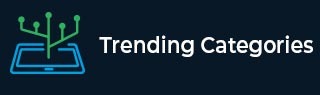
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

3K+ Views
The extern storage class specifier lets you declare objects that several source files can use. An extern declaration makes the described variable usable by the succeeding part of the current source file. This declaration does not replace the definition. The declaration is used to describe the variable that is externally defined.An extern declaration can appear outside a function or at the beginning of a block. If the declaration describes a function or appears outside of a function and describes an object with external linkage, the keyword extern is optional.If a declaration for an identifier already exists at file scope, any ... Read More

434 Views
The static storage class instructs the compiler to keep a local variable in existence during the lifetime of the program instead of creating and destroying it each time it comes into and goes out of scope. Therefore, making local variables static allows them to maintain their values between function calls.The static modifier may also be applied to global variables. When this is done, it causes that variable's scope to be restricted to the file in which it is declared.In C++, when static is used on a class data member, it causes only one copy of that member to be shared ... Read More

287 Views
In C, the register storage class specifier indicates to the compiler that the object should be stored in a machine register. The register storage class specifier is typically specified for heavily used variables, such as a loop control variable, in the hopes of enhancing performance by minimizing access time. However, the compiler is not required to honor this request. Because of the limited size and number of registers available on most systems, few variables can actually be put in registers.In C++ it is simply an unused reserved keyword, but it's reasonable to assume that it was kept for syntactical compatibility ... Read More

176 Views
In C, The auto storage class specifier lets you explicitly declare a variable with automatic storage. The auto storage class is the default for variables declared inside a block. A variable x that has automatic storage is deleted when the block in which x was declared exits.You can only apply the auto storage class specifier to names of variables declared in a block or to names of function parameters. However, these names by default have automatic storage. Therefore the storage class specifier auto is usually redundant in a data declaration.It was initially carried over to C++ for syntactical compatibility only, ... Read More

7K+ Views
Auto was a keyword that C++ "inherited" from C that had been there nearly forever, but virtually never used. All this changed with the introduction of auto to do type deduction from the context in C++11. Before C++ 11, each data type needs to be explicitly declared at compile time, limiting the values of an expression at runtime but after a new version of C++, many keywords are included which allows a programmer to leave the type deduction to the compiler itself.With type inference capabilities, we can spend less time having to write out things compiler already knows. As all ... Read More

2K+ Views
All number types in C++ can either have a sign or not. For example, you can declare an int to only represent positive integers. Unless otherwise specified, all integer data types are signed data types, i.e. they have values which can be positive or negative. The unsigned keyword can be used to declare variables without signs.Example#include using namespace std; int main() { unsigned int i = -1; int x = i; cout

2K+ Views
volatile means two things −- The value of the variable may change without any code of yours changing it. Therefore whenever the compiler reads the value of the variable, it may not assume that it is the same as the last time it was read, or that it is the same as the last value stored, but it must be read again.- The act of storing a value to a volatile variable is a "side effect" which can be observed from the outside, so the compiler is not allowed to remove the act of storing a value; for example, if ... Read More

1K+ Views
There's no such keyword in C++. List of C++ keywords can be found in section 2.11/1 of C++ language standard. restrict is a keyword in the C99 version of C language and not in C++.In C, A restrict-qualified pointer (or reference) is basically a promise to the compiler that for the scope of the pointer, the target of the pointer will only be accessed through that pointer (and pointers copied from it).C++ compilers also support this definition for optimization purposes, but it is not a part of the official language specification.

2K+ Views
We use the const qualifier to declare a variable as constant. That means that we cannot change the value once the variable has been initialized. Using const has a very big benefit. For example, if you have a constant value of the value of PI, you wouldn't like any part of the program to modify that value. So you should declare that as a const.Objects declared with const-qualified types may be placed in read-only memory by the compiler, and if the address of a const object is never taken in a program, it may not be stored at all. For ... Read More

183 Views
There's no such keyword in C++. List of C++ keywords can be found in section 2.11/1 of C++ language standard. restrict is a keyword in the C99 version of C language and not in C++.In C, A restrict-qualified pointer (or reference) is basically a promise to the compiler that for the scope of the pointer, the target of the pointer will only be accessed through that pointer (and pointers copied from it).C++ compilers also support this definition for optimization purposes, but it is not a part of the official language specification.