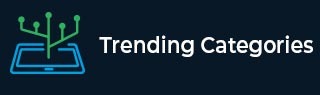
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

149 Views
In Python 2.x there are two styles of classes depending on the presence or absence of a built-in type as a base-class −‘Old style’ or "Classic" style classes: they have no built-in type as a base class −>>> class OldFoo: # no base class ... pass >>> OldFoo.__bases__ ()"New" style classes: they have a built-in type as a base class meaning that, directly or indirectly, they have object as a base class −>>> class NewFoo(object): # directly inherit from object ... pass >>> NewFoo.__bases__ (, )In Python 3.x however, only ... Read More

3K+ Views
Metaprogramming in python is defined as the ability of a program to influence itself. It is achieved by using metaclass in python. Metaclasses in Python Metaclasses are an OOP concept present in all python code by default. Python provides the functionality to create custom metaclasses by using the keyword type. Type is a metaclass whose instances are classes. Any class created in python is an instance of type metaclass. The type() function can create classes dynamically as calling type() creates a new instance of type metaclass. Syntax Syntax to create a class using type() is given below − class ... Read More

4K+ Views
Python variable name may begin with a single underscore. It functions as a convention to indicate that the variable name is now a private variable. It should be viewed as an implementation detail that could change at any time. Programmers can assume that variables marked with a single underscore are reserved for internal usage. Single underscores are advised for semi-private variables, and double underscores are advised for fully private variables. To paraphrase PEP-8; single leading underscore: a poor signal of "internal use." For instance, from M import * excludes objects with names that begin with an underscore. Syntax The syntax ... Read More

5K+ Views
When a double underscore is added as prefix to python variables the name mangling process is applied to a specific identifier(__var) In order to avoid naming conflicts with the subclasses, name mangling includes rewriting the attribute name. Example Following is the program to explain the double underscore in Python − class Python: def __init__(self): self.car = 5 self._buzz = 9 self.__fee = 2 d = Python() print(dir(d)) Output Following is an output of the above code − ['_Python__fee', ... Read More

3K+ Views
Data hidingIn Python, we use double underscore before the attributes name to make them inaccessible/private or to hide them.The following code shows how the variable __hiddenVar is hidden.Exampleclass MyClass: __hiddenVar = 0 def add(self, increment): self.__hiddenVar += increment print (self.__hiddenVar) myObject = MyClass() myObject.add(3) myObject.add (8) print (myObject.__hiddenVar)Output 3 Traceback (most recent call last): 11 File "C:/Users/TutorialsPoint1/~_1.py", line 12, in print (myObject.__hiddenVar) AttributeError: MyClass instance has no attribute '__hiddenVar'In the above program, we tried to access hidden variable outside the class using object and it threw an ... Read More

312 Views
The cmp() functionThe cmp(x, y) function compares the values of two arguments x and y −cmp(x, y)The return value is −A negative number if x is less than y.Zero if x is equal to y.A positive number if x is greater than y.The built-in cmp() function will typically return only the values -1, 0, or 1. However, there are other places that expect functions with the same calling sequence, and those functions may return other values. It is best to observe only the sign of the result.>>> cmp(2, 8) -1 >>> cmp(6, 6) 0 >>> cmp(4, 1) 1 >>> cmp('stackexchange', ... Read More

346 Views
The __str__ method__str__ is a special method, like __init__, that returns a 'informal' string representation of an object. It is useful in debugging.Consider the following code that uses the __str__ methodclass Time: def __str__(self): return '%.2d:%.2d:%.2d' % (self.hour, self.minute, self.second)When we print an object, Python invokes the str method −>>> time = Time(7, 36) >>> print time 07:36:00

18K+ Views
In this tutorial, we will discuss how we can check for a number whether it is a decimal number or a whole number using the JavaScript. In JavaScript, we can use in−built methods as well as the user defined methods to check for a number if it has a decimal place or it is a whole number. We will discuss about all those methods in details. Let us see what are those methods that we can use to accomplish this task. Following methods are very useful to check for a number if it is decimal or whole number − ... Read More

542 Views
The official Python documentation says __repr__() is used to compute the “official” string representation of an object. The repr() built-in function uses __repr__() to display the object. __repr__() returns a printable representation of the object, one of the ways possible to create this object. __repr__() is more useful for developers while __str__() is for end users.ExampleThe following code shows how __repr__() is used.class Point: def __init__(self, x, y): self.x, self.y = x, y def __repr__(self): return 'Point(x=%s, y=%s)' % (self.x, self.y) p = Point(3, 4) print pOutputThis gives ... Read More

514 Views
The __del__() method is a known as a destructor method. It is called when an object is garbage collected which happens after all references to the object have been deleted.In a simple case this could be right after you delete a variable like del x or, if x is a local variable, after the function ends. In particular, unless there are circular references, CPython which is the standard Python implementation will garbage collect immediately.The only property of Python garbage collection is that it happens after all references have been deleted, so this might not necessarily happen right after and even ... Read More