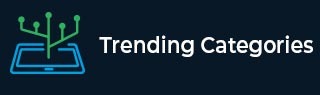
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

5K+ Views
In this article, we will show how to randomly select from a range in python. Below are the various methods to accomplish this task − Using random.randrange() function Using random.randint() function Using random.random() function Using random.sample() Using random.randrange() function Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Use the import keyword, to import the random module. Use the random.randrange() function(Returns a random number within the specified range) to generate a random number within the given range by passing minimum, and maximum numbers as arguments. Generate a random number from ... Read More

2K+ Views
In this article, we will show you how to pick a random number not in a list in python. Below are the various methods to accomplish this task − Using random.choice() function Using random.choice() function and List Comprehension Using random.choice() & set() functions Using random.randrange() function Using random.choice() function The random.choice() method returns a random element from the specified sequence. The sequence could be a string, a range, a list, a tuple, or anything else. Syntax random.choice(sequence) Parameters sequence − any sequence like list, tuple etc. Algorithm (Steps) Following are the Algorithm/steps to be followed to perform ... Read More

8K+ Views
In this article, we will show you how to randomly select an item from a string using python. Below are the various methods in python to accomplish this task − Using random.choice() method Using random.randrange() method Using random.randint() method Using random.random() Using random.sample() method Using random.choices() method Assume we have taken a string containing some elements. We will generate a random element from the given input string using different methods as specified above. Method 1: Using random.choice() method Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Use the import keyword ... Read More

4K+ Views
In this article, we will show you how to randomly select an item from a tuple using python. Below are the various methods in python to accomplish this task: Using random.choice() method Using random.randrange() method Using random.randint() method Using random.random() Using random.sample() method Using random.choices() method Assume we have taken a tuple containing some elements. We will generate a random element from the given input tuple using different methods as specified above. Using random.choice() method Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task – Use the import keyword to ... Read More

218 Views
Floor and modulus operators (// and % respectively) are not allowed to be used on complex number in Python 3.x. However, these operations are defined for complex numbers in Python 2.7.xPython 3>>> x=9+2j >>> y=2+1j >>> x%y Traceback (most recent call last): File "", line 1, in x%y TypeError: can't mod complex numbers.Python 2.7>>> x=9+2j >>> y=2+1j >>> x%y (1-2j)Modulus of complex number operands returns their floor division multiplied by denominator>>> x-(x//y)*y (1-2j)

894 Views
The random module in Numpy package contains many functions for generation of random numbersnumpy.random.rand() − Create an array of the given shape and populate it with random samples>>> import numpy as np >>> np.random.rand(3,2) array([[0.10339983, 0.54395499], [0.31719352, 0.51220189], [0.98935914, 0.8240609 ]])numpy.random.randn() − Return a sample (or samples) from the “standard normal” distribution.>>> np.random.randn() -0.6808986872330651numpy.random.randint() − Return random integers from low (inclusive) to high (exclusive).>>> np.random.randint(5, size=(2, 4)) array([[2, 4, 0, 4], [3, 4, 1, 2]])numpy.random.random() − Return random floats in the half-open interval [0.0, 1.0).>>> np.random.random_sample() 0.054638060174776126

175 Views
Python has an in-built function isdigit() which returns true if all characters in a string are digit (between 0-9)>>> string='9764135408' >>> string.isdigit() True >>> string='091-9764135408' >>> string.isdigit() FalseYou can also use regex expression to check if string contains digits only.>>> import re >>> bool(re.match('^[0-9]+$','9764135408')) True >>> bool(re.match('^[0-9]+$','091-9764135408')) False

40K+ Views
In this article, we will show you how to generate non-repeating random numbers in Python. Below are the methods to accomplish this task: Using randint() & append() functions Using random.sample() method of given list Using random.sample() method of a range of numbers Using random.choices() method Using randint() & append() functions Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task − Use the import keyword, to import the random module. Create an empty list which is the resultant random numbers list. Use the for loop, to traverse the loop 15 times. Use ... Read More

12K+ Views
Python has magic methods to define overloaded behaviour of operators. The comparison operators (=, == and !=) can be overloaded by providing definition to __lt__, __le__, __gt__, __ge__, __eq__ and __ne__ magic methods. Following program overloads == and >= operators to compare objects of distance class.class distance: def __init__(self, x=5, y=5): self.ft=x self.inch=y def __eq__(self, other): if self.ft==other.ft and self.inch==other.inch: return "both objects are equal" ... Read More