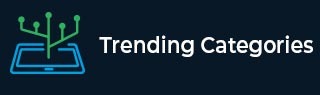
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

297 Views
@ symbol is used to define decorator in Python. Decorators provide a simple syntax for calling higher-order functions. By definition, a decorator is a function that takes another function and extends the behavior of the latter function without explicitly modifying it.we have two different kinds of decorators in Python:Function decoratorsClass decorators A decorator in Python is any callable Python object that is used to modify a function or a class. A reference to a function or a class is passed to a decorator and the decorator returns a modified function or class. The modified functions or classes usually contain calls to ... Read More

3K+ Views
Python has magic methods to define overloaded behaviour of operators. The comparison operators (=, == and !=) can be overloaded by providing definition to __lt__, __le__, __gt__, __ge__, __eq__ and __ne__ magic methods. Following program overloads < and > operators to compare objects of distance class. class distance: def __init__(self, x=5,y=5): self.ft=x self.inch=y def __eq__(self, other): if self.ft==other.ft and self.inch==other.inch: return "both objects are equal" else: return "both objects are not equal" def __lt__(self, other): in1=self.ft*12+self.inch in2=other.ft*12+other.inch if in1

40K+ Views
CSV (Comma Separated Values) is a most common file format that is widely supported by many platforms and applications.Use csv module from Python's standard library. Easiest way is to open a csv file in 'w' mode with the help of open() function and write key value pair in comma separated form.import csv my_dict = {'1': 'aaa', '2': 'bbb', '3': 'ccc'} with open('test.csv', 'w') as f: for key in my_dict.keys(): f.write("%s, %s"%(key, my_dict[key]))The csv module contains DictWriter method that requires name of csv file to write and a list object containing field names. The writeheader() ... Read More

8K+ Views
In this article, we will show you how to split python tuples into sub-tuples. Below are the various methods to accomplish this task − Using slicing Using enumerate() & mod operator Tuples are an immutable, unordered data type used to store collections in Python. Lists and tuples are similar in many ways, but a list has a variable length and is mutable in comparison to a tuple which has a fixed length and is immutable. Using slicing Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Create a variable to ... Read More

188 Views
Argument-dependent lookup(ADL) is a protocol for looking up unqualified function names in function-call expressions.These function call expressions include implicit function calls to overloaded operators.The function names are looked up in the namespaces of their arguments in addition to the scopes and namespaces considered by the usual unqualified name lookup. Argument-dependent lookup makes it possible to use operators defined in a different namespace. Examplenamespace MyNamespace{ class A {}; void f( A &a, int i) {} } int main() { MyNamespace::A a; f( a, 0 ); //calls MyNamespace::f }The lookup of a function call to f was dependent ... Read More

2K+ Views
The Standard Template Library (STL) is a software library for the C++ programming language that influenced many parts of the C++ Standard Library. It provides four components called algorithms, containers, functions, and iterators. Note that the term "STL" or "Standard Template Library" does not show up anywhere in the ISO 14882 C++ standard. So referring to the C++ standard library as STL is wrong, ie, STL and C++ Standard Library are 2 different things with the former being the subset of the latter.The STL consists ofContainersThe STL contains sequence containers and associative containers. Containers are objects that store data. The ... Read More

50 Views
new is used for dynamic memory allocation. The memory allocated in this case goes on the heap. There are several costs associated with this type of memory allocation along with the programmer having to do manual memory cleaning and management. This type of allocation must be used when − You don't know how much memory you need at compile time.You want to allocate memory which will persist after leaving the current block.Other than these, there are very few cases where dynamic memory allocation is required. This is because, in C++, there is the concept of a destructor. This function gets called ... Read More

2K+ Views
A translation unit is any preprocessed source file.A translation unit is the basic unit of compilation in C++. This unit is made up of the contents of a single source file after it passes through preprocessing. It contains included any header files without blocks that are ignored using conditional preprocessing statements like ifdef, ifndef, etc.A single translation unit can be compiled into an object file, library, or executable program.