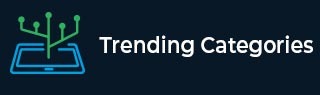
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming
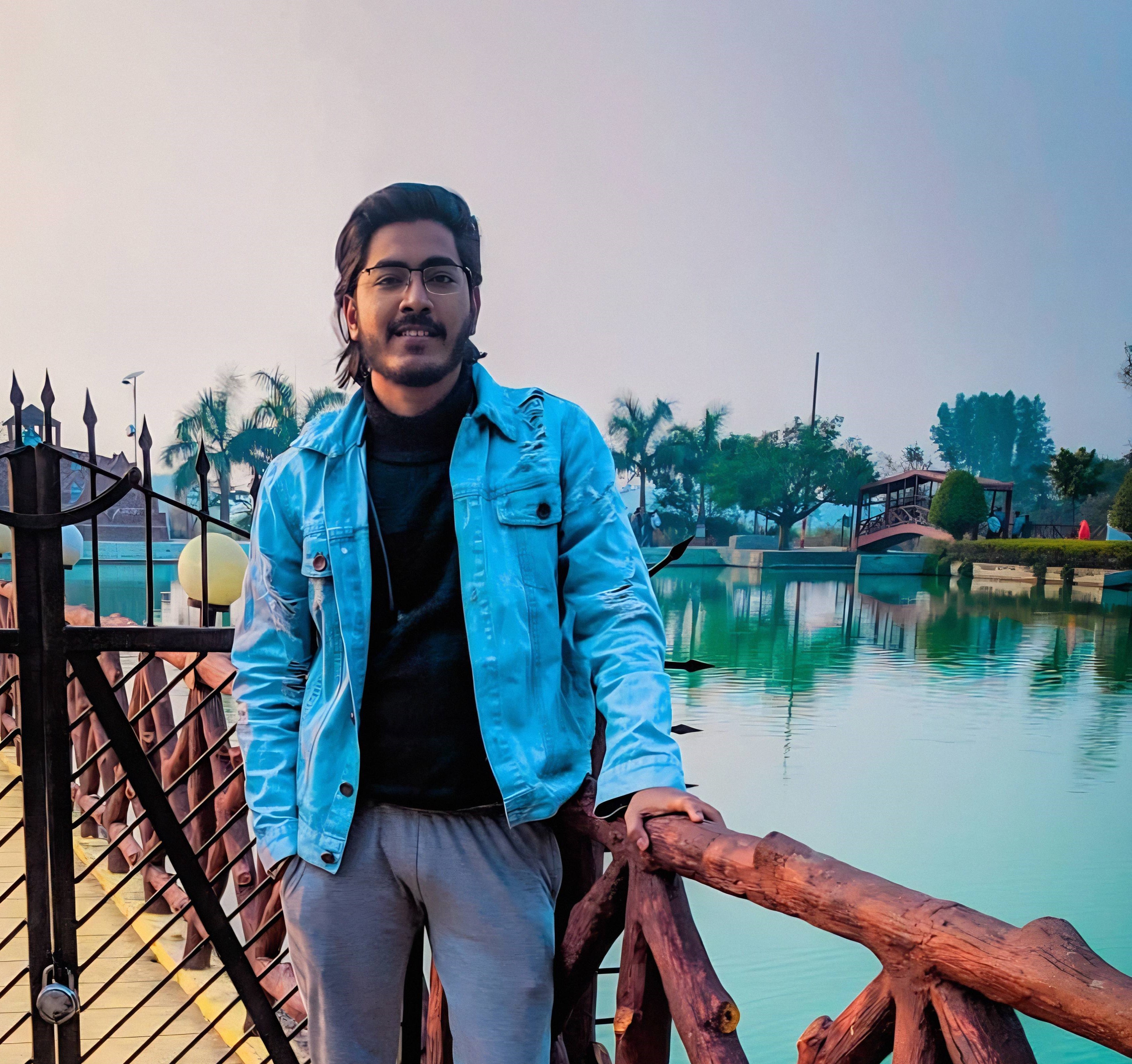
773 Views
In Python, we can group similar value list to dictionary using methods like using for loop and conditional statement, using defaultdict, and using groupby methods from the itertools module. Grouping them together can be useful when analyzing complex data. In this article, we will understand how we can group similar value list to dictionary using these methods. Method 1: Using a for loop and conditional statements One of the simplest methods to group similar values in a list and convert them into a dictionary is by using a for loop and conditional statements. In this method, we initialize an empty ... Read More
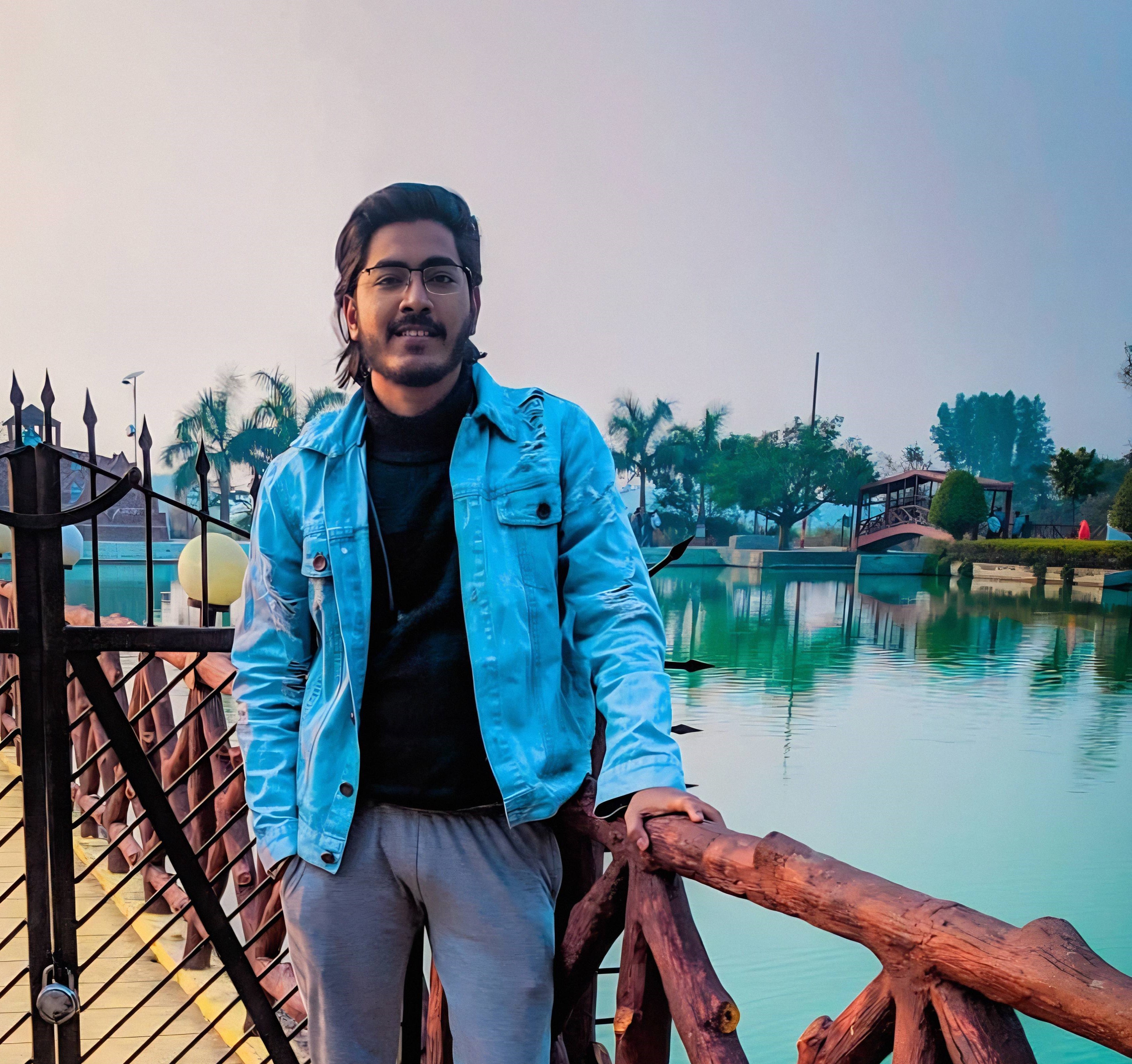
1K+ Views
