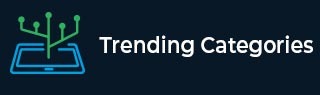
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

7K+ Views
In this article, we will explain nested/inner functions in Python and how they work with examples. Nested (or inner) functions are functions defined within other functions that allow us to directly access the variables and names defined in the enclosing function. Nested functions can be used to create closures and decorators, among other things. Defining an inner/nested Function Simply use the def keyword to initialize another function within a function to define a nested function. The following program is to demonstrate the inner function in Python − Example # creating an outer function def outerFunc(sample_text): sample_text ... Read More

1K+ Views
The function datetime.now() takes tzinfo as keyword argument but datetime.today() does not take any keyword arguments. Quoting the docs −datetime.now() returns the current local date and time. If optional argument tz is None or not specified, this is like today(), but, if possible, supplies more precision than can be gotten from going through a time.time() timestamp (for example, this may be possible on platforms supplying the C gettimeofday() function).

43K+ Views
In this article, we will show you how to convert date and time with different timezones in Python. Using astimezone() function Using datetime.now() function The easiest way in Python date and time to handle timezones is to use the pytz module. This library allows accurate and cross−platform timezone calculations. pytz brings the Olson tz database into Python. It also solves the issue of ambiguous times at the end of daylight saving time, which you can read more about in the Python Library Reference (datetime.tzinfo) Before you use it you'll need to install it using − pip install pytz ... Read More

4K+ Views
In this article, we will show you how to compare time to different timezones in Python using the below methods. Comparing the given Timezone with the local TimeZone Comparing the Current Datetime of Two Timezones Comparing Two Times with different Timezone Method 1: Comparing the given Timezone with the local TimeZone Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task – Use the import keyword, to import the datetime, pytz modules. Use the timezone() function (gets the time zone of a specific location) of the pytz module, to get the timezone ... Read More

30K+ Views
In this article, we will show you how to do date validation in Python. Now we see 2 methods to accomplish this task− Using datetime.strptime() function Using dateutil.parser.parse() function Method 1: Using datetime.strptime() function Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Use the import keyword, to import the datetime (To work with dates and times) module. Enter the date as a string and create a variable to store it. Enter the date format as a string and create another variable to store it. Use the try−except blocks for handling the ... Read More

836 Views
Python date implementations support all the comparision operators. So, if you are using the datetime module to create and handle date objects, you can simply use the , =, etc. operators on the dates. This makes it very easy to compare and check dates for validations, etc.Examplefrom datetime import datetime from datetime import timedelta today = datetime.today() yesterday = today - timedelta(days=1) print(today < yesterday) print(today > yesterday) print(today == yesterday)OutputThis will give the output −False True False

14K+ Views
To insert a date in a MySQL database, you need to have a column of Type date or datetime in your table. Once you have that, you'll need to convert your date in a string format before inserting it to your database. To do this, you can use the datetime module's strftime() formatting function. For example from datetime import datetime now = datetime.now() id = 1 formatted_date = now.strftime('%Y-%m-%d %H:%M:%S') # Assuming you have a cursor named cursor you want to execute this query on: cursor.execute('insert into table(id, date_created) values(%s, %s)', (id, formatted_date))Running this will try to insert the ... Read More

4K+ Views
To insert a date in a MySQL database, you need to have a column of Type date or datetime in your table. Once you have that, you'll need to convert your date in a string format before inserting it to your database. To do this, you can use the datetime module's strftime formatting function.Example from datetime import datetime now = datetime.now() id = 1 formatted_date = now.strftime('%Y-%m-%d %H:%M:%S') # Assuming you have a cursor named cursor you want to execute this query on: cursor.execute('insert into table(id, date_created) values(%s, %s)', (id, formatted_date))Running this will try to insert the tuple (id, date) ... Read More