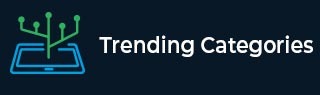
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

251 Views
There is no such operator in C++. Sometimes, we need to create wrapper types. For example, types like unique_ptr, shared_ptr, optional and similar. Usually, these types have an accessor member function called .get but they also provide the operator→ to support direct access to the contained value similarly to what ordinary pointers do.The problem is that sometimes we have a few of these types nested into each other. This means that we need to call .get multiple times or to have a lot of dereference operators until we reach the value.Something like this −wrapper wp; wp.get().get().length(); wp.get()->length();This can be a ... Read More

15K+ Views
Operator precedence determines the grouping of terms in an expression. The associativity of an operator is a property that determines how operators of the same precedence are grouped in the absence of parentheses. This affects how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has higher precedence than the addition operator:For example x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.Here, operators with the highest precedence appear ... Read More

3K+ Views
C++ provides two pointer operators, which are Address of Operator (&) and Indirection Operator (*). A pointer is a variable that contains the address of another variable or you can say that a variable that contains the address of another variable is said to "point to" the other variable. A variable can be any data type including an object, structure or again pointer itself.The indirection Operator (*), and it is the complement of &. It is a unary operator that returns the value of the variable located at the address specified by its operand. For example, Example#include using namespace ... Read More

2K+ Views
C++ provides two pointer operators, which are Address of Operator (&) and Indirection Operator (*). A pointer is a variable that contains the address of another variable or you can say that a variable that contains the address of another variable is said to "point to" the other variable. A variable can be any data type including an object, structure or again pointer itself.The address of Operator (&), and it is the complement of *. It is a unary operator that returns the address of the variable(r-value) specified by its operand. For example, Example#include using namespace std; int main ... Read More

3K+ Views
The dot and arrow operator are both used in C++ to access the members of a class. They are just used in different scenarios. In C++, types declared as a class, struct, or union are considered "of class type". So the following refers to both of them.a.b is only used if b is a member of the object (or reference[1] to an object) a. So for a.b, a will always be an actual object (or a reference to an object) of a class.a →b is essentially a shorthand notation for (*a).b, ie, if a is a pointer to an object, ... Read More

1K+ Views
The dot and arrow operator are both used in C++ to access the members of a class. They are just used in different scenarios. In C++, types declared as a class, struct, or union are considered "of class type". So the following refers to both of them.a.b is only used if b is a member of the object (or reference[1] to an object) a. So for a.b, a will always be an actual object (or a reference to an object) of a class.a →b is essentially a shorthand notation for (*a).b, ie, if a is a pointer to an object, ... Read More

203 Views
The conditional operator (? :) is a ternary operator (it takes three operands). The conditional operator works as follows −The first operand is implicitly converted to bool. It is evaluated and all side effects are completed before continuing.If the first operand evaluates to true (1), the second operand is evaluated.If the first operand evaluates to false (0), the third operand is evaluated.The result of the conditional operator is the result of whichever operand is evaluated — the second or the third. Only one of the last two operands is evaluated in a conditional expression. The evaluation of the conditional operator ... Read More

2K+ Views
Unary operator is operators that act upon a single operand to produce a new value. The unary operators are as follows:OperatorsDescriptionIndirection operator (*)It operates on a pointer variable and returns an l-value equivalent to the value at the pointer address. This is called "dereferencing" the pointer.Address-of operator (&)The unary address-of operator (&) takes the address of its operand. The operand of the address-of operator can be either a function designator or an l-value that designates an object that is not a bit field and is not declared with the register storage-class specifier.Unary plus operator (+)The result of the unary plus ... Read More

481 Views
There are many types of operators in C++. These can be broadly categorized as: arithmetic, relational, logical, bitwise, assignment and other operators.Arithmetic OperatorsAssume variable A holds 10 and variable B holds 20, then −OperatorDescription + Adds two operands. A + B will give 30-Subtracts second operand from the first. A - B will give -10*Multiplies both operands. A * B will give 200/Divides numerator by de-numerator. B / A will give 2%Modulus Operator and remainder of after an integer division. B % A will give 0++Increment operator, increases integer value by one. A++ will give ... Read More

1K+ Views
The mutable storage class specifier is used only on a class data member to make it modifiable even though the member is part of an object declared as const. You cannot use the mutable specifier with names declared as static or const, or reference members.In the following example −class A { public: A() : x(4), y(5) { }; mutable int x; int y; }; int main() { const A var2; var2.x = 345; // var2.y = 2345; }the compiler would not allow the assignment var2.y = 2345 because var2 has been declared ... Read More