In Python, similar keys in the dictionary can be grouped using various methods in Python as using defaultdict, using a dictionary of lists, using the itertools module, and the groupby function. During data analysis sometimes we may need to group similar keys together in a dictionary based on certain criteria. In this article, we will explore various methods to group similar keys in dictionaries. Method 1: Using a defaultdict Python's defaultdict class from the collections module provides a convenient way to group similar keys. It automatically initializes a default value when a new key is accessed. Syntax groups = defaultdict(list) ... Read More
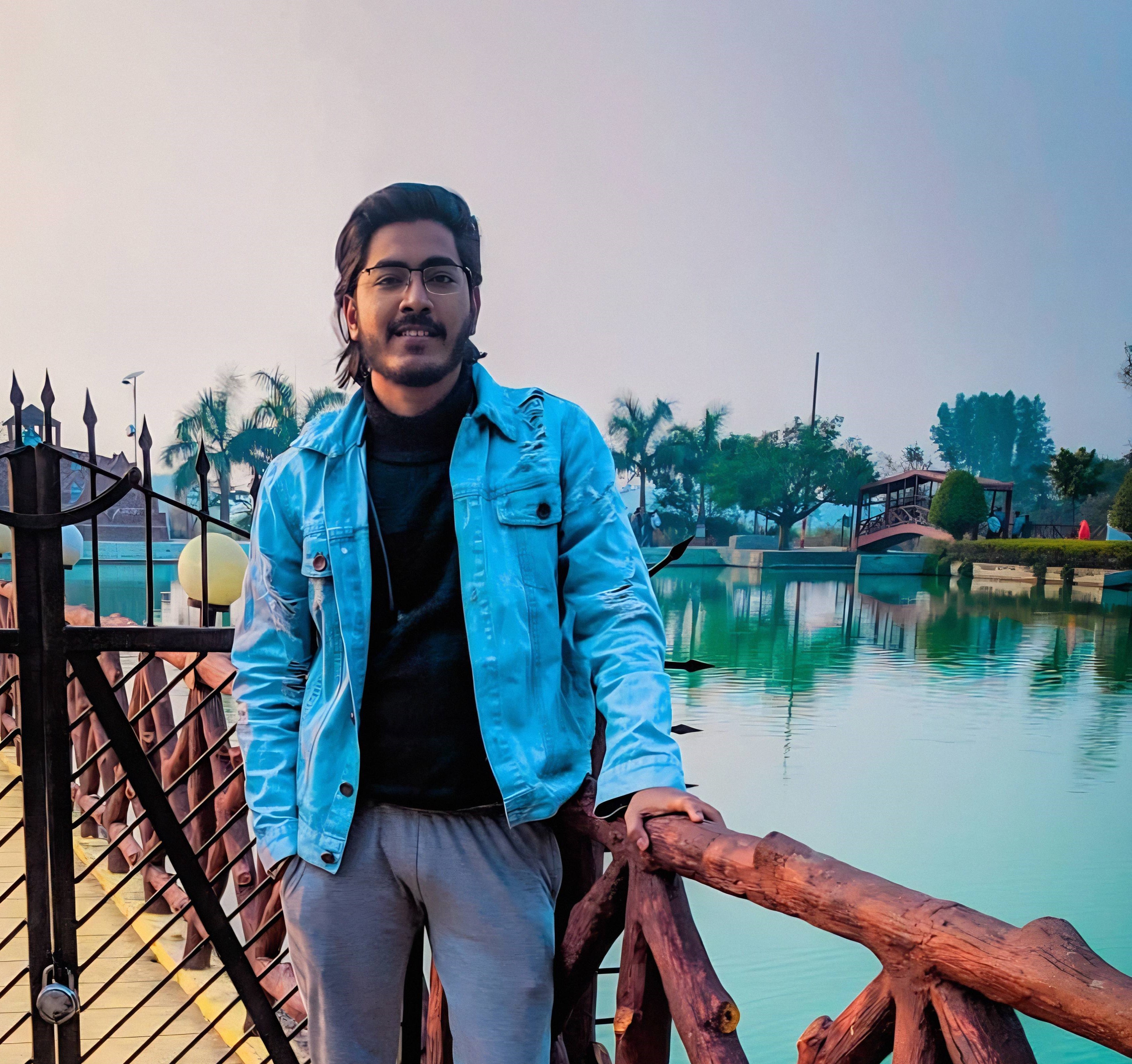
133 Views
In data analysis and processing, it is necessary to group similar elements together for better organization and analysis of data. Python