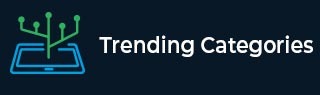
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

168 Views
In the field of computer programming, finding the count of numbers between a given range that are coprime with a specific number can be a common task. Coprime numbers, also known as relatively prime numbers, are those that have no common factors other than 1. In this piece, we shall delve into finding the coprime numbers count between two given integers, L and R when referring to a particular number P, through the aid of C++ language. Syntax We'll commence by outlining the syntax of the approach we will be utilizing in the succeeding code examples − int countCoprimes(int ... Read More

516 Views
Python is a versatile programming language that has gained immense popularity in the field of data analysis and machine learning. Its simplicity, readability, and vast array of libraries make it an ideal choice for handling complex data tasks. One such powerful application is RFM analysis, a technique used in marketing to segment customers based on their purchasing behavior. In this tutorial, we will guide you through the process of implementing RFM analysis using Python. We will start by explaining the concept of RFM analysis and its significance in marketing. Then, we will dive into the practical aspects of conducting ... Read More

452 Views
In the realm of computer science and programming, discovering effective algorithms for resolving issues is highly significant. An intriguing issue is identifying the smallest possible quantity of maneuvers necessary to convert a string into a palindrome by increasing all characters within substrings. This write-up delves into two methodologies to handle this concern utilizing the C++ programming language. Syntax Before diving into the approaches, let's define the syntax of the function we will be using − int minMovesToMakePalindrome(string str); Algorithm Our objective is to minimize move count in converting strings into palindromes—a problem which our algorithm approaches with these ... Read More

50 Views
In the field of computer science, solving optimization problems efficiently is crucial for developing optimal algorithms and systems. One such problem is minimizing the XOR (exclusive OR) of pairs in an array to make it a palindrome. This situation holds significance because it offers a chance to determine the optimal approach towards reordering items within an array, which can lead to lower XOR value and creation of palindromes. This essay examines two methods that utilize C++ programming language for resolving this predicament. Syntax To begin with, let's define the syntax of the function we will be using in the following ... Read More

1K+ Views
Python and OpenCV have revolutionized the field of computer vision, enabling developers and researchers to explore a wide range of applications. With its simplicity, versatility, and extensive library support, Python has become a popular programming language for various domains, including computer vision. Complementing Python's capabilities, OpenCV (Open Source Computer Vision Library) provides a powerful toolkit for image and video processing, making it a go−to choice for implementing computer vision algorithms. In this article, we will guide you through the process of setting up your development environment, capturing real−time video feeds, calibrating the camera, and implementing object detection and tracking ... Read More

1K+ Views
Good problem-solving in computer science heavily relies on efficient algorithms like the Greedy-Best First Search (GBFS). GBFS has already established credibility as an optimal solution method for pathfinding or optimization issues. Therefore, we're discussing GBFS thoroughly in this article while exploring its implementation approach using C++. Syntax void greedyBestFirstSearch(Graph graph, Node startNode, Node goalNode); Algorithm The Greedy Best-First Search algorithm aims to find the path from a given start node to a goal node in a graph. Here are the general steps of the algorithm − Initialize an empty priority queue. Enqueue the start node into the priority ... Read More

26 Views
Efficiency and accuracy are often essential when solving complex problems in programming. One particular challenge involves appropriately identifying whether the maximum sum of N dice visible faces equals or surpasses X. In this piece, we evaluate various methods of tackling this difficulty in C++ coding, complete with syntax explanations and step-by-step algorithms. Furthermore, we will provide two real, full executable code examples based on the approaches mentioned. By the end, you will have a clear understanding of how to check if the maximum sum of visible faces of N dice is at least X in C++. Syntax Before diving into ... Read More

394 Views
In graph theory, finding the minimum spanning tree (MST) of a connected weighted graph is a common problem. The MST is a subset of the graph's edges that connects all the vertices while minimizing the total edge weight. One efficient algorithm to solve this problem is Boruvka's algorithm. Syntax struct Edge { int src, dest, weight; }; // Define the structure to represent a subset for union-find struct Subset { int parent, rank; }; Algorithm Now, let's outline the steps involved in Boruvka's algorithm for finding the minimum spanning tree − ... Read More

251 Views
Python, known for its versatility and extensive libraries, has now ventured into the realm of quantum computing, opening doors to extraordinary phenomena like quantum teleportation. In this tutorial, we explore the captivating concept of quantum teleportation and showcase how Python can be harnessed to implement this remarkable phenomenon. Whether you're a quantum enthusiast, a Python developer, or simply curious about the marvels of quantum computing, join us on this practical journey to understand and implement quantum teleportation in Python. In this article, we will delve into the intricate world of quantum teleportation, where information is transferred instantaneously across space using ... Read More

727 Views
In computer science and graph theory, solutions to a variety of real-life model scenarios rely heavily on directed graphs. These specialized graphs consist of linked vertices anchored by directed edges pointing to other vertices. Determining if there's a pathway between two designated points is one typical quandary associated with using directed graphs. In this discourse, we explore diverse methods for resolving this dilemma using C++, including the required syntax for each procedure to keep things understandable. Further still, we'll present detailed algorithms meticulously illustrating each method and include two executable code examples. Syntax Its' essential to comprehend the language structure ... Read More