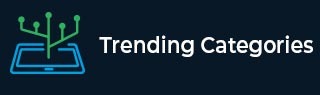
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

153K+ Views
In this article, we will show you how to round off a floating number upto 2 decimals in python. Below are the various methods to accomplish this task: Using round() function Using format() function Using Decimal Module Using ceil() function Using round() function The round() function gives a floating point number with a specified number of decimals which is the rounded version of the specified number. The function will return the nearest integer because the default value for the number of decimals is 0. Syntax round(number, digits) Parameters number(required)- a number that should be rounded digits(optional)- ... Read More

778 Views
Following program accepts a number and index from user. The recursive funcion rpower() uses these two as arguments. The function multiplies the number repeatedly and recursively to return power.Exampledef rpower(num,idx): if(idx==1): return(num) else: return(num*rpower(num,idx-1)) base=int(input("Enter number: ")) exp=int(input("Enter index: ")) rpow=rpower(base,exp) print("{} raised to {}: {}".format(base,exp,rpow))OutputHere is a sample run −Enter number: 10 Enter index: 3 10 raised to 3: 1000

13K+ Views
In this article, we will show you how to find the power of a number in python. Below are the various methods to accomplish this task − Using for loop Using Recursion Using pow() function Using ** operator Using for loop Algorithm (Steps) Following are the Algorithm/steps to be followed to perform the desired task –. Create a function findPower() that returns the power of a Number. The function accepts number, and exponent value as parameters. Take a variable that stores the result and initialize its value with 1. To obtain the power, multiply the given ... Read More

97 Views
The method modf() returns the fractional and integer parts of x in a two-item tuple. Both parts have the same sign as x. The integer part is returned as a float. First item in tuple is fractional part>>> import math >>> math.modf(100.73) (0.730000000000004, 100.0) >>> math.modf(-5.55) (-0.5499999999999998, -5.0)

8K+ Views
In this article, we will show you how to find the exponential value in python. Below are the various methods to accomplish this task − Using exponential operator(**) Using exp() function Using exp() values with inbuilt numbers Using pow() function Using exponential operator(**) The exponential operator(**) can be used to calculate the power of a number. Since importing a module or calling a function is not necessary, this is the most convenient to use. The exponential value of an x is xth power of e, Euler's constant which is an irrational number called Euler's number and is equal ... Read More

3K+ Views
If a function calls itself, it is called a recursive function. In order to prevent it from falling in infinite loop, recursive call is place in a conditional statement.Following program accepts a number as input from user and sends it as argument to rsum() function. It recursively calls itself by decrementing the argument each time till it reaches 1.def rsum(n): if n

9K+ Views
In this article, we will show you how to represent an infinite number in python. Infinity is an undefined number that can be either positive or negative. A number is used to represent infinity; the sum of two numeric values may be a numeric but distinct pattern; it may have a negative or positive value. All arithmetic operations on an infinite value, whether sum, subtraction, multiplication or any other operation, always result in an infinite number. In the field of computer science, infinity is commonly used to evaluate and optimize algorithms that do calculations on a huge scale. Infinity in ... Read More

1K+ Views
In this article, we will show you how to find the smallest number greater than x in Python. Introduction to Ceil Function in Python Ceil is a function in Python's math module, which is included in the Python Standard Library. In mathematics, it is the same as the Least Integer Function or the Ceil Function. If you are given a real integer x, ceil(x) is represented in mathematical notation as ⌈x⌉, where the upper direction of the brackets corresponds to ceiling operation (as the ceiling lies above your head). In contrast, the floor(x) (which returns the greatest integer ≤x) is ... Read More