provides several methods to group elements into a dictionary value list efficiently in Python, using for loop, using defaultdict, and using itertools.groupby methods. In this article, we will explore different approaches to Group Similar items to Dictionary Values List in Python. Method 1: Using a for loop The simplest way to group similar items into dictionary value lists is by using a for loop. Let's consider an example where we have a list of fruits, and we want to ... Read More
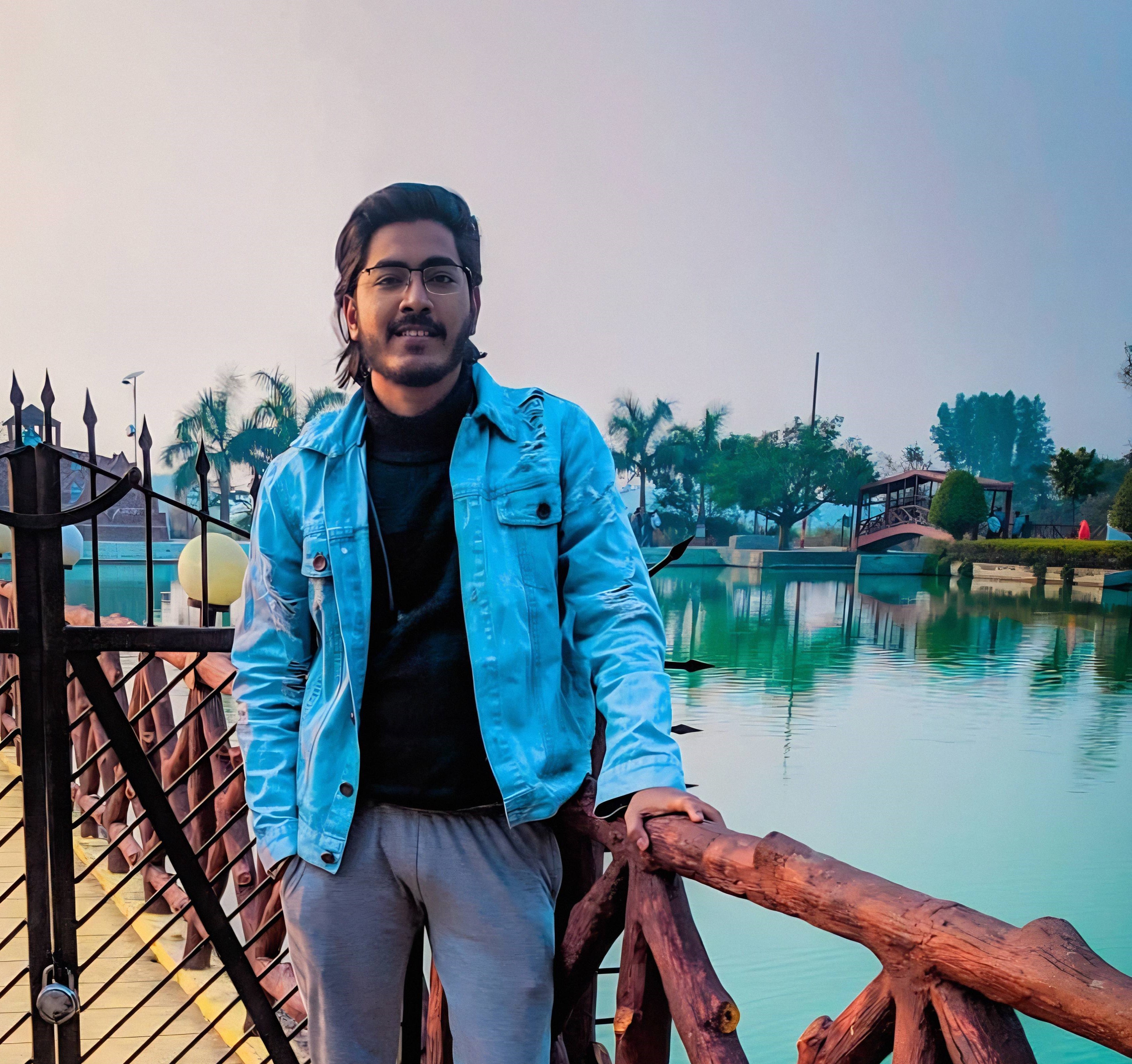
310 Views
In Python, we can group list of tuples to a dictionary using different methods like using a for loop and conditional statements, using the defaultdict class from the collections module, and using the groupby() function from the itertools module. In this article we will explore all these