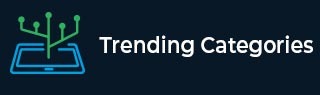
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

13K+ Views
This is a simple way to read whole ASCII file into std::string in C++ −AlgorithmBegin Declare a file a.txt using file object f of ifstream type to perform read operation. Declare a variable str of string type. If(f) Declare another variable ss of ostringstream type. Call rdbuf() fuction to read data of file object. Put the data of file object in ss. Put the string of ss into the str string. Print the value of str. End.Example#include #include #include #include using namespace std; int ... Read More

4K+ Views
The main reason for segmentation fault is accessing memory that is either not initialized, out of bounds for your program or trying to modify string literals. These may cause a segmentation fault though it is not guaranteed that they will cause a segmentation fault. Here are some of the common reasons for segmentation faults −Accessing an array out of boundsDereferencing NULL pointersDereferencing freed memoryDereferencing uninitialized pointersIncorrect use of the "&" (address of) and "*" (dereferencing) operatorsImproper formatting specifiers in printf and scanf statementsStack overflowWriting to read-only memory

6K+ Views
Data hiding is one of the important features of Object Oriented Programming which allows preventing the functions of a program to access directly the internal representation of a class type. The access restriction to the class members is specified by the labeled access modifiers − public, private, and protected sections within the class body.The default access for members and classes is private.Exampleclass Base { public: // public members go here protected: // protected members go here private: // private members go here };A public member is accessible from anywhere outside the class but within a program. ... Read More

933 Views
The output stream cout allows using manipulators that you can use to set the precision directly on cout and use the fixed format specifier. To get the full precision of a double, you can use the limits library. For example,Example#include #include using namespace std; int main() { // Get numeric limits of double typedef std::numeric_limits< double > dbl; double PI = 3.14159265358979; cout.precision(dbl::max_digits10); cout

4K+ Views
The compilation of a C++ program consists of three steps −Preprocessing − In simple terms, a C Preprocessor is just a text substitution tool and it instructs the compiler to do required pre-processing before the actual compilation. It handles preprocessing directives like #include, #define, etc.Compilation − The compilation takes place on the preprocessed files. The compiler parses the pure C++ source code and converts it into assembly code. This in turn calls the assembler that converts the assembly code to machine code(binary) as Object files. These Object files can refer to symbols that are not defined. The compiler won't give ... Read More

1K+ Views
You can use a string stream to parse an int in c++ to an int. You need to do some error checking in this method.example#include #include using namespace std; int str_to_int(const string &str) { stringstream ss(str); int num; ss >> num; return num; } int main() { string s = "12345"; int x = str_to_int(s); cout

3K+ Views
A portable solution doesn't exist for doing this. On windows, you can use the getch() function from the conio(Console I/O) library to get characters pressed.example#include #include using namespace std; int main() { char c; while(1){ // infinite loop c = getch(); cout

2K+ Views
C++ has header and .ccp files for separating the interface from the implementation. The header files declare "what" a class (or whatever is being implemented) will do, ie the API of the class, kind of like an interface in Java. The cpp file on the other hand defines "how" it will perform those features, ie, the implementation of these declared functionality.This reduces dependencies. The code that uses the header doesn't need to know all the details of the implementation and any other classes/headers needed only for that. It just needs to focus on things it is trying to implement. This ... Read More

603 Views
Calling virtual functions from a constructor or destructor is dangerous and should be avoided whenever possible. This is because the virtual function you call is called from the Base class and not the derived class.In C++ every class builds its version of the virtual method table prior to entering its own construction. So a call to the virtual method in the constructor will call the Base class' virtual method. Or if it has no implementation at that level, it'll produce a pure virtual method call. Once the Base is fully constructed, the compiler starts building Derived class, and overrides the ... Read More