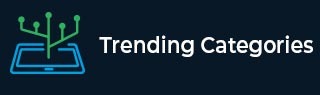
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

795 Views
The following code uses Python regex to match floating point numbersExampleimport re s = '234.6789' match = re.match(r'[+-]?(\d+(\.\d*)?|\.\d+)([eE][+-]?\d+)?',s) print match.group() s2 = '0.45' match = re.match(r'[+-]?(\d+(\.\d*)?|\.\d+)([eE][+-]?\d+)?',s2) print match.group()OutputThis gives the output234.6789 0.45

221 Views
The following code validates a number exactly equal to '2018'Exampleimport re s = '2018' match = re.match(r'\b2018\b',s) print match.group()OutputThis gives the output2018ExampleThe following code validates any five digit positive integerimport re s = '2346' match = re.match(r'(?

341 Views
The re.findall() helps to get a list of all matching patterns. It searches from start or end of the given string. If we use method findall to search for a pattern in a given string it will return all occurrences of the pattern. While searching a pattern, it is recommended to use re.findall() always, it works like re.search() and re.match() both.Exampleimport re result = re.search(r'TP', 'TP Tutorials Point TP') print result.group()OutputTP

483 Views
According to Python docs, perhaps the most important metacharacter in regular expressions is the backslash, \. As in Python string literals, the backslash can be followed by various characters to indicate various special sequences. It’s also used to escape all the metacharacters so you can still match them in patterns; for example, if you need to match a [ or \, you can precede them with a backslash to remove their special meaning: \[ or \.The following code highlights the function of backslash in Python regexExampleimport re result = re.search('\d', '\d') print result result = re.search(r'\d', '\d') print result.group()OutputThis gives ... Read More

756 Views
The re.findall() methodThe re.findall() helps to get a list of all matching patterns. It searches from start or end of the given string. If we use method findall to search for a pattern in a given string it will return all occurrences of the pattern. While searching a pattern, it is recommended to use re.findall() always, it works like re.search() and re.match() both.Exampleimport re result = re.search(r'TP', 'TP Tutorials Point TP') print result.group()OutputTPThe re.finditer() methodre.finditer(pattern, string, flags=0) Return an iterator yielding MatchObject instances over all non-overlapping matches for the RE pattern in string. The string is scanned left-to-right, and matches ... Read More

2K+ Views
URL is an acronym for Uniform Resource Locator; it is used to identify the location resource on internet. For example, the following URLs are used to identify the location of Google and Microsoft websites − https://www.google.com https://www.microsoft.com URL consists of domain name, path, port number etc. The URL can be parsed and processed by using Regular Expression. Therefore, if we want to use Regular Expression we have to use re library in Python. Example Following is the example demonstrating URL − URL: https://www.tutorialspoint.com/courses If we parse the above URL we can find the website name and protocol ... Read More

2K+ Views
Range in Regular ExpressionsRanges of characters can be indicated by giving two characters and separating them by a '-', for example [a-z] will match any lowercase ASCII letter, [0-5][0-9] will match all the two-digits numbers from 00 to 59.If - is escaped (e.g. [a\-z]) or if it’s placed as the first or last character (e.g. [a-]), it will match a literal '-'. The regex [A-Z] matches all uppercase letters from A to Z. Similarly the regex [a-c] matches the lowercase letters from a to z.The regex [0-9] matches single-digit numbers 0 to 9. [1-9][0-9] matches double-digit numbers 10 to 99. That's ... Read More

6K+ Views
From Python documentationNon-special characters match themselves. Special characters don't match themselves −\ Escape special char or start a sequence..Match any char except newline, see re.DOTALL^Match start of the string, see re.MULTILINE $ Match end of the string, see re.MULTILINE[ ]Enclose a set of matchable charsR|S Match either regex R or regex S.()Create capture group, & indicate precedenceAfter '[', enclose a set, the only special chars are −]End the set, if not the 1st char-A range, eg. a-c matches a, b or c^Negate the set only if it is the 1st char Quantifiers (append '?' for non-greedy) −{m}Exactly m repetitions {m, n}From m (default 0) ... Read More