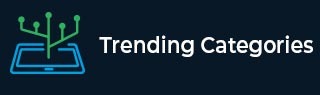
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

292 Views
Special character dot '.'(Dot.) In the default mode, this matches any character except a newline. If the DOTALL flag has been specified, this matches any character including a newline.Special character '?'Causes the resulting RE to match 0 or 1 repetitions of the preceding RE. ab? will match either ‘a’ or ‘ab’Special character asterisk'*"Causes the resulting RE to match 0 or more repetitions of the preceding RE, as many repetitions as are possible. ab* will match ‘a’, ‘ab’, or ‘a’ followed by any number of ‘b’s.

182 Views
Here are two basic examples of Python regular expressionsThe re.match() method finds match if it occurs at start of the string. For example, calling match() on the string ‘TP Tutorials Point TP’ and looking for a pattern ‘TP’ will match. However, if we look for only Tutorials, the pattern will not match. Let’s check the code.Exampleimport re result = re.match(r'TP', 'TP Tutorials Point TP') print resultOutputThe re.search() method is similar to re.match() but it doesn’t limit us to find matches at the beginning of the string only. Unlike in re.match() method, here searching for pattern ‘Tutorials’ in the string ‘TP ... Read More

9K+ Views
We must first understand some regular expression fundamentals as we will use them. There are various ways to declare patterns in regular expressions, which might make them appear complex but are pretty simple. Regular expressions are patterns that can be used to match strings that adhere to that pattern. You need to read the following article to learn how regular expressions operate. You may commonly extract dates from a given text when learning to code. If you are automating a Python script and need to extract specific numerical figures from a CSV file, if you are a data scientist and ... Read More

1K+ Views
If we want to extract all numbers/digits individually from given text we use the following regexExampleimport re s = '12345 abcdf 67' result=re.findall(r'\d', s) print resultOutput['1', '2', '3', '4', '5', '6', '7']If we want to extract groups of numbers/digits from given text we use the following regexExampleimport re s = '12345 abcdf 67' result=re.findall(r'\d+', s) print resultOutput['12345', '67']

22K+ Views
Classes that encompass a collection of characters are known as regular expression classes. One of these classes, d, which matches any decimal digit, will be used. Learning how to split data may be valuable. Data arrives in various kinds and sizes, and it's sometimes not as clean as we'd like. You frequently wish to divide a string by more than one delimiter to make it easier to deal with. The built-in regular expression library re is the easiest way to split a string. The library has a.split() function that works similarly to the above example. This approach stands out since ... Read More

236 Views
The re.split() methodre.split(pattern, string, [maxsplit=0]):This methods helps to split string by the occurrences of given pattern.Exampleimport re result=re.split(r'a', 'Dynamics') print resultOutput['Dyn', 'mics']Above, we have split the string “Dynamics” by “a”. Method split() has another argument “maxsplit“. It has default value of zero. In this case it does the maximum splits that can be done, but if we give value to maxsplit, it will split the string. ExampleLet’s look at the example below −import result=re.split(r'a', 'Dynamics Kinematics') print resultOutput['Dyn', 'mics Kinem', 'tics']ExampleConsider the following codeimport re result=re.split(r'i', 'Dynamics Kinematics', maxsplit=1) print resultOutput['Dyn', 'mics Kinematics']Here, you can notice that we have fixed the ... Read More

1K+ Views
Regex, often known as regexp, is a potent tool for finding and manipulating text strings, especially when processing text files. Regex may easily replace many hundred lines of computer code with only one line. All scripting languages, including Perl, Python, PHP, JavaScript, general-purpose programming languages like Java, and even word processors like Word, support Regex for text searching. Regex may be challenging to learn because of its complicated syntax, but it is time well spent. Special Characters Text processing becomes more challenging when special characters are included because context must be carefully considered. You must think about what you see, ... Read More

2K+ Views
Introduction The question mark makes the previous token in the regular expression optional. For example: colou?r is complementary to both colour and colour. A quantifier is what the question mark is known as. You may make multiple tokens optional by combining numerous tokens in parentheses and adding the question mark after the final set of parentheses. Like Nov(ember)? matches between Nov and Nov. Using many question marks, you may create a regular expression matching a wide range of options. Feb(ruary)? 23(rd)? Matches February 23rd, February 23, Feb 23rd and Feb 23. Curly braces can also be used to make something ... Read More