methods and implement them to group a list of tuples to dictionary. Method 1: Using a for loop and conditional statements This method involves using a for loop to iterate over the list of tuples. We will check if the key exists in the dictionary and add the corresponding tuple as a value ... Read More
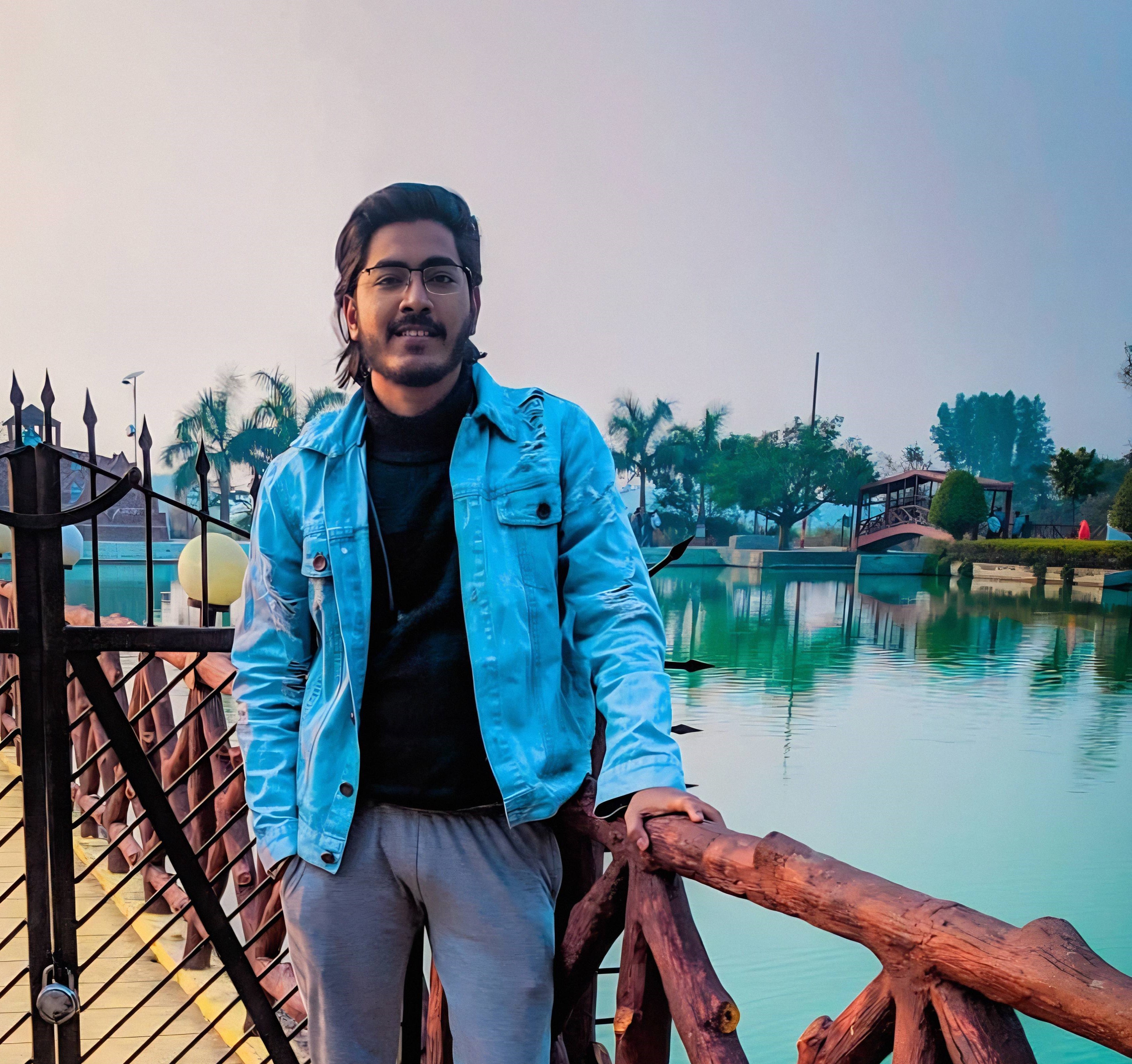
488 Views
In Python, the grouping of elements in a tuple list based on the values of their second elements can be done using various methods like using a dictionary or using itertools.groupby() method, and using defaultdict from collections. Group first elements by second elements in the Tuple list means the tuple having the same second element can be grouped into a single group of elements. In this article, we will discuss, how we can implement these methods so that we are able to easily group first elements based on second elements in a tuple list. Method 1: Using a Dictionary This ... Read More
