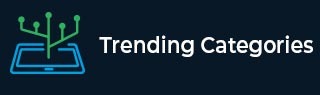
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

2K+ Views
In Python, a list is an ordered sequence that can hold several object types such as integer, character, or float. In other programming languages, a list is equivalent to an array. In this article, we will show you how to reverse the objects/elements in a list using python. The following are the 4 different methods to accomplish this task − Using built-in reverse() method Using the builtin reversed() method Using Slicing Using For Loop, pop() and append() Functions Assume we have taken a list containing some elements. We will return a new list after reversing all the items ... Read More

45K+ Views
To remove items (elements) from a list in Python, use the list functions clear(), pop(), and remove(). You can also delete items with the del statement by specifying a position or range with an index or slice. In this article, we will show you how to remove an object/element from a list using Python. The following are the 4 different methods to accomplish this task − Using remove() method Using del keyword Using pop() method Using clear() method Assume we have taken a list containing some elements. We will return a resultant list after removing the given item ... Read More

33K+ Views
In Python, a list is an ordered sequence that can hold several object types such as integer, character, or float. In other programming languages, a list is equivalent to an array. In this article, we will show you how to insert an item/object in a list at a given position using python. − Insert an item at a specific position in a list Insert an item at the first position in the list Insert an item at the last/end position in the list Assume we have taken a list containing some elements. We will insert an object in ... Read More

4K+ Views
In this article, we will show you how to find the index of an object in a given input list using python. Below are the 3 different methods to accomplish this task − Using index() method Using For Loop Using List comprehension and enumerate() Function Assume we have taken a list containing some elements. We will return the index of a specified object in a given input list. Method 1: Using index() method The index() function in Python allows you to find the index position of an element or item within a string of characters or a list ... Read More

4K+ Views
List is one of the most commonly used data structures provided by python. List is a data structure in python that is mutable and has an ordered sequence of elements. Following is a list of integer values − Example Following is a list of integer values. lis= [1, 2, 7, 5, 9, 1, 4, 8, 10, 1] print(lis) Output If you execute the above snippet, it produces the following output. [1, 2, 7, 5, 9, 1, 4, 8, 10, 1] In this article, we will discuss how to find the total count of occurrences of an object in ... Read More

16K+ Views
List is one of the most commonly used data structures provided by python. List is a data structure in Python that is mutable and has an ordered sequence of elements. Following is a list of integer values Example Following is a list of integer values. lis= [11, 22, 33, 44, 55] print(lis) Output If you execute the above snippet, it produces the following output. [11, 22, 33, 44, 55] In this article, we will discuss how to add objects to a list and the different ways to add objects such as append(), insert(), and extend() methods in Python. ... Read More

2K+ Views
List is one of the most commonly used data structures provided by python. List is a data structure in python that is mutable and has an ordered sequence of elements. Following is a list of integer values − Example Following is a list of integer values. lis= [12, 22, 32, 54, 15] print(lis) Output If you execute the above snippet, it produces the following output. [12, 22, 32, 54, 15] In this article, we will look at different ways to find the minimum of the list in Python. For the above list, the minimum element of the list ... Read More

9K+ Views
List is one of the most commonly used data structures provided by python. List is a data structure in Python that is mutable and has an ordered sequence of elements. Following is a list of integer values Example Following is a list of integer values. lis= [1, 2, 3, 4, 5] print(lis) Output If you execute the above snippet, it produces the following output. [1, 2, 3, 4, 5] In this article, we will look at different ways to find the maximum of the list in Python. For the above list, the maximum element of the list is ... Read More

2K+ Views
Character classA "character class", or a "character set", is a set of characters put in square brackets. The regex engine matches only one out of several characters in the character class or character set. We place the characters we want to match between square brackets. If you want to match any vowel, we use the character set [aeiou].A character class or set matches only a single character. The order of the characters inside a character class or set does not matter. The results are identical.We use a hyphen inside a character class to specify a range of characters. [0-9] matches ... Read More

563 Views
You can import any built-in module while writing Python script using TutorialsPoint's online IDE. For example you can import time, sys, os, re modules. However, the modules that are not bundled with the distribution - the one which needs to be installed using package managers like pip or conda - can not be imported.