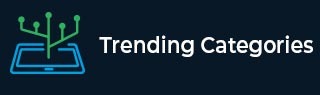
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

96K+ Views
In this article, we will show you how to format date and time in python. The datetime module in Python offers methods for working with date and time values. To use this module, we must first import it using the following import keyword− import datetime strftime function() The strftime() function returns a formatted date and time. It accepts a format string that you can use to get the result you want. The following are the directives that it supports. Directive Meaning %a Locale's abbreviated weekday name %B Locale's full month name. ... Read More

1K+ Views
You can get the current date and time using multiple ways. The easiest way is to use the datetime module. It has a function, now, that gives the current date and time. exampleimport datetime now = datetime.datetime.now() print("Current date and time: ") print(str(now))OutputThis will give the output −2017-12-29 11:24:48.042720You can also get the formatted date and time using strftime function. It accepts a format string that you can use to get your desired output. Following are the directives supported by it.Directive Meaning%a Locale's abbreviated weekday name.%A Locale's full weekday name.%b Locale's abbreviated month name. %BLocale's full month name. ... Read More

129 Views
The timetuple() method of datetime.date instances returns an object of type time.struct_time. The struct_time is a named tuple object (A named tuple object has attributes that can be accessed by an index or by name). exampleYou can print the complete time tuple by simply passing it to print.import datetime todaysDate = datetime.date.today() timeTuple = todaysDate.timetuple() print(timeTuple)OutputThis will give the output −time.struct_time(tm_year=2017, tm_mon=12, tm_mday=28, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=362, tm_isdst=-1)

2K+ Views
To get the timer ticks at a given time, use the clock. The python docs state that this function should be used for benchmarking purposes. Exampleimport time t0= time.clock() print("Hello") t1 = time.clock() - t0 print("Time elapsed: ", t1 - t0) # CPU seconds elapsed (floating point)OutputThis will give the output −Time elapsed: 0.0009403145040156798Note that different systems will have different accuracy based on their internal clock setup (ticks per second). but it's generally at least under 20ms. Also note that clock returns different things on different platforms. On Unix, it returns the current processor time as a floating point number expressed ... Read More

2K+ Views
The floating-point numbers in units of seconds for time interval are indicated by Tick in python. Particular instants in time are expressed in seconds since 12:00am, January 1, 1970(epoch). You can use the time module to use functions for working with times, and for converting between representations. For example, if you want to print the current time in ticks, you'd write:Exampleimport time ticks = time.time() print("Ticks since 12:00am, January 1, 1970: ", ticks)OutputThis will give the output −Ticks since 12:00 am, January 1, 1970: 1514482031.2905385

16K+ Views
In this article, we'll show you how to use several ways to extract a list of all the values from a Python dictionary. Using the following methods, we may obtain a list of all the values from a Python dictionary − Using dict.values() & list() functions Using [] and * Using List comprehension Using append() function & For loop Assume we have taken an example dictionary. We will return the list of all the values from a python dictionary using different methods as specified above. Method 1: Using dict.values() & list() functions To obtain the list, we can ... Read More

42K+ Views
In this article, we will show you how to get a list of all the keys from a Python dictionary using various methods. We can get the list of all the keys from a Python dictionary using the following methods − Using dict.keys() method Using list() & dict.keys() function Using List comprehension Using the Unpacking operator(*) Using append() function & For loop Assume we have taken an example dictionary. We will return the list of all the keys from a Python dictionary using different methods as specified above. Method 1: Using dict.keys() method In Python Dictionary, the dict.keys() ... Read More

29K+ Views
In this article, we will show you how to get a value for the given key from a dictionary in Python. Below are the 4 different methods to accomplish this task − Using dictionary indexing Using dict.get() method Using keys() Function Using items() Function Assume we have taken a dictionary containing key-value pairs. We will return the value for a given key from a given input dictionary. Method 1: Using dictionary indexing In Python, we can retrieve the value from a dictionary by using dict[key]. Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired ... Read More

1K+ Views
A dictionary maintains mappings of unique keys to values in an unordered and mutable manner. In python, dictionaries are a unique data structure, and the data values are stored in key:value pairs using dictionaries. Dictionaries are written with curly brackets and have keys and values. Dictionaries are now ordered as of Python 3.7. Dictionaries in Python 3.6 and before are not sorted. Example In the following example, companyname, and tagline are the keys, and Tutorialspoint, simplyeasylearning are the values respectively. thisdict = { "companyname": "Tutorialspoint", "tagline" : "simplyeasylearning", } print(thisdict) Output The above ... Read More

2K+ Views
A dictionary is a Python is a container that maintains mappings of unique keys to values in an unordered and mutable manner. Data values are stored in key:value pairs using dictionaries. Dictionaries are written with curly brackets and have keys and values. Dictionaries are now ordered as of Python 3.7. Dictionaries in Python 3.6 and before are not sorted. Example This example shows the working of a dictionary in python. thisdict = { "companyname": "Tutorialspoint", "tagline" : "simplyeasylearning", } print(thisdict) Output The above code produces the following results {'companyname': 'Tutorialspoint', 'tagline': 'simplyeasylearning'} ... Read More