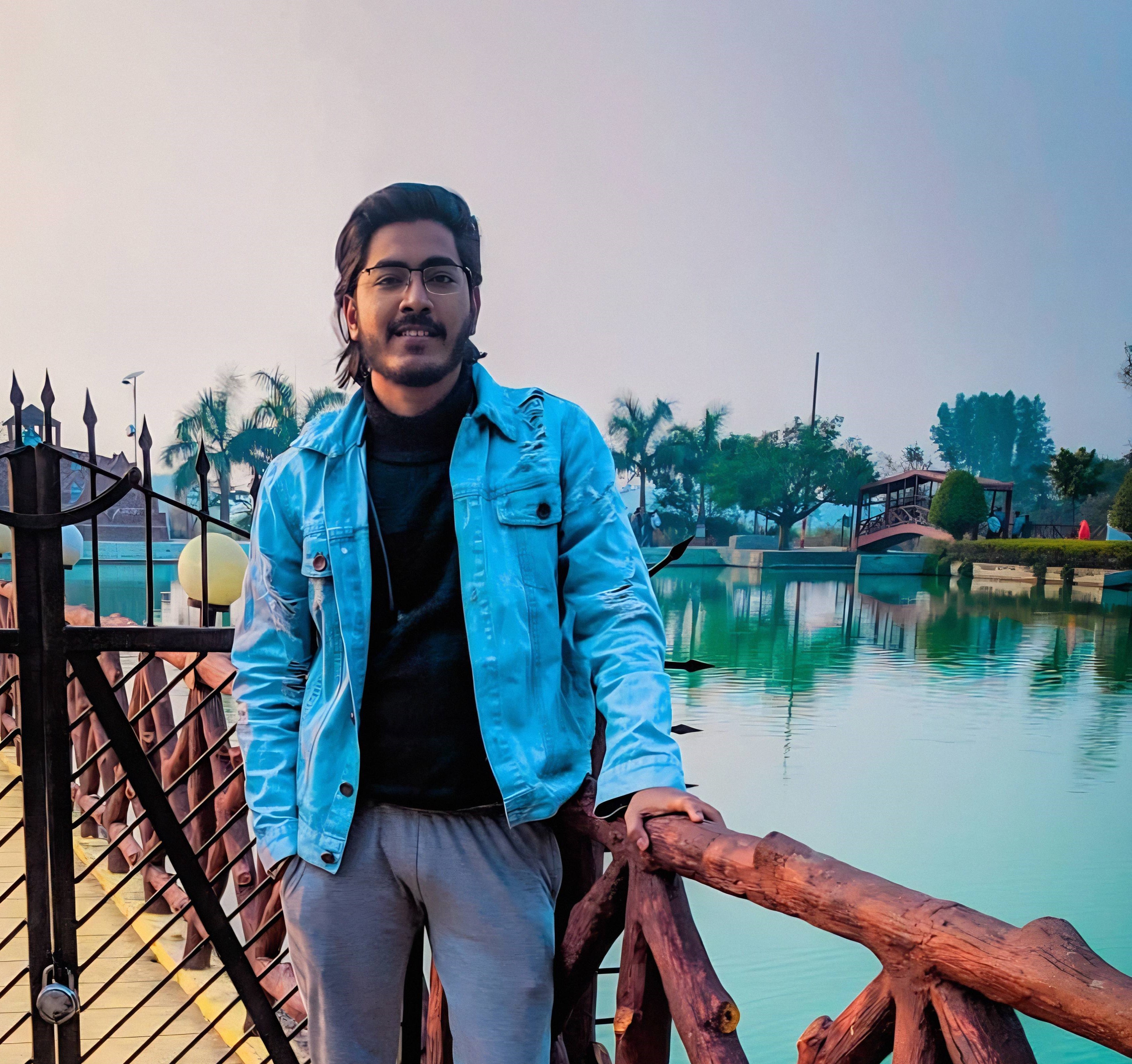
54 Views
Group concatenate till K means concatenating elements within a group or sequence until a specific condition is met. In Python we can group concatenate till K using various methods like using a loop and accumulator, Using itertools.groupby(), and using regular expressions. In this article, we will use and explore all these methods to Group concatenate till K or a certain condition is met. Method 1: Using a Loop and Accumulator This method utilizes a loop and an accumulator to group elements until the target value K is encountered. It iterates through the list, accumulating elements in a temporary group until ... Read More
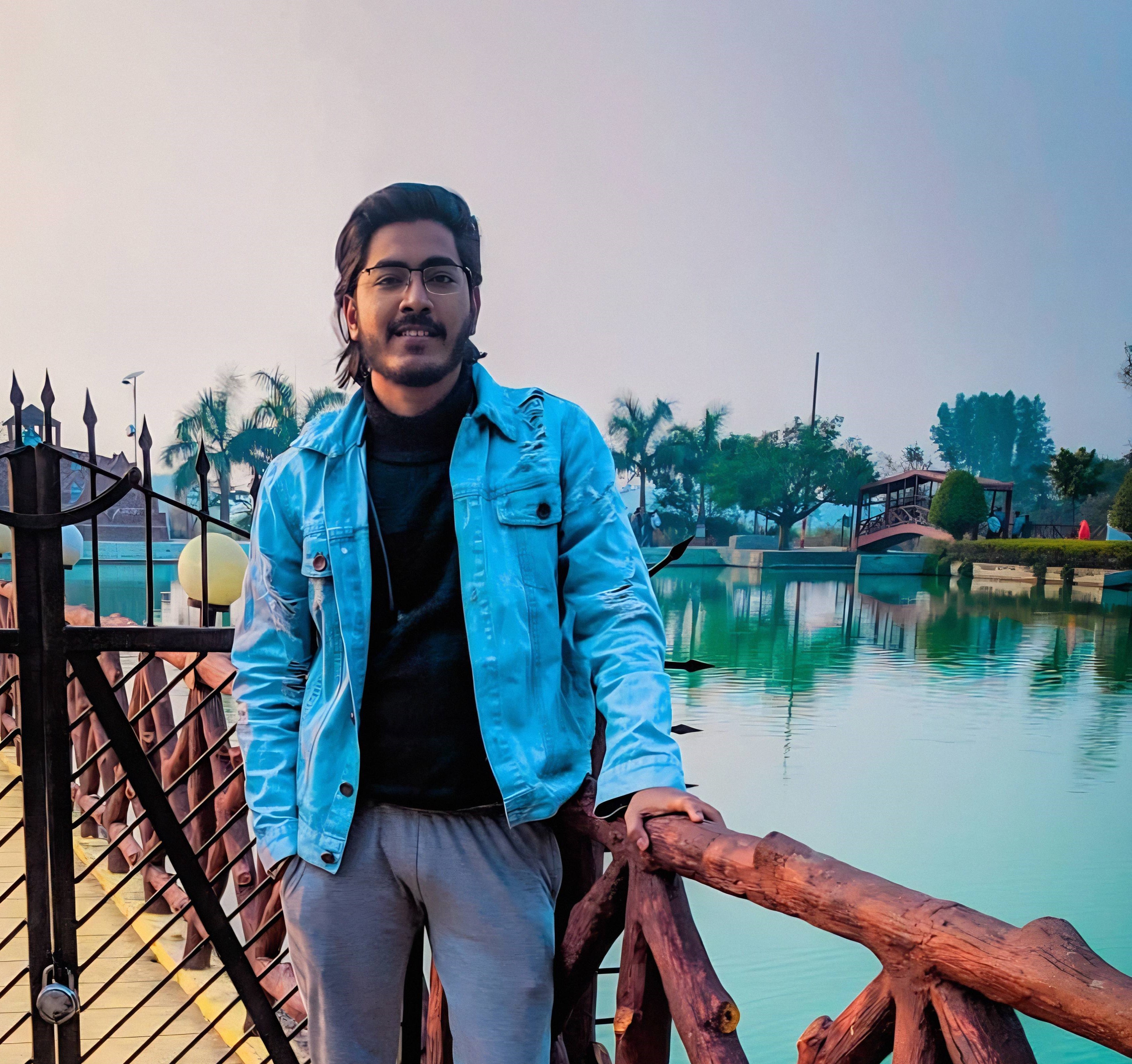
