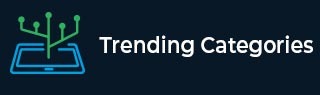
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

12K+ Views
Assuming a following text file (dict.txt) is present1 aaa2 bbb3 cccFollowing Python code reads the file using open() function. Each line as string is split at space character. First component is used as key and second as valued = {} with open("dict.txt") as f: for line in f: (key, val) = line.split() d[int(key)] = val print (d)The output shows contents of file in dictionary form{1: 'aaa', 2: 'bbb', 3: 'ccc'}

11K+ Views
In this article, we will show you how to zip a python dictionary and list together. Below are the various methods to accomplish this task − Using zip() function Using append() function and items() function Using append() function and in operator. Combing dictionary and list together using zip() Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Create a variable to store the input dictionary. Create another variable to store the input list. Use the zip() function(The zip() function can be used to combine two lists/iterators) to combine the input ... Read More

2K+ Views
Use dictionary comprehension technique.We have dictionary object having name and percentage of students>>> marks = { 'Ravi': 45.23, 'Amar': 62.78, 'Ishan': 20.55, 'Hema': 67.20, 'Balu': 90.75 }To obtain dictionary of name and marks of students with percentage>50>>> passed = { key:value for key, value in marks.items() if value > 50 } >>> passed {'Amar': 62.78, 'Hema': 67.2, 'Balu': 90.75}To obtain subset of given names>>> names = { 'Amar', 'Hema', 'Balu' } >>> lst = { key:value for key,value in marks.items() if key in names} >>> lst {'Amar': 62.78, 'Hema': 67.2, 'Balu': 90.75}

4K+ Views
Python dictionary doesn't allow key to be repeated. However, we can use defaultdict to find a workaround. This class is defined in collections module.Use list as default factory for defaultdict object>>> from collections import defaultdict >>> d=defaultdict(list)Here is a list of tuples each with two items. First item is found to be repeatedly used. This list is converted in defaultdict>>> for k,v in l: d[k].append(v)Convert this defaultdict in a dictionary object using dict() function>>> dict(d) {1: [111, 'aaa'], 2: [222, 'bbb'], 3: [333, 'ccc']}

4K+ Views
Python enumerate() function takes any iterable as argument and returns enumerate object using which the iterable can be traversed. It contains index and corresponding item in the iterable object like list, tuple, or string.Such enumerate object with index and value is then converted to a dictionary using dictionary comprehension.>>> l1=['aa','bb','cc','dd'] >>> enum=enumerate(l1) >>> enum >>> d=dict((i,j) for i,j in enum) >>> d {0: 'aa', 1: 'bb', 2: 'cc', 3: 'dd'} Learn Python with our step-by-step Python Tutorial.

694 Views
If sum of cubes of individual digits in a number add up to the number itself, it is called armstrong number. for example 153=1**3+5**3+3**3ExampleFollowing Python program find armstrong numbers between 100 to 1000for num in range(100,1000): temp=num sum=0 while temp>0: digit=temp%10 sum=sum+digit**3 temp=temp//10 if sum==num: print (num)OutputThe output is as follows −153 370 371 407

10K+ Views
In order to find factors of a number, we have to run a loop over all numbers from 1 to itself and see if it is divisible.Examplenum=int(input("enter a number")) factors=[] for i in range(1,num+1): if num%i==0: factors.append(i) print ("Factors of {} = {}".format(num,factors))If i is able to divide num completely, it is added in the list. Finally the list is displayed as the factors of given numberOutputenter a number75 Factors of 75 = [3, 5, 15, 25, 75]

8K+ Views
In this article, we will show you how to sort words in alphabetical order in Python. Below are the methods to sort the words in alphabetical order: Using bubble sort Using sorted() function Using sort() function Using bubble sort Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Create a variable to store an input string. Use the split() function (splits a string into a list. We can define the separator; the default separator is any whitespace) to split the input string into a list of words and create a variable ... Read More

654 Views
LCM (Least common multiple) of two (or more) numbers is a number which is the smallest number that is divisible by both (or all).First we find the larger number of two given numbers. Starting from it we try and find the first number that is divisible by both, which is LCMExamplex=12 y=20 if x > y: greater = x else: greater = y while(True): if((greater % x == 0) and (greater % y == 0)): lcm = greater break greater += 1 print ("LCM of {} and {}={}".format(x,y,lcm))OutputThe result is −LCM of 12 and 20=60