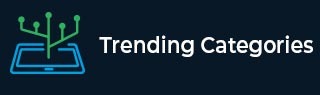
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

216 Views
Both re.match() and re.search() are methods of the Python module re.The re.match() method finds match if it occurs at start of the string. For example, calling match() on the string ‘TP Tutorials Point TP’ and looking for a pattern ‘TP’ will match.Exampleresult = re.match(r'TP', 'TP Tutorials Point TP') print result.group(0)OutputTPThe re.search() method is similar to re.match() but it doesn’t limit us to find matches at the beginning of the string only. Exampleresult = re.search(r'Tutorials', 'TP Tutorials Point TP') print result.group(0)OutputTutorialsHere you can see that, search() method is able to find a pattern from any position of the string.Read More

656 Views
Named GroupsMost modern regular expression engines support numbered capturing groups and numbered backreferences. Long regular expressions with lots of groups and backreferences can be difficult to read and understand. More over adding or removing a capturing group in the middle of the regex disturbs the numbers of all the groups that follow the added or removed group.Python's re module was the first to come up with a solution: named capturing groups and named backreferences. (?Pgroup) captures the match of group into the backreference "name". name must be an alphanumeric sequence starting with a letter. group can be any regular expression. ... Read More

1K+ Views
Non capturing groupsIf we do not want a group to capture its match, we can write this regular expression as Set(?:Value). The question mark and the colon after the opening parenthesis are the syntax that creates a non-capturing group. The regex Set(Value)? matches Set or SetValue. In the first case, the first (and only) capturing group remains empty. In the second case, the first capturing group matches Value. The question mark appearing at the end is the quantifier that makes the previous token optional. Set(?:Value) matches Setxxxxx, i.e., all those strings starting with Set but not followed by Value. Such would be ... Read More

778 Views
The following code extracts data like first_id, second_id, category from given stringsExampleimport re s = 'TS001B01.JPG' match = re.match(r'(TS\d+)([A|B])(\d+)\.JPG', s) first_id = match.group(1) category = match.group(2) second_id = match.group(3) print first_id print category print second_idOutputThis gives outputTS001 B 01

8K+ Views
The following code demonstrates the use of variables in Python regex.The variable cannot contain any special or meta characters or regular expression. We just use string concatenation to create a string.Example import re s = 'I love books' var_name = 'love' result = re.search('(.+)'+var_name+'(.+)',s) print result var_name = 'hate' s2 = 'I hate books' result = re.search('(.+)'+var_name+'(.+)',s2) print resultOutputThis gives the output

1K+ Views
The re.search() method is similar to re.match() but it doesn’t limit us to find matches at the beginning of the string only. Exampleimport re result = re.search(r'Tutorials', 'TP Tutorials Point TP') print result.group()OutputTutorialsHere you can see that, search() method is able to find a pattern from any position of the string.The re.findall() helps to get a list of all matching patterns. It searches from start or end of the given string. If we use method findall to search for a pattern in a given string it will return all occurrences of the pattern. While searching a pattern, it is recommended to ... Read More