181 Views
Golomb encoding is a data compression technique used to encode non−negative integers with a specific distribution. It was introduced by Solomon W. Golomb in 1966 and has been widely used in various applications, including video and image compression, information retrieval, and data storage. In this article, we will explore Golomb encoding and understand the two cases i.e. base is a power of 2(b=2^n) and when the base is not a power of 2 (b ≠2^n). Golomb Encoding for b=2^n (Base is power of 2) When the base is a power of 2, Golomb encoding becomes relatively simpler. Let's consider ... Read More
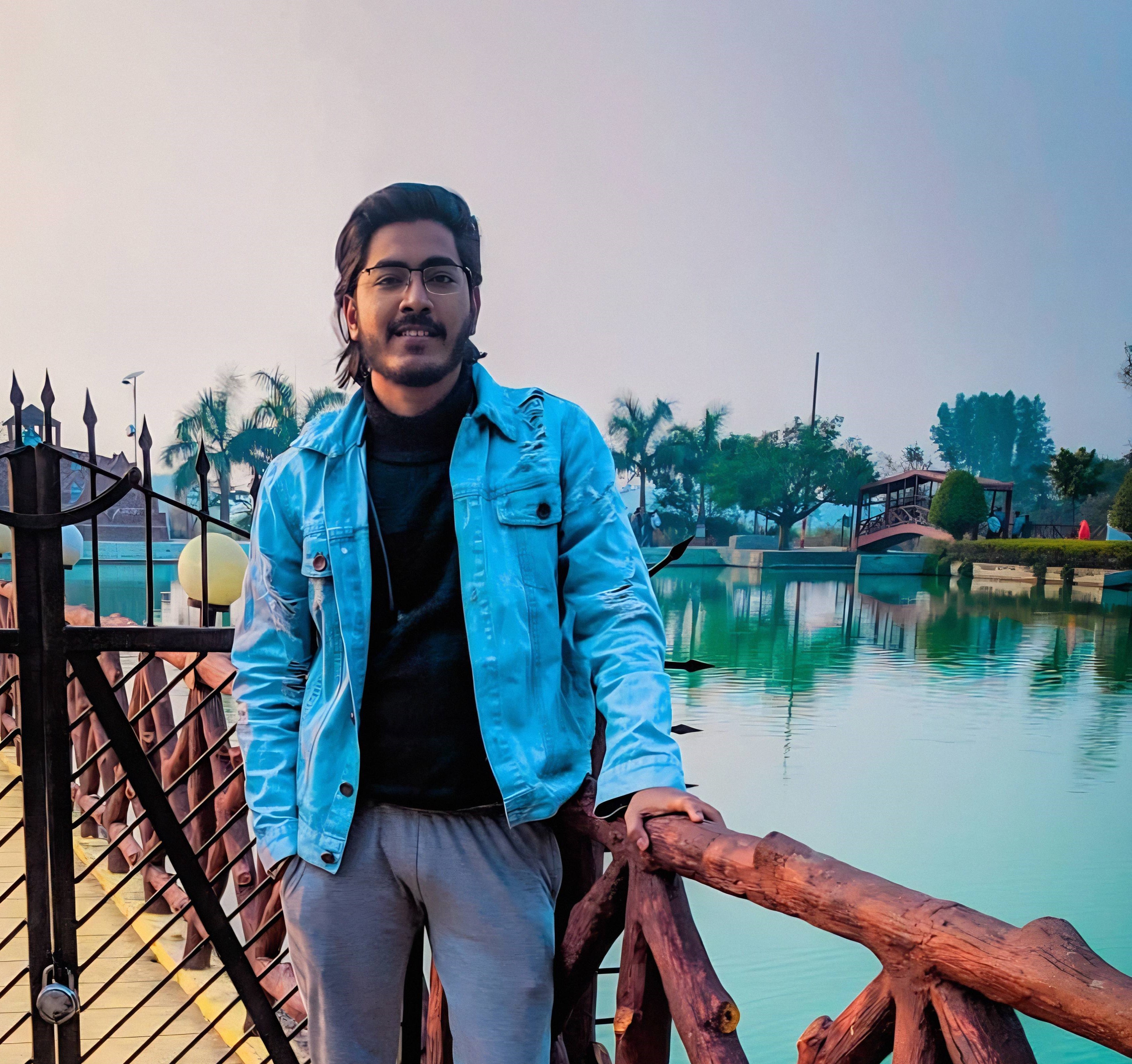
3K+ Views
In Python, we can get the object with a max attribute value in a list of objects using the max() function with a lambda function, using the operator