple as assigning a value to ... Read More

50K+ Views
In Python, a list is an ordered sequence that can hold several object types such as integer, character, or float. In other programming languages, a list is equivalent to an array. Square brackets [] are used to denote it, and a comma (, ) is used to divide two items in the list. In this article, we will show you how to get a comma-separated string from a list using python. − Using join() method Using join() & map() Functions Using join() & List comprehension Assume we have taken a list containing some random elements. We will return ... Read More

99 Views
Python has an in-built join() function that returns a string by joining elements in a sequence object by inserting separator between elements. If we need a string without any separator, we initialize it with null string>>> lst=['h','e','l','l','o'] >>> str='' >>> str.join(lst) 'hello'

209 Views
A complex number is a pair of real numbers a and b, most often written as a+bi, or a+ib, where i is called the imaginary unit and acts as a label for the second term. Mathematically, i2 = -1. Sometimes, j is used instead of i.Here’s how a complex number is assigned to a variable:>>> a=5+6j >>> a (5+6j) >>> type(a) Python has a built-in function complex() which returns a complex data type.complex(x) returns a complex number with x as real part and imaginary part as zero. complex(x, y) returns a complex number with x as real part and y ... Read More