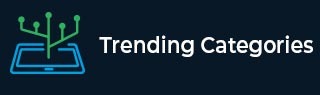
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

159 Views
The following code prints the list of functions of the given class as followsExampleclass foo: def __init__(self): self.x = x def bar(self): pass def baz(self): pass print (type(foo)) import inspect print(inspect.getmembers(foo, predicate=inspect.ismethod))OutputThe output is [('__init__', ), ('bar', ), ('baz', )]

10K+ Views
Given a string as user input to a Python function, I'd like to get a class object out of it if there's a class with that name in the currently defined namespace.Exampleclass Foobar: pass print eval("Foobar") print type(Foobar)Output __main__.Foobar Another way to convert a string to class object is as followsExampleimport sys class Foobar: pass def str_to_class(str): return getattr(sys.modules[__name__], str) print str_to_class("Foobar") print type(Foobar)Output__main__.Foobar

897 Views
We can use python-jsonschema-objects which is built on top of jsonschema.The python-jsonschema-objects provide an automatic class-based binding to JSON schemas for use in Python.We have a sample json schema as followsschema = '''{ "title": "Example Schema", "type": "object", "properties": { "firstName": { "type": "string" }, "lastName": { "type": "string" }, "age": { "description": "Age in years", ... Read More

10K+ Views
A global variable is a variable with global scope, meaning that it is visible and accessible throughout the program, unless shadowed. The set of all global variables is known as the global environment or global scope of the program.We declare a variable global by using the keyword global before a variable. All variables have the scope of the block, where they are declared and defined in. They can only be used after the point of their declaration.ExampleExample of global variable declarationdef f(): global s print(s) ... Read More

351 Views
As per Python documentation ‘super’ can help in extending multiple python classes in inheritance. It returns a proxy object that delegates method calls to a parent or sibling class of type. This is useful for accessing inherited methods that have been overridden in a class. The search order is same as that used by getattr() except that the type itself is skipped.In other words, a call to super returns a fake object which delegates attribute lookups to classes above you in the inheritance chain. Points to note:This does not work with old-style classes.You need to pass your own class and ... Read More

303 Views
A class attribute exists until the last reference goes away. A global variable also exists until the last reference goes away. Neither of these are guaranteed to last the entire duration of the program.Also, a class defined at module scope is a global variable. So the class (and, by implication, the attribute) have the same lifetime as a global variable in that case. If no instances of the class are currently live, the class and its class attributes might be garbage-collected if their reference counts become zero.

259 Views
Object serialization and deserialization are a routine aspect of any non-trivial Python program. Saving to a file, reading a configuration file, responding to an HTTP request, all involve object serialization and deserialization. Serialization and deserialization involve various schemes, formats and protocols to stream Python objects and to get them back later intact. Serialization schemes, formats or protocols you choose determine how fast your program runs and how secure it is.Lets use a dictionary of python objects to serialize. Class is also a Python object. We use a Python module named pickle and its method pickle.dumps(object).foo = dict(int_list=[3, 4, 5], text='Hello World', ... Read More

3K+ Views
Python is not class-based exclusively - the basic unit of code decomposition in Python is the module. A module is a distinct thing that may have one or two dozen closely-related classes. Modules may as well contain functions along with classes. In Python there is rule of one module=one file.In Python if you restrict yourself to one class per file (which in Python is not prohibited) you may end up with large number of small files – not easy to keep track.So depending on the scenario and convenience one can have one or more classes per file in Python.Read More

762 Views
I would recommend generateDS for converting a XSD file to a Python class . In my opinion, it is a good tool for the said purpose.It (generatS) generates the Python class with all methods (setters and getters, export to XML, import from XML). It does a good job and works very well !.

335 Views
A class can be derived from more than one base classes in Python. This is called multiple inheritance.In multiple inheritance, the features of all the base classes are inherited into the derived class. The syntax for multiple inheritance is similar to single inheritance.class Super1: pass class Super2: pass class MultiDerived(Super1, Super2): passIn the multiple inheritance scenario, any specified attribute is searched first in the current class. If not found, the search continues into parent classes in depth-first, left-right fashion without searching same class twice.So, in the above example of MultiDerived class the search order is ... Read More