module, using a custom comparison function, etc. In this article, we will explore different methods that can be used to get the objects with the max attribute value. Algorithm To get the object with the maximum attribute value in a list of objects, you can follow this common algorithm: Initialize a variable, let's call it max_object, to None initially. Iterate over each object in the list. For each object, compare its ... Read More
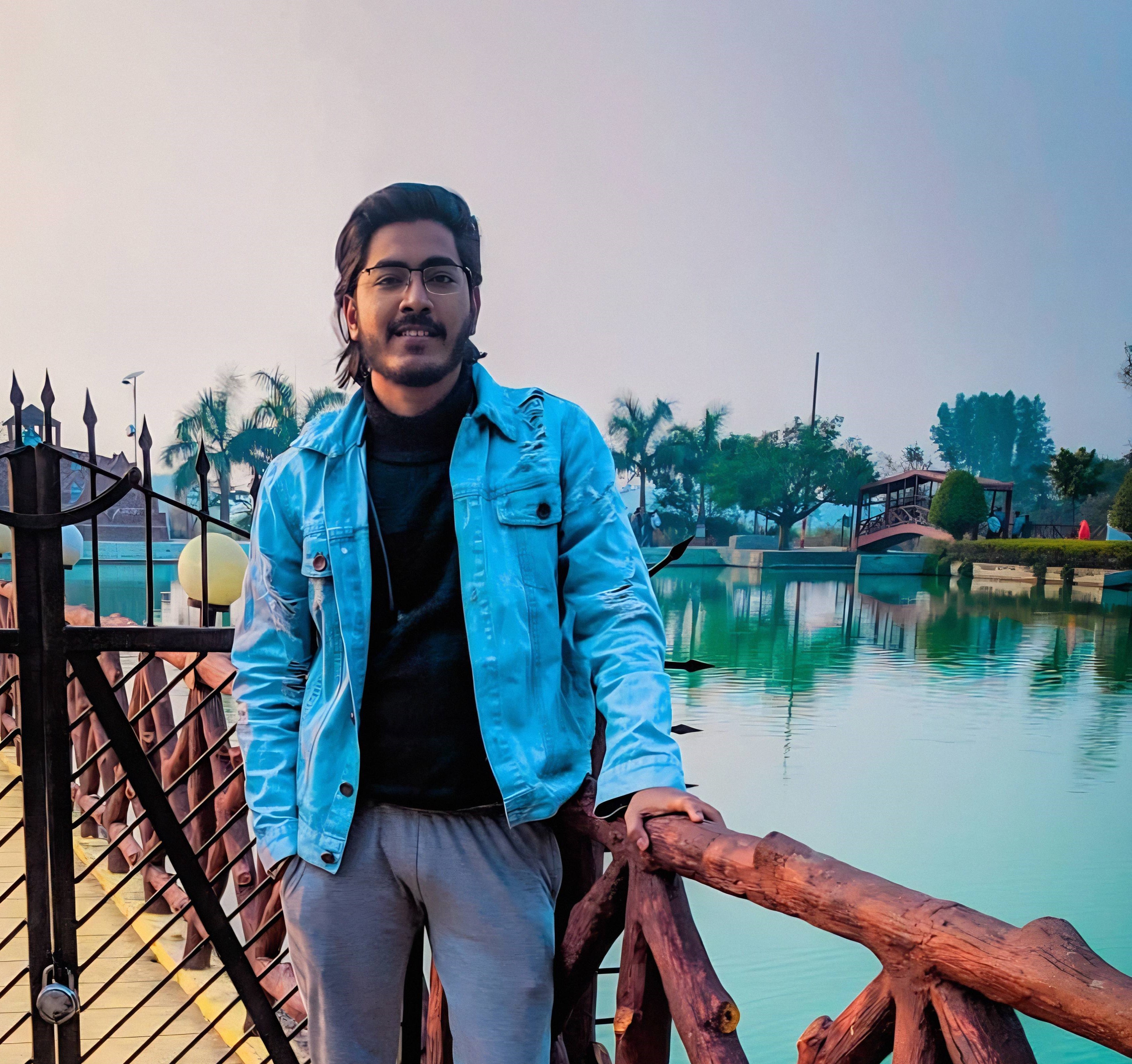
7K+ Views
To retrieve the indices of all occurrences of a specific element in a list using Python we can use methods like using for loop, using list comprehension, using enumerate() function, using the index() method in a while loop, etc. In this article, we will explore these different methods and perform the operations to get the indices of all