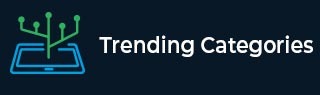
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

364 Views
The % symbol is defined in Python as modulo operator. It can also be called remainder operator. It returns remainder of division of two numeric operands (except complex numbers).>>> a=10 >>> b=3 >>> a%b 1 >>> a=12.25 >>> b=4 >>> a%b 0.25 >>> a=-10 >>> b=6 >>> a%b 2 >>> a=1.55 >>> b=0.05 >>> a%b 0.04999999999999996

5K+ Views
The bitwise operator ~ (pronounced as tilde) is a complement operator. It takes one bit operand and returns its complement. If the operand is 1, it returns 0, and if it is 0, it returns 1For example if a=60 (0011 1100 in binary) its complement is -61 (-0011 1101) stored in 2's complement>>> a=60 >>> bin(a) '0b111100' >>> b=~a >>> a 60 >>> >>> b -61 >>> bin(b) '-0b111101

29K+ Views
In this tutorial, we will learn how to subtract days from a date in JavaScript. To subtract days from a JavaScript Date object, use the setDate() method. Under that, get the current days and subtract days. JavaScript date setDate() method sets the day of the month for a specified date according to local time. Following are the methods we can use to subtract days from a date in JavaScript − Using the setTime() and getTime() methods Using the setDate() and getDate() methods ... Read More

156 Views
In C/C++ and Java etc, ++ and -- operators are defined as increment and decrement operators. In Python they are not defined as operators.In Python objects are stored in memory. Variables are just the labels. Numeric objects are immutable. Hence they can't be incremented or decremented.However, prefix ++ or -- doesn't give error but doesn't perform either.>>> a=5 >>> b=6 >>> ++a 5 >>> --b 6Postfix ++ or -- produce errors>>> a=5 >>> b=6 >>> a++ SyntaxError: invalid syntax >>> b-- SyntaxError: invalid syntax

329 Views
In Python, ^ is called EXOR operator. It is a bitwise operator which takes bits as operands. It returns 1 if one operand is 1 and other is 0.Assuming a=60 (00111100 in binary) and b=13 (00001101 in binary) bitwise XOR of a and b returns 49 (00110001 in binary)>>> a=60 >>> bin(a) '0b111100' >>> b=a^2 >>> bin(b) '0b111110' >>> a=60 >>> bin(a) '0b111100' >>> b=13 >>> bin(b) '0b1101' >>> c=a^b >>> bin(c) '0b110001'

5K+ Views
In Python, 'not in' membership operator evaluates to true if it does not finds a variable in the specified sequence and false otherwise. For example >>> a = 10 >>> b = 4 >>> l1 = [1,2,3,4,5] >>> a not in l1 True >>> b not in l1 False Since 'a' doesn't belong to l1, a not in b returns True. However, b can be found in l1, hence b not in l1 returns False

275 Views
The keys() method of Python dictionary class returns a view object consisting of keys used in the dictionary.>>> d1 = {'name': 'Ravi', 'age': 21, 'marks': 60, 'course': 'Computer Engg'} >>>d1.keys() dict_keys(['name', 'age', 'marks', 'course'])It can be stored as a list object. If new key-value pair is added, the view object is automatically updated.>>> l1=d1.keys() >>> l1 dict_keys(['name', 'age', 'marks', 'course']) >>>d1.update({"college":"IITB"}) >>> l1 dict_keys(['name', 'age', 'marks', 'course', 'college'])

291 Views
The update method is one of the methods of dictionary data structure. It is used to update the values in the already created dictionary which means it adds a new key value pair to the dictionary. The updated key and value will be updated at the last. The dictionary is represented by curly braces{}.The dictionary contains key and value pairs, combinedly called as items which can accept any data type of elements as values. It is mutable which means once the dictionary is created we can apply the changes. It has key, value pairs separated by colon and the key ... Read More