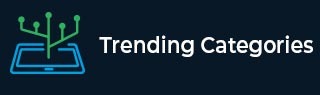
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

217 Views
Numpy is a powerful Python library that serves to store and manipulate large, multi-dimensional arrays. Although it is fast and more efficient than other similar collections like lists, we can further enhance its performance by using the parallelizing mechanism. Parallelizing means splitting the tasks into multiple processes to achieve one single goal. Python provides several ways to parallelize a numpy vector operation including multiprocessing and numexpr module. Python Programs for Parallelizing a NumPy Vector Operation Let's discuss the ways to parallelize a numpy vector: Using multiprocessing Every Python program is considered as one single process and ... Read More

841 Views
A square wave is a type of non-sinusoidal waveform that is widely used in electric and digital circuits to show signals. Basically, these circuits use a square wave to represent input and output or on and off. Python provides several ways to plot square waves including Matplotlib, NumPy and Scipy libraries. These libraries offer various built-in methods for data visualization, making it easy to create and customize square wave plots. Python Program for plotting a Square Wave Before discussing the example programs, it is necessary to familiarize ourselves with the basics of Matplotlib, NumPy and Scipy libraries. ... Read More

2K+ Views
A line graph is a common way to display the relationship between two dependent datasets. Its general purpose is to show change over time. To plot a line graph from the numpy array, we can use matplotlib which is the oldest and most widely used Python library for plotting. Also, it can be easily integrated with numpy which makes it easy to create line graphs to represent trends and patterns in the given datasets. Python Program to Plot line graph from NumPy array Here are the example programs that demonstrate how to plot line graphs from numpy ... Read More

145 Views
String manipulation is an essential skill in programming, as it helps us process and analyze text data efficiently. C++ provides a rich set of string manipulation functions and objects, making it easier to work with text data. In this article, we will discuss how to reverse a string according to the number of words in C++. Approaches Approach 1 − Using stringstreams and vectors Approach 2 − Using substrings and string manipulation functions Syntax String object in C++: The std::string class is a part of the C++ Standard Library and provides various string manipulation functions. String manipulation functions: ... Read More

4K+ Views
Numpy is a powerful Python library that serves to handle large, multi-dimensional arrays. However, when printing large numpy arrays, the interpreter often truncates the output to save space and shows only a few elements of that array. In this article, we will show how to print a full Numpy array without truncation. To understand the problem statement properly, consider the below example: Input aray = np.arange(1100) Output [ 0 1 2 ... 1097 1098 1099] In the above example, we have created an array with 1100 elements. When ... Read More

885 Views
Pandas is a powerful Python library mainly used for data analysis. Since it contains large and complicated numeric datasets that are difficult to understand, we need to plot these datasets which makes it easy to visualize relationships within the given dataset. Python provides several libraries such as Matplotlib, Plotly and Seaborn to create informative plots from the given data with ease. In this article, we will show how to plot the size of each group in a Groupby object in Pandas. Python Program to Plot the Size of each Group in a Groupby Object To plot the ... Read More

418 Views
In the realm of C++ programming, it is possible to replace every character of a specified string with a symbol whose ASCII value is elevated by a factor of K compared to the original character. This can be accomplished through the implementation of a straightforward algorithmic technique. This piece delves into the syntax, the algorithm, and two distinct methods to address the problem, complete with code illustrations in C++. Syntax To substitute every character in a string with a character whose ASCII value is multiplied by K, the syntax is as follows − string replace(string str, int K); Here, ... Read More

1K+ Views
A dynamic multi-stack is a remarkable data structure that possesses the capacity to store elements in numerous stacks, with an ever-changing quantity of stacks. It can be a daunting task to implement K stacks utilizing only one data structure. In this instructional guide, we shall investigate two distinct techniques to execute dynamic multi-stack (K stacks) using C++. The initial technique employs an array to stock the elements, along with two additional arrays to monitor the topmost and following indices of the stacks. The secondary technique employs a vector of nodes to stock the elements, along with a vector to keep ... Read More

2K+ Views
Finding the percentile rank is a common operation that is used for comparison between data of a single dataset. The end result of this operation shows a certain percentage is greater than or equal to the specified percentile. For instance, suppose a student obtains a score greater than or equal to 80% of all other scores. Then, the percentile rank of that student is 80th. To find the percentile rank of a column in a Pandas DataFrame, we can use the built-in methods named 'rank()' and 'percentile()' provided by Python. Python Program to find Percentile Rank of ... Read More

322 Views
While presenting or explaining some facts and figures using Pandas DataFrame, we might need to highlight important rows and columns of the given data that help in making them more appealing, easier to understand and visually stunning. One way of highlighting the Pandas DataFrame's specific columns is by using the built-in method applymap(). Python Program to Highlight Pandas DataFrame using applymap() To understand the code properly, we need to discuss the basics of Pandas and applymap(): Pandas It is an open-source Python library that is mainly used for data analysis and manipulation. It can handle ... Read More