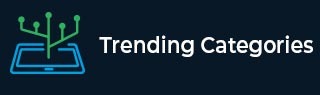
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

1K+ Views
Character class operations are a way to match certain types of characters in a string using regular expressions in Python. In regular expressions, a "character class" is a set of characters that you want to match. You can use square brackets `[]` to create a character class. For example, if you want to match any vowel, you can use the character class `[aeiou]`. This will match any single character that is either 'a', 'e', 'i', 'o', or 'u'. Example This code will search for all the vowels in the `text` string using the regular expression `[aeiou]`, and it will return ... Read More

307 Views
Nested Character Class SubtractionSince we can use the full character class syntax within the subtracted character class, we can subtract a class from the class being subtracted. [0-9-[0-7-[0-3]]] first subtracts 0-3 from 0-7, yielding [0-9-[4-7]], or [0-38-9], which matches any character in the string 012389.The class subtraction is always the last element in the character class. [0-9-[4-7]a-d] is not a valid regular expression. It should be rewritten as [0-9a-d-[4-7]]. The subtraction works on the whole class. While we can use nested character class subtraction, we cannot subtract two classes sequentially. To subtract ASCII characters and Arabic characters from a class with ... Read More

4K+ Views
The following code uses a regular expression '(a|b)' to match a or b in the given Python string.We are also using the flag re.I to ignore case of a or b while matching. Example import re s = 'Bank of Baroda' print(re.findall(r'(a|b)',s, re.I))OutputThis gives the output['B', 'a', 'B', 'a', 'a']

814 Views
The following code matches anything except space and new line in the given string using regex.Exampleimport re print re.match(r'^[^ ]*$', """IfindTutorialspointuseful""") print re.match(r'^[^ ]*$', """I find Tutorialspointuseful""") print re.match(r'^[^ ]*$', """Ifind Tutorialspointuseful""")OutputThis gives the output None None

419 Views
The following code strips the spaces/tabs/newlines from given string using Python regexWe use '\S' in regex which stands for all non-whitespace charactersExampleimport re print re.findall(r"[\S]","""I find Tutorialspoint useful""")OutputThis gives the output['I', 'f', 'i', 'n', 'd', 'T', 'u', 't', 'o', 'r', 'i', 'a', 'l', 's', 'p', 'o', 'i', 'n', 't', 'u', 's', 'e', 'f', 'u', 'l']

663 Views
Recursion Recursion is useful in dividing and solving problems. Each recursive call itself spins off other recursive calls. At the centre of a recursive function are two types of cases: base cases, which tell the recursion when to terminate, and recursive cases that call the function they are in. A simple problem that naturally uses recursive solutions is calculating factorials. The recursive factorial algorithm has two cases: the base case when n = 0, and the recursive case when n>0 . BacktrackingBacktracking is a general algorithm for finding solutions to some computational problem, that incrementally builds choices to the solutions, and rejects ... Read More