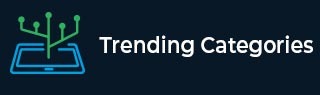
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

87 Views
Finding the kth digit sum means we must find the sum of all the digits at the kth index of a list of numbers. This is an important programming concept, and we use this in data validation, financial analysis, etc. In this article, we will explore how to find the kth digit sum with the help of several custom methods like loops, list comprehensions, lambda, map functions, etc. We will also use libraries like Numpy and Functool to achieve the same. Understanding The Problem Statement Suppose we have the following inputs: list: [12, 73, 64] k=0 ... Read More

84 Views
List is an important data type in Python that can hold sequence of either homogeneous or heterogeneous data types. They are mutable. Finding the k summation from two lists means we need to find the combinations of the elements of two lists, which can add up to make the sum exactly equal to k. In this article, we will first explore the brute force method. Next, we would look into several optimized algorithms like two pointer approach, hashing algorithms, list comprehensions, etc. Using The Brute Force Method Brute force is the simplest approach. We write the logic without considering ... Read More

296 Views
The list is a popular mutable data type in Python that can hold heterogeneous data. We can access the list elements by using a process called indexing. The indexing means the distance of any element from the first element; hence it starts from 0. This article will explore how to find the k maximum elements and index number. We will achieve this through several methods like brute force, recursion, heapq module, Numpy library, enumerate functions, etc. We will also explore the time and space complexity of the algorithms used. Using The Brute Force Method Brute force is the simplest ... Read More

200 Views
Matrix is a popular data representation technique in mathematics, machine modeling, etc. They are designed to deal with linear functions. Matrix initialization is a process to fill up the elements (rows and columns) of the matrix with either random or some predetermined values. After initialization there should be no undefined entries in the matrix. Initializing the matrix is one of the essential tasks in several fields, like competitive programming, machine and deep learning algorithms. In this article, we will learn how to initialize the matrix using various methods like loops, Numpy arrays, etc. We would also explore different types of ... Read More

115 Views
In computer science we define decimal numbers to be the numbers which utilize base10 notations. For example 12, 4, 5.7, 99 are decimal numbers because they utilize base 10 notation. We can define the length of a decimal number as the number of digits present in the decimal number. More precisely, the number of significant figures present in the decimal number includes both digits after and before the decimal parts. This article will explore several methods to find the kālength decimal places like the round method, String formatting, Global precisions, etc. Using round Method One of the ... Read More

922 Views
Consecutive characters are those characters that appear one after the other. k length consecutive characters mean the same character appearing k times consecutively. In this article, we will adopt several methods to achieve it. We will start with brute force by using loop statements. Next, we will perform the same using regular expressions, sliding window techniques, etc. A sliding window is a better and optimized way to find the k-length consecutive characters. Numpy Library also offers us methods to adopt similar techniques. Using the Brute Force Method Brute force is a simple algorithm that we can think about without ... Read More

219 Views
K length combinations from given characters mean the combinations of characters that we can create with the given characters, which are exactly of length k. In this article, we will explore several methods to achieve this, like recursion, map and lambda function, itertools library, etc. While recursion and lambda functions are custom functions, the itertools provide the in-built method to generate the combinations. Using Recursion Recursion is one of the traditional programming techniques. In this technique, we try to break a large problem into smaller chunks of problems, and our motive is to solve the smaller problems to solve ... Read More

942 Views
Understanding function signatures in Python is essential for unraveling the inner workings of functions. The inspect module offers a range of methods to retrieve signatures, allowing you to analyze parameters, access data types, and determine default values. By exploring these techniques, you can gain valuable insights that enhance code readability, maintainability, and documentation. So, dive into the world of function signatures and unlock a deeper understanding of your Python code. Using inspect Method The inspect method provides a powerful way to retrieve the function signatures. This method enables us to access detailed information about a function's parameters, data types, default ... Read More

140 Views
The Tuple is one of the most important data types in Python. They are exclusively used as the data structures to pass sequential data as the parameters of any method. Indexing refers to accessing the elements of the sequential data through the index. In this article, we will learn how to get an even index in elements of Tuple. Use The List And range Expression. The mutable lists sequences of data separated by ", " and enclosed by "[ ]". Python allows the conversion between the lists and the Tuples through the in-built methods. We can traverse the lists and ... Read More

151 Views
One of the most frequent tasks in web automation and testing is finding objects on a webpage. There are several ways to locate components with Selenium WebDriver, a potent tool for programmatically managing a web browser. The many locator strategies in Selenium Python will be covered in this post, with examples to help you along the way. Introduction The first step in using Selenium WebDriver to interact with a web element on a webpage, such as clicking a button or typing text into a textbox, is to locate the element. Eight alternative locators, or methods, are supported by selenium for ... Read More