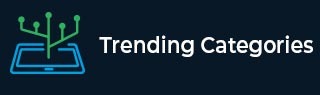
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

329 Views
As per Python documentationIf one wants more information about all matches of a pattern than the matched text, finditer() is useful as it provides match objects instead of strings. If one was a writer who wanted to find all of the adverbs and their positions in some text, he or she would use finditer() in the following manner −>>> text = "He was carefully disguised but captured quickly by police." >>> for m in re.finditer(r"\w+ly", text): ... print('%02d-%02d: %s' % (m.start(), m.end(), m.group(0))) 07-16: carefully 40-47: quickly

875 Views
When the match method is implemented, if it turns out that there are no matches, then None is returned. There are no functions in the re module that throw up an exception when the list or matches is emptyexception re.errorException raised when a string passed to one of the functions here is not a valid regular expression (for example, it might contain unmatched parentheses) or when some other error occurs during compilation or matching. It is never an error if a string contains no match for a pattern.

525 Views
Presently, when regular expressions are compiled, the result is cached so that if the same regex is compiled again, it is retrieved from the cache and no extra effort is required. This cache supports up to 100 entries. Once the 100th entry is reached, the cache is cleared and a new compile must occur.The objective of caching is to decrease the average call time of the function. The overhead associated with keeping more information in _cache and paring it instead of clearing it would increase that average call time. The _cache.clear() call will complete quickly, and even though cache is ... Read More

4K+ Views
re.match(), re.search(), and re.findall() are methods of the Python module re (Python Regular Expressions). The re.match() method The re.match() method finds match if it occurs at start of the string. For example, calling match() on the string ‘TP Tutorials Point TP’ and looking for a pattern ‘TP’ will match. Example import re result = re.match(r'TP', 'TP Tutorials Point TP') print result.group(0) Output TP The re.search() method The re.search() method is similar to re.match() but it doesn’t limit us to find matches at the beginning of the string only. Example import re result = re.search(r'Tutorials', 'TP Tutorials Point TP') ... Read More

51 Views
You will study regular expressions (RegEx) in this blog and interact with RegEx using Python's re-module (with the help of examples). A Regular Expression (RegEx) is a sequence of characters that defines a search pattern. For example, ^a...s$ A RegEx pattern is defined by the code above. Any five-letter string with an a and a s at the end forms the pattern. Python has a module named re to work with RegEx. Here's an example − import re pattern = '^a...s$' test_string = 'abyss' result = re.match(pattern, test_string) if result: print("Search successful.") else: ... Read More

5K+ Views
According to Python documentationPython does not currently have an equivalent to scanf(). Regular expressions are generally more powerful, though also more verbose, than scanf() format strings. The table below offers some more-or-less equivalent mappings between scanf() format tokens and regular expressions.scanf() TokenRegular Expression%c.%5c.{5}%d[-+]?\d+%e, %E, %f, %g[-+]?(\d+(\.\d*)?|\.\d+)([eE][-+]?\d+)?%i[-+]?(0[xX][\dA-Fa-f]+|0[0-7]*|\d+)%o[-+]?[0-7]+%s\S+%u\d+%x, %X[-+]?(0[xX])?[\dA-Fa-f]+To extract the filename and numbers from a string like/usr/sbin/sendmail - 0 errors, 4 warningsyou would use a scanf() format like%s - %d errors, %d warningsThe equivalent regular expression would be(\S+) - (\d+) errors, (\d+) warningsRead More

255 Views
The most basic regex features are nearly the same in nearly every implementation: wild character ., quantifiers *, +, and ?, anchors ^ and $, character classes inside [], and back references \1, \2, \3etc.Alternation is denoted | in Perl and PythonPerl and Python will let you modify a regular expression with (?aimsx). For example, (?i) makes an expression case-insensitive. These modifiers have the same meaning on both languages. Also, both languages let you introduce a comment in a regular expression with (?# … ).Perl and Python support positive and negative look-around with the same syntax: (?=), (?!), (?Both languages ... Read More