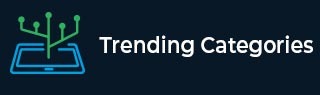
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

3K+ Views
We should avoid using global variables in any language, not only C++. This is because these variables pollute the global namespace, can cause some very nasty bugs in big projects as they can be accessed from any file and hence be modified from anywhere. These are some of the reasons why global variables are considered bad −Global variables can be altered by any part of the code, making it difficult to remember or reason about every possible use.A global variable can have no access control. It can not be limited to some parts of the program.Using global variables causes very ... Read More

366 Views
Forward declaration lets the code following the declaration know that there is are classes with the name Person. This satisfies the compiler when it sees these names used. Later the linker will find the definition of the classes. exampleClass Person; void myFunc(Person p1) { // ... } Class Person { // Class definition here };So in this case when the compiler encounters myFunc, it'll know that it's going to encounter this class somewhere down in the code. This can be used in cases where code using the class is placed/included before the code containing the class definition.

7K+ Views
argc stands for argument count and argv stands for argument values. These are variables passed to the main function when it starts executing. When we run a program we can give arguments to that program like −$ ./a.out helloExampleHere hello is an argument to the executable. This can be accessed in your program. For example,#include using namespace std; int main(int argc, char** argv) { cout

10K+ Views
static_cast − This is used for the normal/ordinary type conversion. This is also the cast responsible for implicit type coersion and can also be called explicitly. You should use it in cases like converting float to int, char to int, etc.dynamic_cast −This cast is used for handling polymorphism. You only need to use it when you're casting to a derived class. This is exclusively to be used in inheritence when you cast from base class to derived class.Regular Cast − This is the most powerful cast available in C++ as it combines const_cast, static_cast and reinterpret_cast. but it's also unsafe ... Read More

42K+ Views
C++ does not allow to pass an entire array as an argument to a function. However, You can pass a pointer to an array by specifying the array's name without an index. There are three ways to pass a 2D array to a function −Specify the size of columns of 2D arrayvoid processArr(int a[][10]) { // Do something }Pass array containing pointersvoid processArr(int *a[10]) { // Do Something } // When callingint *array[10]; for(int i = 0; i < 10; i++) array[i] = new int[10]; processArr(array);Pass a pointer to a pointervoid processArr(int **a) { // ... Read More

58 Views
The following are most common causes of undefined behaviour in C++ programming. Note that all of these are specified in the standard to lead to undefined behaviour and should be avaoided at all costs when writing programs. Signed integer overflowDereferencing a NULL pointer, a pointer returned by a "new" allocation of size zero, pointer that has not yet been definitely initialized, pointer at a location beyond the end of an array. Using pointers to objects which have gone out of scope or have been deleted Performing pointer arithmetic that yields a result outside the boundaries of an array.Converting pointers to objects of incompatible ... Read More

236 Views
The members and base classes of a struct are public by default, while in class, they default to private. Struct and class are otherwise functionally equivalent.They are however used in different places due to semantics. a struct is more like a data structure that is used to represent data. class, on the other hand, is more of a functionality inclined construct. It mimics the way things are and work.

1K+ Views
The only safe way is to check for overflow before it occurs. There are some hacky ways of checking for integer overflow though. So if you're aiming for detecting overflow in unsigned int addition, you can check if the result is actually lesser than either value-added. So for example, unsigned int x, y; unsigned int value = x + y; bool overflow = value < x; // Alternatively "value < y" should also workThis is because if x and y are both unsigned ints if added and they overflow, their values can't be greater than either of them as it ... Read More

587 Views
There are four ways of passing objects to functions. Let's assume you have a class X and want to pass it to a function fun, then − Pass by value This creates a shallow local copy of the object in the function scope. Things you modify here won't be reflected in the object passed to it. For example, Declaration void fun(X x); Calling X x; fun(x); Pass by reference This passes a reference to the object to the function. Things you modify here will be reflected in the object passed to it. No copy of the ... Read More

1K+ Views
This is due to the fact that C++ does not do bounds checking. Languages like Java and python have bounds checking so if you try to access an out of bounds element, they throw an error. C++ design principle was that it shouldn't be slower than the equivalent C code, and C doesn't do array bounds checking.So if you try to access this out of bounds memory, the behavior of your program is undefined as this is written in the C++ standard. In general, whenever you encounter undefined behavior, anything might happen. The application may crash, it may freeze, it ... Read More