occurrences of an element in a list. Method 1: Using for loop In this method, we start iterating through the list and comparing each element to the desired element. If the element matches we add its index to the index list. Algorithm ... Read More
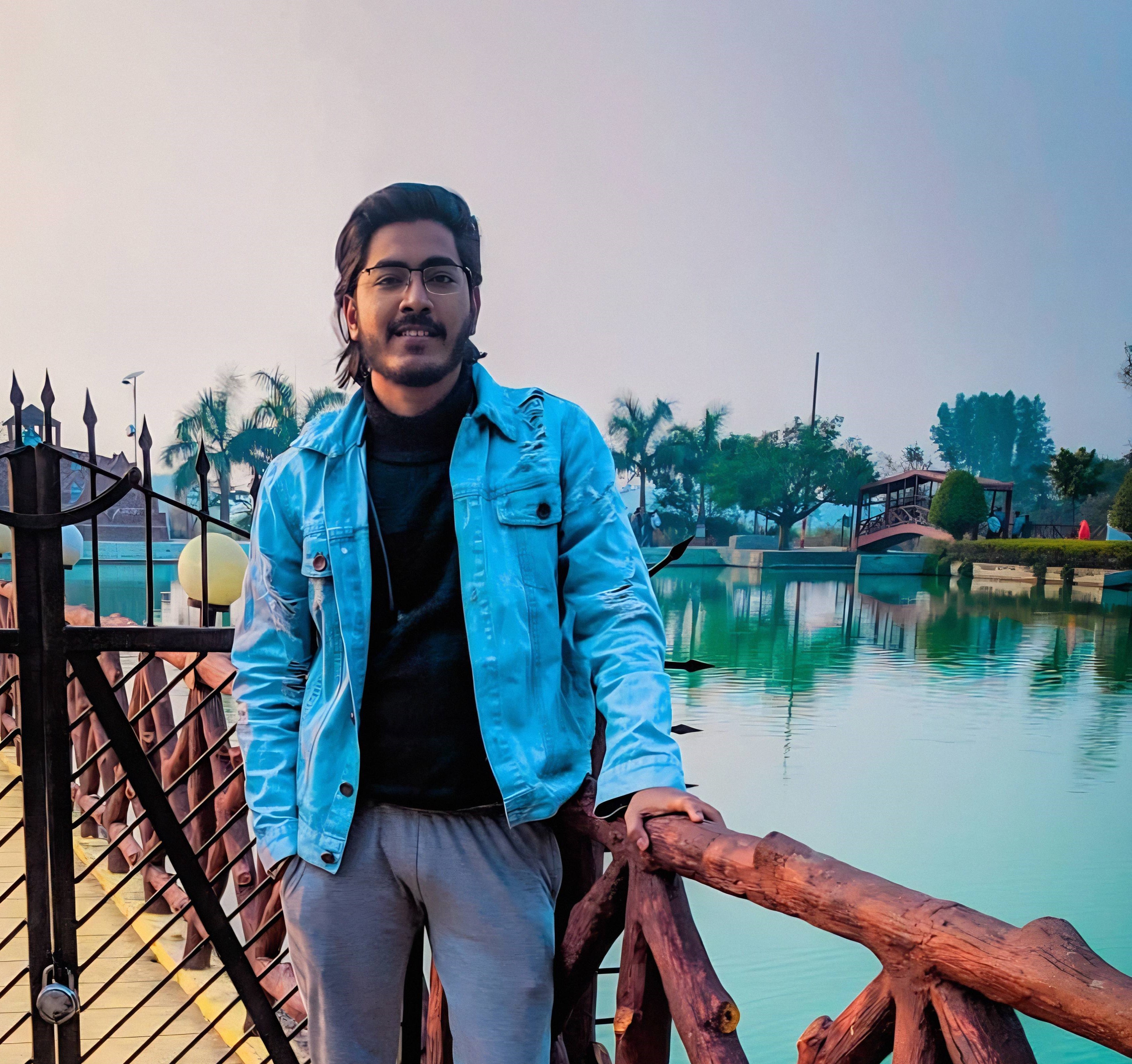
295 Views
In Python, the slice method allows us to extract specific elements from a sequence, such as strings, lists, or tuples. It provides a concise and flexible way to work with subsequences within a larger sequence. In this article, we will explore how to obtain the sum of the last K items in a list using slice operations. Algorithm To find the sum of the last K items in a list, we can follow a simple algorithm: Accept the list and the value of K as inputs. Use the slice operator to extract the last K items from the list. ... Read More