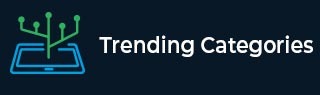
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 27104 Articles for Server Side Programming

39 Views
Programming requires regular optimization of algorithms geared toward specific outcomes. One fundamental task is identifying partitions of arrays within C++ that maximize sums made up entirely of left-side zeros and right-side ones. To achieve a solution, this article will survey different approaches alongside step-by-step instructions and two functional code demonstrations. Syntax To make sure our readers have an easy time following along with our code examples. It's critical that we establish a consistent syntax upfront. So before getting into algorithms and approaches let's determine this essential baseline. − #include #include using namespace std; // Function to find ... Read More

2K+ Views
The algorithm known as Union-Find (or Disjoint-Set) is in charge of maintaining distinct sets and offers operations to verify membership in a set and to combine sets together. It adeptly handles the union and find operations, both crucial for maintaining current connectivity information between elements. Syntax To ensure clarity let us first comprehend the syntax of the methods that are to be utilized in the upcoming code examples. // Method to perform Union operation void Union(int x, int y); // Method to find the representative element of a set int Find(int x); Algorithm The Union-Find algorithm consists of ... Read More

71 Views
A standard representation for intervals usually entails a set of starting and ending points arranged in pairs. Finding the nearest non-overlapping interval to the right of each designated interval constitutes our current dilemma. This task bears immense significance across many different applications such as resource allocation and scheduling since it involves identifying the following interval that does not intersect with or include the present one. Syntax To aid in comprehension of the forthcoming code demonstrations, let us first examine the syntax that will be utilized before delving into the algorithm − // Define the Interval structure struct Interval { ... Read More

89 Views
Dividing a given value N by distinct powers of prime integers presents an interesting problem within computer programming. It mandates analyzing the number of times that N can potentially undergo division with these varying powers. Here, we aim to explore this challenge and demonstrate its resolution via implementation in C++. Syntax Prior to analyzing the algorithm and various approaches to it. It is vital to have a comprehensive understanding of how syntax will be incorporated in forthcoming code instances. // Syntax for dividing N by distinct powers of prime integers int countDivisions(int N); Algorithm The algorithm for determining the ... Read More

282 Views
For C++ coders, reducing the gap between maximum and minimum element amounts in an array can prove useful. This promotes even dispersal of values across all its elements, potentially resulting in manifold benefits in multiple scenarios. Our present focus is implementing methods to optimize balance within array structures by means of augmenting or reducing their size via practical techniques. Syntax Before diving into the algorithms' specifics let us first briefly examine the syntax of the method used in our illustrative code examples − void minimizeDifference(int arr[], int n); The minimizeDifference function takes an array arr and its size n ... Read More

141 Views
Our purpose is to determine whether performing multiple divisions on each item contained within an array creates a list of integers from one through N devoid of any duplicates. Success in this endeavor would denote the accomplishment of our investigative objectives satisfactorily. In essence, ascertaining whether cutting all elements provided within the given array by two produces permutations comprised entirely of non-repeating values ranging between one and N constitutes the main focus of our work. When confirmed, evaluating our thesis follows as the next logical step. Syntax Before delving into our proposed solution it is important for us to have ... Read More

64 Views
In this article, we aim to delve into a captivating problem concerning the greatest common divisor (GCD) of arrays in multiple programming languages, with a focus on C++. We'll showcase an algorithmic method that leverages element exchange in pairs alongside their product quantities to verify if it is feasible to raise GCD above 1. Additionally, we'll offer alternative approaches towards solving this problem, each with its syntax definition. Alongside these solutions, we'll present two complete executable codes incorporating said approaches. Syntax To ensure a clear understanding of subsequent code examples, it is imperative we evaluate and understand the syntax utilized ... Read More

61 Views
Our objective is to successfully confront the presented issue by determining the number of indices with a value of 1 following consecutive operations. We have planned to accomplish this task through sequential and methodical execution of each operation utilizing C++ as our preferred programming language. However, for a permanent solution to be achieved, it is crucial for us to formulate an effective algorithmic blueprint coded appropriately. Syntax To better prepare ourselves for the algorithm. It is advisable to become acquainted with the coding syntax. The code snippets that follow will make use of a particular method as shown below − ... Read More

2K+ Views
In the world of data−driven applications and analysis, APIs (Application Programming Interfaces) play a crucial role in retrieving data from various sources. When working with API data, it is often necessary to store it in a format that is easily accessible and manipulatable. One such format is CSV (Comma Separated Values), which allows tabular data to be organized and stored efficiently. This article will explore the process of saving API data into CSV format using the powerful programming language Python. By following the steps outlined in this guide, we will learn how to retrieve data from an API, extract relevant ... Read More

111 Views
Data structure manipulation is now an integral aspect of successful solution development in modern programming and computation. This arises from increasing complexities presented in these structures over time. A case in point is performing swaps to minimize the sum of maximum numbers included within two arrays; thereby decreasing their overall value. In this write-up, we discuss two approaches to accomplishing such tasks with C++ as our primary programming language while acknowledging both methods' strengths and weaknesses based on varying opinions. Syntax In order to comprehend the methods and codes effectively we need a solid understanding of the fundamental grammar in ... Read More