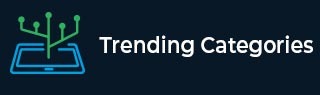
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

9K+ Views
A segmentation fault occurs when your program attempts to access an area of memory that it is not allowed to access. In other words, when your program tries to access memory that is beyond the limits that the operating system allocated for your program.Seg faults are mostly caused by pointers that are −Used to being properly initialized.Used after the memory they point to has been reallocated or freed.Used in an indexed array where the index is outside of the array bounds.

322 Views
A process dumps core when it is terminated by the operating system due to a fault in the program. The most typical reason this occurs is that the program accessed an invalid pointer value like NULL or some value out of its memory area. As part of that process, the operating system tries to write our information to a file to allow us to analyze what happened.This core can be used as follows to diagnose and debug our program −The core is dumped to the /proc/sys/kernel directory by default. To debug a core, the program must be compiled with the ... Read More

3K+ Views
The explicit keyword in C++ is used to mark constructors to not implicitly convert types. For example, if you have a class Foo −class Foo { public: Foo(int n); // allocates n bytes to the Foo object Foo(const char *p); // initialize object with char *p };Now if you tryFoo mystring = 'x';The char 'x' is implicitly converted to int and then will call the Foo(int) constructor. But this is not what was intended. So to prevent such conditions and make the code less error-prone, define the constructor as explicit −Example class Foo { public: ... Read More

2K+ Views
Standard C++ doesn't provide a way to do this. You could use the system command to initialize the ls command as follows −Example#include int main () { char command[50] = "ls -l"; system(command); return 0; }OutputThis will give the output −-rwxrwxrwx 1 root root 9728 Feb 25 20:51 a.out -rwxrwxrwx 1 root root 131 Feb 25 20:44 hello.cpp -rwxrwxrwx 1 root root 243 Sep 7 13:09 hello.py -rwxrwxrwx 1 root root 33198 Jan 7 11:42 hello.o drwxrwxrwx 0 root root 512 Oct 1 21:40 hydeout -rwxrwxrwx 1 root root 42 Oct 21 11:29 ... Read More

345 Views
Undefined behavior is a way to give freedom to implementors (e.g. of compilers or of OSes) and to computers to do whatever they "want", in other words, to not care about consequences.The cases in which segmentation fault occurs are transient in nature. They won't always result in a segmentation fault but can also run correctly(or at least appear to). For example, consider the following code fragment −#include int main() { int arr[2]; arr[0] = 0; arr[1] = 1; arr[2] = 2; // Undefined behaviour arr[3] = 3; // Undefined behaviour ... Read More

12K+ Views
You can sort a vector of custom objects using the C++ STL function std::sort. The sort function has an overloaded form that takes as arguments first, last, comparator. The first and last are iterators to first and last elements of the container. The comparator is a predicate function that can be used to tell how to sort the container. example#include #include #include using namespace std; struct MyStruct { int key; string data; MyStruct(int key, string data) { this -> key = key; this -> data = data; } }; int ... Read More

11K+ Views
You can use the popen and pclose functions to pipe to and from processes. The popen() function opens a process by creating a pipe, forking, and invoking the shell. We can use a buffer to read the contents of stdout and keep appending it to a result string and return this string when the processes exit.example#include #include #include #include using namespace std; string exec(string command) { char buffer[128]; string result = ""; // Open pipe to file FILE* pipe = popen(command.c_str(), "r"); if (!pipe) { ... Read More

767 Views
Variables can be declared in a switch statement. You'll just need to declare them and use them within a new scope in the switch statement. For example,Example#include using namespace std; int main() { int i = 10; switch(i) { case 2: //some code break; case 10:{ int x = 13; cout

763 Views
In C++, there is no difference between 'struct' and 'typedef struct' because, in C++, all struct/union/enum/class declarations act like they are implicitly typedef'ed, as long as the name is not hidden by another declaration with the same name.Though there is one subtle difference that typedefs cannot be forward declared. So for the typedef option, you must include the file containing the typedef before it is used anywhere.