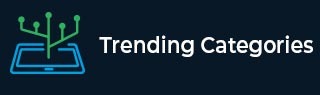
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

1K+ Views
There is no one elegant way to iterate the words of a C/C++ string. The most readable way could be termed as the most elegant for some while the most performant for others. I've listed 2 methods that you can use to achieve this. First way is using a stringstream to read words seperated by spaces. This is a little limited but does the task fairly well if you provide the proper checks. example#include #include #include using namespace std; int main() { string str("Hello from the dark side"); string tmp; ... Read More

2K+ Views
Object slicing is used to describe the situation when you assign an object of a derived class to an instance of a base class. This causes a loss of methods and member variables for the derived class object. This is termed as information being sliced away. For example, class Foo { int a; }; class Bar : public Foo { int b; }; Since Bar extends Foo, it now has 2 member variables, a and b. So if you create a variable bar of type Bar and then create ... Read More

2K+ Views
From MSDN docs −Use of two sequential underscore characters ( __ ) at the beginning of an identifier, or a single leading underscore followed by a capital letter, is reserved for C++ implementations in all scopes. You should avoid using one leading underscore followed by a lowercase letter for names with file scope because of possible conflicts with current or future reserved identifiers.So you should avoid using names like −__foo, __FOO, _FOOAnd names like the following should not be used in the global namespace −_foo, _barOther than this, there are some more prefixes like LC_, SIG_, and suffixes like _t ... Read More

396 Views
C++ has a standard library that contains common functionality you use in building your applications like containers, algorithms, etc. If names used by these were out in the open, for example, if they defined a queue class globally, you'd never be able to use the same name again without conflicts. So they created a namespace, std to contain this change.The using namespace statement just means that in the scope it is present, make all the things under the std namespace available without having to prefix std:: before each of them.While this practice is okay for example code, pulling in the ... Read More

139 Views
Just because we haven't reached the EOF, doesn't mean the next read will succeed.Consider you have a file that you read using file streams in C++. When writing a loop to read the file, if you are checking for stream.eof(), you're basically checking if the file has already reached eof. So you'd write the code like −Example#include #include using namespace std; int main() { ifstream myFile("myfile.txt"); string x; while(!myFile.eof()) { myFile >> x; // Need to check again if x is valid or eof if(x) { ... Read More

100 Views
The Rule of three is a rule of thumb when using C++. This is kind of a good practice rule that says that If your class needs any ofa copy constructor, an assignment operator, or a destructor, defined explicitly, then it is likely to need all three of them.Why is this? Its because, if your class requires any of the above, it is managing dynamically allocated resources and would likely be needing the other to successfully achieve that. For example, if you require an assignment operator, you would be creating copies of objects currently being copied by reference, hence allocating ... Read More

741 Views
When you instantiate a template in C++, the compiler creates a new class. This class has all the places where you placed the template arguments replaced with the actual argument you pass to it when using it. For example −template class MyClass { T foo; T myMethod(T arg1, T arg2) { // Impl } };And somewhere in your program use this class, MyClass x;The compiler creates a new class upon encountering this for every type argument you pass it. For example, if you created 3 objects with different template arguments you'll get 3 classes, ... Read More

608 Views
Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects", which may contain data, in the form of attributes; and instructions to do things, in the form of methods.For example, a person is an object which has certain properties such as height, gender, age, etc. It also has certain methods such as move, talk, and so on.ObjectThis is the basic unit of object oriented programming. That is both data and function that operate on data are bundled as a unit called as object.ClassWhen you define a class, you define a blueprint for an object. This doesn't actually ... Read More

27K+ Views
The & symbol is used as an operator in C++. It is used in 2 different places, one as a bitwise and operator and one as a pointer address of operator.Bitwise ANDThe bitwise AND operator (&) compares each bit of the first operand to that bit of the second operand. If both bits are 1, the bit is set to 1. Otherwise, the bit is set to 0. Both operands to the bitwise AND operator must be of integral types. Example #include using namespace std; int main() { unsigned short a = 0x5555; // pattern 0101 ... unsigned short b = 0xAAAA; // pattern 1010 ... cout