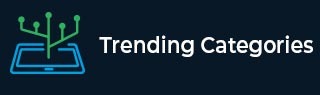
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

425 Views
Python includes a built-in package i.e random module for generating random numbers. In this article, we will show you how to generate random numbers in python using different methods − Using random.seed() method Using random.randrange() method Using random.randint() method Using random.random() Using random.choice() method Using random.uniform() method Method 1: Using random.seed() method The random number generator is initialized using the seed() method. To generate a random number, the random number generator requires a starting numeric value (a seed value). NOTE − The random number generator utilizes the current system time by default. To change the random number generator's ... Read More

6K+ Views
Python is not a "statically typed" programming language. We do not need to define variables or their types before utilizing them. Once we initially assign a value to a variable, it is said to be created. Each variable is assigned with a memory location. The assignment operator (=) assigns the value provided to right to the variable name which is at its left. Syntax The syntax of the assignment operator is shown below. var_name = value Example The following is the example which shows the usage of the assignment operator. Name = ‘Tutorialspoint’ In Python, variable is really ... Read More

5K+ Views
In this article we will discuss different ways to assign values to variables in a list using loops in python. Using simple loop iterations In this method we use the for loop for appending elements into the lists. When we do not enter any element into the list and stop appending, then we just press the enter key. To append the elements into the list we use the append() method. Example 1 The following is an example to assign values to the variables in a list using a loop in python using append() method. L=[] while True: ... Read More

34K+ Views
The Python tuple elements are enclosed inside the parenthesis, while dictionary elements are present in the form of a key-value pair and are enclosed between curly brackets. In this article, we will show you how to convert a Python tuple into a dictionary. The following are the methods to convert a tuple into a dictionary − Using dict() function Using Dictionary Comprehension and enumerate() function Using zip() and dict() functions Assume we have taken a tuple containing some elements. We will convert the input tuple into a python dictionary and return it using different methods as specified above. ... Read More

852 Views
Python provides a variety of data structure collections such as lists, tuples, sets, and dictionaries. However, these tuples are very similar to lists. Because lists are a commonly used data structure, developers frequently mistake how tuples differ from lists. Python tuples, like lists, are a collection of items of any data type, but tuples are immutable, which means that once assigned, we cannot change the elements of the tuple or the tuple itself, whereas list elements are mutable In this article, we will explain to you what is a tuple in python and the various operations on it. Creating a ... Read More

241 Views
Index in python refers to the position of the element within an ordered list. The starting element is of zero index and the last element is of n 1 index where n is the length of the list. In this tutorial, we will look at how to find out the index of an element in a list in Python. They are different methods to retrieve the index of an element. Using index() method Three arguments can be passed to the list index() method in Python − element − element that has to be found. start (optional) − Begin your ... Read More

156 Views
Since Python 3.0, it is no longer possible to define unpacked tuple as a parameter in a function (PEP 3113). It means if you try to define a function as below −def fn(a, (b, c)): passPython interpreter displays syntax error at first bracket of tuple. Instead, define tuple objects as parameter and unpack inside the function. In following code, two tuple objects representing x and y coordinates of two points are passed as parameters to calculate distance between the two. Before calculating, the tuple objects are unpacked in respective x and y coordinates.def hypot(p1, p2): x1, y1=p1 ... Read More

1K+ Views
The following code shows how space is added before and after the pipe '|' character in given string. Exampleimport re regex = r'\b[|:]\b' s = "abracadabra abraca|dabara | abra cadabra abra ca dabra abra ca dabra abra" print(re.sub(regex, ' \g ', s))OutputThis gives the outputabracadabra abraca | dabara | abra cadabra abra ca dabra abra ca dabra abra

715 Views
Python's built−in module 're' provides a powerful tool for working with text data, and regular expressions (regex) are a crucial part of it. However, sometimes you might need to use alternative methods to perform text manipulation tasks that don't involve regex. In this article, we'll explore five code examples that demonstrate how to use alternative methods to perform text manipulation tasks in Python, along with stepwise explanations and illustrations. Regular expressions are an incredibly powerful tool for working with text in Python. They allow us to search, manipulate, and process text in ways that would be otherwise time−consuming and complex. ... Read More

543 Views
GroupingWe group part of a regular expression by surrounding it with parentheses. This is how we apply operators to the complete group instead of a single character.Capturing GroupsParentheses not only group sub-expressions but they also create backreferences. The part of the string matched by the grouped part of the regular expression, is stored in a backreference. With the help of backreferences, we reuse parts of regular expressions. In practical applications, we often need regular expressions that can match any one of two or more alternatives. Also, we sometimes want a quantifier to apply to several expressions. All of these can be ... Read More