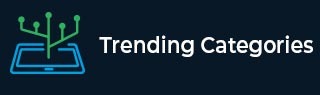
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

3K+ Views
It is very easy to do date and time maths in Python using timedelta objects. Whenever you want to add or subtract to a date/time, use a datetime.datetime(), then add or subtract datetime.timedelta() instances. A timedelta object represents a duration, the difference between two dates or times. The timedelta constructor has the following function signature −datetime.timedelta([days[, seconds[, microseconds[, milliseconds[, minutes[, hours[, weeks]]]]]]])Note: All arguments are optional and default to 0. Arguments may be ints, longs, or floats, and may be positive or negative. You can read more about it here − https://docs.python.org/2/library/datetime.html#timedelta-objectsExampleAn example of using the timedelta objects and dates ... Read More

2K+ Views
In this article, we will show you how to write a function to get the time spent in each function using python. Now we see 4 methods to accomplish this task− Now we see 2 methods to accomplish this task− Using time.clock() function Using time.time() function Using time.process_time() function Using datetime.now() function Method 1: Using time.clock() Python's Time module provides a variety of time−related functions. The method time.clock() returns the current processor time as a floating point number expressed in seconds on Unix. The precision depends on that of the C function of the same name, but in ... Read More

6K+ Views
In this article, we will discuss various ways to convert time in seconds to H M S format in python. H M S format means Hours: Minutes: Seconds. Using arithmetic operations (Naïve method) In this method, we use mathematic calculations to convert time in seconds to h m s format. Here we take the input from the user in seconds and convert it into the required format. Example In this example, we convert a time in seconds to h m s format. seconds = int(input("Enter the number of seconds:")) seconds = seconds % (24 * 3600) hour = seconds // ... Read More

656 Views
You can get the current time in milliseconds in Python using the time module. You can get the time in seconds using time.time function(as a floating point value). To convert it to milliseconds, you need to multiply it with 1000 and round it off. exampleimport time milliseconds = int(round(time.time() * 1000)) print(milliseconds)OutputThis will give the output −1514825676008If you want to convert a datetime object to milliseconds timestamp, you can use the timestamp function then apply the same math as above to get the milliseconds time. exampleimport time from datetime import datetime dt = datetime(2018, 1, 1) milliseconds = int(round(dt.timestamp() * 1000)) print(milliseconds)OutputThis ... Read More

134 Views
An timestamp is an offset value between a point in time line and the epoch, it's nothing to do with timezone. When it's converted to a human readable string like '%Y-%m-%d %H:%M:%S' that doesn't include any timezone information, python assumes that you want to use local timezone setting.datetime.timestamp() on a naive datetime object calls mktime() internally i.e., the input is interpreted as the local time. Local time definitions may differ between systems.C mktime() may return a wrong result if the local timezone had different utc offset in the past and a historical timezone database is not used.On Unix, when we ... Read More

52K+ Views
The ISO 8601 standard defines an internationally recognized format for representing dates and times. ISO 8601 is a date and time format which helps to remove different forms of the day, date, and time conventions across the world. To tackle this uncertainty of various formats ISO sets a format to represent dates "YYYY-MM-DD". For example, May 31, 2022, is represented as 2022-05-31. In this article, we will discuss how to get an ISO 8601 date in string format in python. Using the .isoformat() method The .isoformat() method returns a string of date and time values of a python datetime.date object ... Read More

4K+ Views
In this article, we will discuss the various way to convert the python datetime string to milliseconds in python. Using time.time() method The time module in python provides various methods and functions related to time. Here we use the time.time() method to get the current CPU time in seconds. The time is calculated since the epoch which returns a floating-point number expressed in seconds. This value is multiplied by 1000 and rounded off with the round() function. NOTE: Epoch is the starting point of time and is platform-dependent. The epoch is January 1, 1970, 00:00:00 (UTC) on Windows and most ... Read More

620 Views
The datetime in Javascript and in Python have 2 major differences. First one being the meaning of the month argument.The month in Javascript is expected between 0-11 while in Python it is expected to be between 1-12. So the following tuple actually represents 2 different dates in Python and in Javascript −(2017, 11, 1) Python: 1st November 2017 Javascript: 1sd December 2017The second difference is that they have different default timezones, with Python defaulting to UTC while JavaScript defaults to the user's "local" timezone. You can use Date.UTC(), which returns the timestamp, for the equivalent in JavaScript. For example,var utc = Date.UTC(2013, 7, 10);

630 Views
If not explicitly stated, the date time functions/modules in Python assume everything in Local time zone.time.mktime() assumes that the passed tuple is in local time, calendar.timegm() assumes it's in GMT/UTC.Depending on the interpretation the tuple represents a different time, so the functions return different values (seconds since the epoch are UTC based).The difference between the values should be equal to the time zone offset of your local time zone.exampleimport calendar import time from datetime import datetime dt = datetime(2017, 12, 31) print(time.mktime(dt.timetuple())) print(calendar.timegm(dt.timetuple()))OutputThis will give the output −1514658600.0 1514678400Read More

33K+ Views
You can use the datetime module to convert a datetime to a UTC timestamp in Python. If you already have the datetime object in UTC, you can the timestamp() to get a UTC timestamp. This function returns the time since epoch for that datetime object. If you have the datetime object in local timezone, first replace the timezone info and then fetch the time. examplefrom datetime import timezone dt = datetime(2015, 10, 19) timestamp = dt.replace(tzinfo=timezone.utc).timestamp() print(timestamp)OutputThis will give the output −1445212800.0If you're on Python 2, then you can use the total_seconds() function to get the total seconds since epoch. And ... Read More