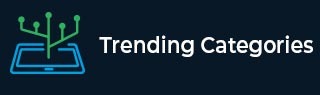
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

1K+ Views
__init__ "__init__" is a reserved method in python classes. It is known as a constructor in OOP concepts. This method called when an object is created from the class and it allows the class to initialize the attributes of a class.How can we use "__init__ " ?Let's consider that we are creating a class named Car. Car can have attributes like "color", "model", "speed" etc. and methods like "start", "accelarate", "change_ gear" and so on.Exampleclass Car(object): def __init__(self, model, color, speed): self.color = color self.speed = ... Read More

3K+ Views
In this article we are going to discuss how to create subclass from a super class in Python. Before proceeding further let us understand what is a class and a super class. A class is a user-defined template or prototype from which objects are made. Classes offer a way to bundle together functionality and data. The ability to create new instances of an object type is made possible by the production of a new class. Each instance of a class may have attributes connected to it to preserve its state. Class instances may also contain methods for changing their state ... Read More

378 Views
Object-oriented programming creates reusable patterns of code to prevent code redundancy in projects. One way that recyclable code is created is through inheritance, when one subclass leverages code from another base class.Inheritance is when a class uses code written within another class.Classes called child classes or subclasses inherit methods and variables from parent classes or base classes.Because the Child subclass is inheriting from the Parent base class, the Child class can reuse the code of Parent, allowing the programmer to use fewer lines of code and decrease redundancy.Derived classes are declared much like their parent class; however, a list of ... Read More

248 Views
The code below shows the if the attribute 'foo' was defined or derived in the classes A and B.Exampleclass A: foo = 1 class B(A): pass print A.__dict__ #We see that the attribute foo is there in __dict__ of class A. So foo is defined in class A. print hasattr(A, 'foo') #We see that class A has the attribute but it is defined. print B.__dict__ #We see that the attribute foo is not there in __dict__ of class B. So foo is not defined in class B print hasattr(B, 'foo') #We see that class B has ... Read More

263 Views
We have the classes A and B defined as follows −class A(object): pass class B(A): passExampleA can be proved to be a super class of B in two ways as followsclass A(object):pass class B(A):pass print issubclass(B, A) # Here we use the issubclass() method to check if B is subclass of A print B.__bases__ # Here we check the base classes or super classes of BOutputThis gives the outputTrue (,)

256 Views
We have the classes A and B defined as follows −class A(object): pass class B(A): passB can be proved to be a sub class of A in two ways as followsclass A(object):pass class B(A):pass print issubclass(B, A) # Here we use the issubclass() method to check if B is subclass of A print B.__bases__ # Here we check the base classes or super classes of BThis gives the outputTrue (,)

121 Views
We can derive a class from multiple parent classes as follows −class A: # define your class A ..... class B: # define your class B ..... class C(A, B): # subclass of A and B .....We can use isinstance() function to check the relationships of two classes and instances.Theisinstance(obj, Class) boolean function returns true if obj is an instance of class Class or is an instance of a subclass of Class

114 Views
We can derive a class from multiple parent classes as follows −class A: # define your class A ..... class B: # define your class B ..... class C(A, B): # subclass of A and B .....We can use issubclass() function to check the relationships of two classes and instances.For example, theissubclass(sub, sup) boolean function returns true if the given subclass sub is indeed a subclass of the superclass sup.

158 Views
Inheritance in classesInstead of defining a class afresh, we can create a class by deriving it from a preexisting class by listing the parent class in parentheses after the new class name.The child class inherits the attributes of its parent class, and we can use those attributes as if they were defined in the child class. A child class can also override data members and methods from the parent.SyntaxDerived classes are declared much like their parent class; however, a list of base classes to inherit from is given after the class name −class SubClassName (ParentClass1[, ParentClass2, ...]): 'Optional class ... Read More

5K+ Views
Python deletes unwanted objects (built-in types or class instances) automatically to free the memory space. The process by which Python periodically frees and reclaims blocks of memory that no longer are in use is called Garbage Collection.Python's garbage collector runs during program execution and is triggered when an object's reference count reaches zero. An object's reference count changes as the number of aliases that point to it changes.An object's reference count increases when it is assigned a new name or placed in a container (list, tuple, or dictionary). The object's reference count decreases when it's deleted with del, its reference is ... Read More