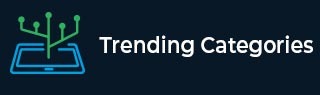
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

1K+ Views
In python there are two number data types: integers and floats. In general integers do not have any decimal points and base value is 10 (i.e., Decimal). Whereas floats have decimal points. Python provides some built−in methods to convert floats to integers. In this article we will discuss some of them. Using the int() function The int() function converts the floating point numbers to integers, by removing the decimals and remains only the integer part. Also the int() function does not round the float values like 49.8 up to 50. Example In the example the data after the decimal ... Read More

21K+ Views
To convert an integer to a character in Python, we can use the chr() method. The chr() is a Python built−in method that returns a character from an integer. The method takes an integer value and returns a unicode character corresponding to that integer. Syntax char(number) Parameter The method takes a single integer between the range of 0 to 1, 114, 111. Return Value A unicode character of the corresponding integer argument. And it will raies a ValueError if we pass an out of range value (i, e. range(0x110000)). Also it will raise TypeError − for a non−integer argument. ... Read More

360 Views
volatile means two things − The value of the variable may change without any code of yours changing it. Therefore whenever the compiler reads the value of the variable, it may not assume that it is the same as the last time it was read, or that it is the same as the last value stored, but it must be read again. The act of storing a value to a volatile variable is a "side effect" which can be observed from the outside, so the compiler is not allowed to remove the act of storing a value; for example, ... Read More

241 Views
A modifier is used to alter the meaning of the base type so that it works in accordance with your needs. For example, time cannot be negative and it makes sense to make it unsigned. C++ allows the char, int, and double data types to have modifiers preceding them. The data type modifiers are listed here −signedunsignedlongshortThe modifiers signed, unsigned, long, and short can be applied to integer base types. In addition, signed and unsigned can be applied to char, and long can be applied to double.The modifiers signed and unsigned can also be used as a prefix too long ... Read More

4K+ Views
Assuming that the list is collection of strings, the first character of each string is obtained as follows −>>> L1=['aaa','bbb','ccc'] >>> for string in L1: print (string[0]) a b cIf list is a collection of list objects. First element of each list is obtained as follows −>>> L1=[[1,2,3],[4,5,6],[7,8,9]] >>> for list in L1: print (list[0]) 1 4 7

2K+ Views
When you first declare a variable in a statically typed language such as C++ you must declare what that variable is going to hold.int number = 42;In that example, the "int" is a type specifier stating that the variable "number" can only hold integer numbers. In dynamically typed languages such as ruby or javascript, you can simply declare the variable.var number = 42;There are a lot of built-in type specifiers like double, char, float, etc in C++. You can also create your own specifiers by creating class and structs.

2K+ Views
A type qualifier is a keyword that is applied to a type, resulting in a qualified type. For example, const int is a qualified type representing a constant integer, while int is the corresponding unqualified type, simply an integer. Type qualifiers are a way of expressing additional information about a value through the type system and ensuring correctness in the use of the data. As of 2014 and C11, there are four type qualifiers in standard C: const (C89), volatile (C89), restrict (C99) and _Atomic (C11). The first two of these, const and volatile, are also present in C++ and ... Read More

649 Views
A character literal is a type of literal in programming for the representation of a single character's value within the source code of a computer program.In C++, A character literal is composed of a constant character. It is represented by the character surrounded by single quotation marks. There are two kinds of character literals −Narrow-character literals of type char, for example 'a'Wide-character literals of type wchar_t, for example L'a'The character used for a character literal may be any graphic character, except for reserved characters such as newline (''), backslash ('\'), single quotation mark ('), and double quotation mark ("). Reserved ... Read More